mirror of
https://github.com/bitwarden/server.git
synced 2025-07-05 10:02:47 -05:00
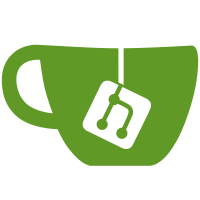
* [PM-17562] Add Azure Service Bus for Distributed Events * Fix failing test * Addressed issues mentioned in SonarQube * Respond to PR feedback * Respond to PR feedback - make webhook opt-in, remove message body from log
29 lines
851 B
C#
29 lines
851 B
C#
using System.Net.Http.Json;
|
|
using Bit.Core.Models.Data;
|
|
using Bit.Core.Settings;
|
|
|
|
namespace Bit.Core.Services;
|
|
|
|
public class WebhookEventHandler : IEventMessageHandler
|
|
{
|
|
private readonly HttpClient _httpClient;
|
|
private readonly string _webhookUrl;
|
|
|
|
public const string HttpClientName = "WebhookEventHandlerHttpClient";
|
|
|
|
public WebhookEventHandler(
|
|
IHttpClientFactory httpClientFactory,
|
|
GlobalSettings globalSettings)
|
|
{
|
|
_httpClient = httpClientFactory.CreateClient(HttpClientName);
|
|
_webhookUrl = globalSettings.EventLogging.WebhookUrl;
|
|
}
|
|
|
|
public async Task HandleEventAsync(EventMessage eventMessage)
|
|
{
|
|
var content = JsonContent.Create(eventMessage);
|
|
var response = await _httpClient.PostAsync(_webhookUrl, content);
|
|
response.EnsureSuccessStatusCode();
|
|
}
|
|
}
|