mirror of
https://github.com/bitwarden/server.git
synced 2025-07-02 00:22:50 -05:00
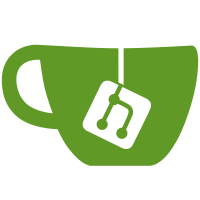
* Enable Nullable In Unowned Repos * Update More Tests * Move to One If * Fix Collections * Format * Add Migrations * Move Pragma Annotation * Add Better Assert Message
74 lines
2.6 KiB
C#
74 lines
2.6 KiB
C#
using System.Data;
|
|
using Bit.Core.Entities;
|
|
using Bit.Core.Enums;
|
|
using Bit.Core.Repositories;
|
|
using Bit.Core.Settings;
|
|
using Dapper;
|
|
using Microsoft.Data.SqlClient;
|
|
|
|
#nullable enable
|
|
|
|
namespace Bit.Infrastructure.Dapper.Repositories;
|
|
|
|
public class TransactionRepository : Repository<Transaction, Guid>, ITransactionRepository
|
|
{
|
|
public TransactionRepository(GlobalSettings globalSettings)
|
|
: this(globalSettings.SqlServer.ConnectionString, globalSettings.SqlServer.ReadOnlyConnectionString)
|
|
{ }
|
|
|
|
public TransactionRepository(string connectionString, string readOnlyConnectionString)
|
|
: base(connectionString, readOnlyConnectionString)
|
|
{ }
|
|
|
|
public async Task<ICollection<Transaction>> GetManyByUserIdAsync(Guid userId, int? limit = null)
|
|
{
|
|
using (var connection = new SqlConnection(ConnectionString))
|
|
{
|
|
var results = await connection.QueryAsync<Transaction>(
|
|
$"[{Schema}].[Transaction_ReadByUserId]",
|
|
new { UserId = userId, Limit = limit ?? int.MaxValue },
|
|
commandType: CommandType.StoredProcedure);
|
|
|
|
return results.ToList();
|
|
}
|
|
}
|
|
|
|
public async Task<ICollection<Transaction>> GetManyByOrganizationIdAsync(Guid organizationId, int? limit = null)
|
|
{
|
|
await using var connection = new SqlConnection(ConnectionString);
|
|
|
|
var results = await connection.QueryAsync<Transaction>(
|
|
$"[{Schema}].[Transaction_ReadByOrganizationId]",
|
|
new { OrganizationId = organizationId, Limit = limit ?? int.MaxValue },
|
|
commandType: CommandType.StoredProcedure);
|
|
|
|
return results.ToList();
|
|
}
|
|
|
|
public async Task<ICollection<Transaction>> GetManyByProviderIdAsync(Guid providerId, int? limit = null)
|
|
{
|
|
await using var sqlConnection = new SqlConnection(ConnectionString);
|
|
|
|
var results = await sqlConnection.QueryAsync<Transaction>(
|
|
$"[{Schema}].[Transaction_ReadByProviderId]",
|
|
new { ProviderId = providerId, Limit = limit ?? int.MaxValue },
|
|
commandType: CommandType.StoredProcedure);
|
|
|
|
return results.ToList();
|
|
}
|
|
|
|
public async Task<Transaction?> GetByGatewayIdAsync(GatewayType gatewayType, string gatewayId)
|
|
{
|
|
// maybe come back to this
|
|
using (var connection = new SqlConnection(ConnectionString))
|
|
{
|
|
var results = await connection.QueryAsync<Transaction>(
|
|
$"[{Schema}].[Transaction_ReadByGatewayId]",
|
|
new { Gateway = gatewayType, GatewayId = gatewayId },
|
|
commandType: CommandType.StoredProcedure);
|
|
|
|
return results.SingleOrDefault();
|
|
}
|
|
}
|
|
}
|