mirror of
https://github.com/bitwarden/server.git
synced 2025-04-05 05:00:19 -05:00
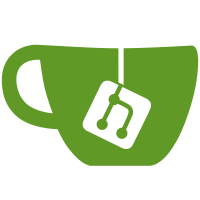
* Allow for binning of comb IDs by date and value * Introduce notification hub pool * Replace device type sharding with comb + range sharding * Fix proxy interface * Use enumerable services for multiServiceNotificationHub * Fix push interface usage * Fix push notification service dependencies * Fix push notification keys * Fixup documentation * Remove deprecated settings * Fix tests * PascalCase method names * Remove unused request model properties * Remove unused setting * Improve DateFromComb precision * Prefer readonly service enumerable * Pascal case template holes * Name TryParse methods TryParse * Apply suggestions from code review Co-authored-by: Justin Baur <19896123+justindbaur@users.noreply.github.com> * Include preferred push technology in config response SignalR will be the fallback, but clients should attempt web push first if offered and available to the client. * Register web push devices * Working signing and content encrypting * update to RFC-8291 and RFC-8188 * Notification hub is now working, no need to create our own * Fix body * Flip Success Check * use nifty json attribute * Remove vapid private key This is only needed to encrypt data for transmission along webpush -- it's handled by NotificationHub for us * Add web push feature flag to control config response * Update src/Core/NotificationHub/NotificationHubConnection.cs Co-authored-by: Justin Baur <19896123+justindbaur@users.noreply.github.com> * Update src/Core/NotificationHub/NotificationHubConnection.cs Co-authored-by: Justin Baur <19896123+justindbaur@users.noreply.github.com> * fixup! Update src/Core/NotificationHub/NotificationHubConnection.cs * Move to platform ownership * Remove debugging extension * Remove unused dependencies * Set json content directly * Name web push registration data * Fix FCM type typo * Determine specific feature flag from set of flags * Fixup merged tests * Fixup tests * Code quality suggestions * Fix merged tests * Fix test --------- Co-authored-by: Justin Baur <19896123+justindbaur@users.noreply.github.com>
99 lines
2.3 KiB
C#
99 lines
2.3 KiB
C#
using System.ComponentModel.DataAnnotations;
|
|
using Bit.Core.Entities;
|
|
using Bit.Core.Enums;
|
|
using Bit.Core.NotificationHub;
|
|
using Bit.Core.Utilities;
|
|
|
|
namespace Bit.Api.Models.Request;
|
|
|
|
public class DeviceRequestModel
|
|
{
|
|
[Required]
|
|
public DeviceType? Type { get; set; }
|
|
[Required]
|
|
[StringLength(50)]
|
|
public string Name { get; set; }
|
|
[Required]
|
|
[StringLength(50)]
|
|
public string Identifier { get; set; }
|
|
[StringLength(255)]
|
|
public string PushToken { get; set; }
|
|
|
|
public Device ToDevice(Guid? userId = null)
|
|
{
|
|
return ToDevice(new Device
|
|
{
|
|
UserId = userId == null ? default(Guid) : userId.Value
|
|
});
|
|
}
|
|
|
|
public Device ToDevice(Device existingDevice)
|
|
{
|
|
existingDevice.Name = Name;
|
|
existingDevice.Identifier = Identifier;
|
|
existingDevice.PushToken = PushToken;
|
|
existingDevice.Type = Type.Value;
|
|
|
|
return existingDevice;
|
|
}
|
|
}
|
|
|
|
public class WebPushAuthRequestModel
|
|
{
|
|
[Required]
|
|
public string Endpoint { get; set; }
|
|
[Required]
|
|
public string P256dh { get; set; }
|
|
[Required]
|
|
public string Auth { get; set; }
|
|
|
|
public WebPushRegistrationData ToData()
|
|
{
|
|
return new WebPushRegistrationData
|
|
{
|
|
Endpoint = Endpoint,
|
|
P256dh = P256dh,
|
|
Auth = Auth
|
|
};
|
|
}
|
|
}
|
|
|
|
public class DeviceTokenRequestModel
|
|
{
|
|
[StringLength(255)]
|
|
public string PushToken { get; set; }
|
|
|
|
public Device ToDevice(Device existingDevice)
|
|
{
|
|
existingDevice.PushToken = PushToken;
|
|
return existingDevice;
|
|
}
|
|
}
|
|
|
|
public class DeviceKeysRequestModel
|
|
{
|
|
/// <inheritdoc cref="Device.EncryptedUserKey" />
|
|
[Required]
|
|
[EncryptedString]
|
|
public string EncryptedUserKey { get; set; }
|
|
|
|
/// <inheritdoc cref="Device.EncryptedPublicKey" />
|
|
[Required]
|
|
[EncryptedString]
|
|
public string EncryptedPublicKey { get; set; }
|
|
|
|
/// <inheritdoc cref="Device.EncryptedPrivateKey" />
|
|
[Required]
|
|
[EncryptedString]
|
|
public string EncryptedPrivateKey { get; set; }
|
|
|
|
public Device ToDevice(Device existingDevice)
|
|
{
|
|
existingDevice.EncryptedUserKey = EncryptedUserKey;
|
|
existingDevice.EncryptedPublicKey = EncryptedPublicKey;
|
|
existingDevice.EncryptedPrivateKey = EncryptedPrivateKey;
|
|
|
|
return existingDevice;
|
|
}
|
|
}
|