mirror of
https://github.com/bitwarden/server.git
synced 2025-07-14 22:27:32 -05:00
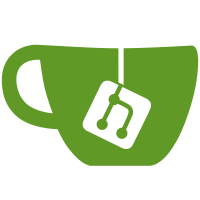
* Increase individual import limits * Increase organizational import limits --------- Co-authored-by: Daniel James Smith <djsmith85@users.noreply.github.com>
78 lines
2.9 KiB
C#
78 lines
2.9 KiB
C#
using Bit.Api.Tools.Models.Request.Accounts;
|
|
using Bit.Api.Tools.Models.Request.Organizations;
|
|
using Bit.Core.Context;
|
|
using Bit.Core.Exceptions;
|
|
using Bit.Core.Repositories;
|
|
using Bit.Core.Services;
|
|
using Bit.Core.Settings;
|
|
using Bit.Core.Vault.Services;
|
|
using Microsoft.AspNetCore.Authorization;
|
|
using Microsoft.AspNetCore.Mvc;
|
|
|
|
namespace Bit.Api.Tools.Controllers;
|
|
|
|
[Route("ciphers")]
|
|
[Authorize("Application")]
|
|
public class ImportCiphersController : Controller
|
|
{
|
|
private readonly ICipherService _cipherService;
|
|
private readonly IUserService _userService;
|
|
private readonly ICurrentContext _currentContext;
|
|
private readonly ILogger<ImportCiphersController> _logger;
|
|
private readonly GlobalSettings _globalSettings;
|
|
|
|
public ImportCiphersController(
|
|
ICollectionCipherRepository collectionCipherRepository,
|
|
ICipherService cipherService,
|
|
IUserService userService,
|
|
ICurrentContext currentContext,
|
|
ILogger<ImportCiphersController> logger,
|
|
GlobalSettings globalSettings)
|
|
{
|
|
_cipherService = cipherService;
|
|
_userService = userService;
|
|
_currentContext = currentContext;
|
|
_logger = logger;
|
|
_globalSettings = globalSettings;
|
|
}
|
|
|
|
[HttpPost("import")]
|
|
public async Task PostImport([FromBody] ImportCiphersRequestModel model)
|
|
{
|
|
if (!_globalSettings.SelfHosted &&
|
|
(model.Ciphers.Count() > 7000 || model.FolderRelationships.Count() > 7000 ||
|
|
model.Folders.Count() > 2000))
|
|
{
|
|
throw new BadRequestException("You cannot import this much data at once.");
|
|
}
|
|
|
|
var userId = _userService.GetProperUserId(User).Value;
|
|
var folders = model.Folders.Select(f => f.ToFolder(userId)).ToList();
|
|
var ciphers = model.Ciphers.Select(c => c.ToCipherDetails(userId, false)).ToList();
|
|
await _cipherService.ImportCiphersAsync(folders, ciphers, model.FolderRelationships);
|
|
}
|
|
|
|
[HttpPost("import-organization")]
|
|
public async Task PostImport([FromQuery] string organizationId,
|
|
[FromBody] ImportOrganizationCiphersRequestModel model)
|
|
{
|
|
if (!_globalSettings.SelfHosted &&
|
|
(model.Ciphers.Count() > 7000 || model.CollectionRelationships.Count() > 14000 ||
|
|
model.Collections.Count() > 2000))
|
|
{
|
|
throw new BadRequestException("You cannot import this much data at once.");
|
|
}
|
|
|
|
var orgId = new Guid(organizationId);
|
|
if (!await _currentContext.AccessImportExport(orgId))
|
|
{
|
|
throw new NotFoundException();
|
|
}
|
|
|
|
var userId = _userService.GetProperUserId(User).Value;
|
|
var collections = model.Collections.Select(c => c.ToCollection(orgId)).ToList();
|
|
var ciphers = model.Ciphers.Select(l => l.ToOrganizationCipherDetails(orgId)).ToList();
|
|
await _cipherService.ImportCiphersAsync(collections, ciphers, model.CollectionRelationships, userId);
|
|
}
|
|
}
|