mirror of
https://github.com/bitwarden/server.git
synced 2025-05-05 11:42:22 -05:00
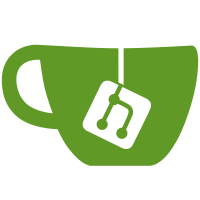
* Require provider payment method during setup behind FF * Fix failing test * Run dotnet format * Rui's feedback
40 lines
1.3 KiB
C#
40 lines
1.3 KiB
C#
using System.ComponentModel.DataAnnotations;
|
|
using System.Text.Json.Serialization;
|
|
using Bit.Api.Billing.Models.Requests;
|
|
using Bit.Api.Models.Request;
|
|
using Bit.Core.AdminConsole.Entities.Provider;
|
|
using Bit.Core.Utilities;
|
|
|
|
namespace Bit.Api.AdminConsole.Models.Request.Providers;
|
|
|
|
public class ProviderSetupRequestModel
|
|
{
|
|
[Required]
|
|
[StringLength(50, ErrorMessage = "The field Name exceeds the maximum length.")]
|
|
[JsonConverter(typeof(HtmlEncodingStringConverter))]
|
|
public string Name { get; set; }
|
|
[StringLength(50, ErrorMessage = "The field Business Name exceeds the maximum length.")]
|
|
[JsonConverter(typeof(HtmlEncodingStringConverter))]
|
|
public string BusinessName { get; set; }
|
|
[Required]
|
|
[StringLength(256)]
|
|
[EmailAddress]
|
|
public string BillingEmail { get; set; }
|
|
[Required]
|
|
public string Token { get; set; }
|
|
[Required]
|
|
public string Key { get; set; }
|
|
[Required]
|
|
public ExpandedTaxInfoUpdateRequestModel TaxInfo { get; set; }
|
|
public TokenizedPaymentSourceRequestBody PaymentSource { get; set; }
|
|
|
|
public virtual Provider ToProvider(Provider provider)
|
|
{
|
|
provider.Name = Name;
|
|
provider.BusinessName = BusinessName;
|
|
provider.BillingEmail = BillingEmail;
|
|
|
|
return provider;
|
|
}
|
|
}
|