mirror of
https://github.com/bitwarden/server.git
synced 2025-07-08 03:15:07 -05:00
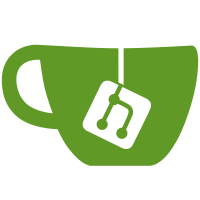
* CSA-2 - adding validation before redirecting for SSO login * Updating server to use generated and signed JWT for SSO redirect * Removing erroneous file * Removing erroneous file * Updating for PR feedback, adding domain_hint to Login and fixing invalid domain_hint name reference * Some code styling changes from PR feedback * Removing unnecessary JSON serialization * Couple small changes from PR feedback * Fixing linting errors * Update formatting in AccountController.cs * Remove unused dependency * Add token lifetime to settings * Use tokenable directly * Return defined models * Revert sso proj file changes * Check expiration validity when validating org * Show error message with expired token * Formatting fixes * Add SsoTokenLifetime to Sso settings * Fix build errors * Fix sql warnings Co-authored-by: Carlos J. Muentes <cmuentes@bitwarden.com> Co-authored-by: Chad Scharf <3904944+cscharf@users.noreply.github.com> Co-authored-by: Matt Gibson <mgibson@bitwarden.com>
91 lines
2.7 KiB
C#
91 lines
2.7 KiB
C#
using System;
|
|
using AutoFixture.Xunit2;
|
|
using Bit.Core.Entities;
|
|
using Bit.Core.Models.Business.Tokenables;
|
|
using Bit.Core.Tokens;
|
|
using Bit.Test.Common.AutoFixture.Attributes;
|
|
using Xunit;
|
|
|
|
namespace Bit.Core.Test.Models.Business.Tokenables
|
|
{
|
|
public class SsoTokenableTests
|
|
{
|
|
[Fact]
|
|
public void CanHandleNullOrganization()
|
|
{
|
|
var token = new SsoTokenable(null, default);
|
|
|
|
Assert.Equal(default, token.OrganizationId);
|
|
Assert.Equal(default, token.DomainHint);
|
|
}
|
|
|
|
[Fact]
|
|
public void TokenWithNullOrganizationIsInvalid()
|
|
{
|
|
var token = new SsoTokenable(null, 500)
|
|
{
|
|
ExpirationDate = DateTime.UtcNow + TimeSpan.FromDays(1)
|
|
};
|
|
|
|
Assert.False(token.Valid);
|
|
}
|
|
|
|
[Theory, BitAutoData]
|
|
public void TokenValidityCheckNullOrganizationIsInvalid(Organization organization)
|
|
{
|
|
var token = new SsoTokenable(organization, 500)
|
|
{
|
|
ExpirationDate = DateTime.UtcNow + TimeSpan.FromDays(1)
|
|
};
|
|
|
|
Assert.False(token.TokenIsValid(null));
|
|
}
|
|
|
|
[Theory, AutoData]
|
|
public void SetsDataFromOrganization(Organization organization)
|
|
{
|
|
var token = new SsoTokenable(organization, default);
|
|
|
|
Assert.Equal(organization.Id, token.OrganizationId);
|
|
Assert.Equal(organization.Identifier, token.DomainHint);
|
|
}
|
|
|
|
[Fact]
|
|
public void SetsExpirationFromConstructor()
|
|
{
|
|
var expectedDateTime = DateTime.UtcNow.AddSeconds(500);
|
|
var token = new SsoTokenable(null, 500);
|
|
|
|
Assert.Equal(expectedDateTime, token.ExpirationDate, TimeSpan.FromMilliseconds(10));
|
|
}
|
|
|
|
[Theory, AutoData]
|
|
public void SerializationSetsCorrectDateTime(Organization organization)
|
|
{
|
|
var expectedDateTime = DateTime.UtcNow.AddHours(-5);
|
|
var token = new SsoTokenable(organization, default)
|
|
{
|
|
ExpirationDate = expectedDateTime
|
|
};
|
|
|
|
var result = Tokenable.FromToken<HCaptchaTokenable>(token.ToToken());
|
|
|
|
Assert.Equal(expectedDateTime, result.ExpirationDate, TimeSpan.FromMilliseconds(10));
|
|
}
|
|
|
|
[Theory, AutoData]
|
|
public void TokenIsValidFailsWhenExpired(Organization organization)
|
|
{
|
|
var expectedDateTime = DateTime.UtcNow.AddHours(-5);
|
|
var token = new SsoTokenable(organization, default)
|
|
{
|
|
ExpirationDate = expectedDateTime
|
|
};
|
|
|
|
var result = token.TokenIsValid(organization);
|
|
|
|
Assert.False(result);
|
|
}
|
|
}
|
|
}
|