mirror of
https://github.com/bitwarden/server.git
synced 2025-07-03 00:52:49 -05:00
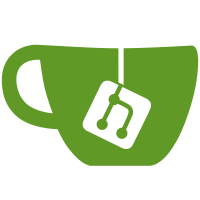
* Remove gRPC and convert PricingClient to HttpClient wrapper * Add PlanType.GetProductTier extension Many instances of StaticStore use are just to get the ProductTierType of a PlanType, but this can be derived from the PlanType itself without having to fetch the entire plan. * Remove invocations of the StaticStore in non-Test code * Deprecate StaticStore entry points * Run dotnet format * Matt's feedback * Run dotnet format * Rui's feedback * Run dotnet format * Replacements since approval * Run dotnet format
63 lines
1.8 KiB
C#
63 lines
1.8 KiB
C#
using Bit.Core.Billing;
|
|
using Bit.Core.Billing.Enums;
|
|
using Bit.Core.Billing.Extensions;
|
|
using Stripe;
|
|
using Plan = Bit.Core.Models.StaticStore.Plan;
|
|
|
|
namespace Bit.Core.Models.Business;
|
|
|
|
public class ProviderSubscriptionUpdate : SubscriptionUpdate
|
|
{
|
|
private readonly string _planId;
|
|
private readonly int _previouslyPurchasedSeats;
|
|
private readonly int _newlyPurchasedSeats;
|
|
|
|
protected override List<string> PlanIds => [_planId];
|
|
|
|
public ProviderSubscriptionUpdate(
|
|
Plan plan,
|
|
int previouslyPurchasedSeats,
|
|
int newlyPurchasedSeats)
|
|
{
|
|
if (!plan.Type.SupportsConsolidatedBilling())
|
|
{
|
|
throw new BillingException(
|
|
message: $"Cannot create a {nameof(ProviderSubscriptionUpdate)} for {nameof(PlanType)} that doesn't support consolidated billing");
|
|
}
|
|
|
|
_planId = plan.PasswordManager.StripeProviderPortalSeatPlanId;
|
|
_previouslyPurchasedSeats = previouslyPurchasedSeats;
|
|
_newlyPurchasedSeats = newlyPurchasedSeats;
|
|
}
|
|
|
|
public override List<SubscriptionItemOptions> RevertItemsOptions(Subscription subscription)
|
|
{
|
|
var subscriptionItem = FindSubscriptionItem(subscription, _planId);
|
|
|
|
return
|
|
[
|
|
new SubscriptionItemOptions
|
|
{
|
|
Id = subscriptionItem.Id,
|
|
Price = _planId,
|
|
Quantity = _previouslyPurchasedSeats
|
|
}
|
|
];
|
|
}
|
|
|
|
public override List<SubscriptionItemOptions> UpgradeItemsOptions(Subscription subscription)
|
|
{
|
|
var subscriptionItem = FindSubscriptionItem(subscription, _planId);
|
|
|
|
return
|
|
[
|
|
new SubscriptionItemOptions
|
|
{
|
|
Id = subscriptionItem.Id,
|
|
Price = _planId,
|
|
Quantity = _newlyPurchasedSeats
|
|
}
|
|
];
|
|
}
|
|
}
|