mirror of
https://github.com/bitwarden/server.git
synced 2025-04-09 15:18:13 -05:00
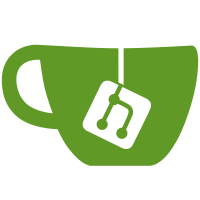
* feat: add new command for updating request and emailing user, refs AC-1191 * feat: inject service with organization service collection extensions, refs AC-1191 * feat: add function to send admin approval email to mail services (interface/noop/handlebars), refs AC-1191 * feat: add html/text mail templates and add view model for email data, refs AC-1191 * feat: update org auth request controller to use new command during auth request update, refs AC-1191 * fix: dotnet format, refs AC-1191 * refactor: update user not found error, FirstOrDefault for enum type display name, refs AC-1191 * refactor: update user not found to log error instead of throws, refs AC-1191 * fix: remove whitespace lint errors, refs AC-1191 * refactor: update hardcoded UTC timezone string, refs AC-1191 * refactor: add unit test for new command, refs AC-1191 * refactor: improve enum name fallback and identifier string creation, refs AC-1191 * refactor: add addtional unit tests, refs AC-1191 * refactor: update success test to use more generated params, refs AC-1191 * fix: dotnet format...again, refs AC-1191 * refactor: make UTC display a constant for handlebars mail service, refs AC-1191 * refactor: update displayTypeIdentifer to displayTypeAndIdentifier for clarity, refs AC-1191
88 lines
3.3 KiB
C#
88 lines
3.3 KiB
C#
using Bit.Api.Auth.Models.Request;
|
|
using Bit.Api.Auth.Models.Response;
|
|
using Bit.Api.Models.Response;
|
|
using Bit.Core;
|
|
using Bit.Core.AdminConsole.OrganizationAuth.Interfaces;
|
|
using Bit.Core.Auth.Models.Api.Request.AuthRequest;
|
|
using Bit.Core.Auth.Services;
|
|
using Bit.Core.Context;
|
|
using Bit.Core.Exceptions;
|
|
using Bit.Core.Repositories;
|
|
using Bit.Core.Utilities;
|
|
using Microsoft.AspNetCore.Authorization;
|
|
using Microsoft.AspNetCore.Mvc;
|
|
|
|
namespace Bit.Api.Controllers;
|
|
|
|
[Route("organizations/{orgId}/auth-requests")]
|
|
[Authorize("Application")]
|
|
[RequireFeature(FeatureFlagKeys.TrustedDeviceEncryption)]
|
|
public class OrganizationAuthRequestsController : Controller
|
|
{
|
|
private readonly IAuthRequestRepository _authRequestRepository;
|
|
private readonly ICurrentContext _currentContext;
|
|
private readonly IAuthRequestService _authRequestService;
|
|
private readonly IUpdateOrganizationAuthRequestCommand _updateOrganizationAuthRequestCommand;
|
|
|
|
public OrganizationAuthRequestsController(IAuthRequestRepository authRequestRepository,
|
|
ICurrentContext currentContext, IAuthRequestService authRequestService,
|
|
IUpdateOrganizationAuthRequestCommand updateOrganizationAuthRequestCommand)
|
|
{
|
|
_authRequestRepository = authRequestRepository;
|
|
_currentContext = currentContext;
|
|
_authRequestService = authRequestService;
|
|
_updateOrganizationAuthRequestCommand = updateOrganizationAuthRequestCommand;
|
|
}
|
|
|
|
[HttpGet("")]
|
|
public async Task<ListResponseModel<PendingOrganizationAuthRequestResponseModel>> GetPendingRequests(Guid orgId)
|
|
{
|
|
await ValidateAdminRequest(orgId);
|
|
|
|
var authRequests = await _authRequestRepository.GetManyPendingByOrganizationIdAsync(orgId);
|
|
var responses = authRequests
|
|
.Select(a => new PendingOrganizationAuthRequestResponseModel(a))
|
|
.ToList();
|
|
return new ListResponseModel<PendingOrganizationAuthRequestResponseModel>(responses);
|
|
}
|
|
|
|
[HttpPost("{requestId}")]
|
|
public async Task UpdateAuthRequest(Guid orgId, Guid requestId, [FromBody] AdminAuthRequestUpdateRequestModel model)
|
|
{
|
|
await ValidateAdminRequest(orgId);
|
|
|
|
var authRequest =
|
|
(await _authRequestRepository.GetManyAdminApprovalRequestsByManyIdsAsync(orgId, new[] { requestId })).FirstOrDefault();
|
|
|
|
if (authRequest == null || authRequest.OrganizationId != orgId)
|
|
{
|
|
throw new NotFoundException();
|
|
}
|
|
|
|
await _updateOrganizationAuthRequestCommand.UpdateAsync(authRequest.Id, authRequest.UserId, model.RequestApproved, model.EncryptedUserKey);
|
|
}
|
|
|
|
[HttpPost("deny")]
|
|
public async Task BulkDenyRequests(Guid orgId, [FromBody] BulkDenyAdminAuthRequestRequestModel model)
|
|
{
|
|
await ValidateAdminRequest(orgId);
|
|
|
|
var authRequests = await _authRequestRepository.GetManyAdminApprovalRequestsByManyIdsAsync(orgId, model.Ids);
|
|
|
|
foreach (var authRequest in authRequests)
|
|
{
|
|
await _authRequestService.UpdateAuthRequestAsync(authRequest.Id, authRequest.UserId,
|
|
new AuthRequestUpdateRequestModel { RequestApproved = false, });
|
|
}
|
|
}
|
|
|
|
private async Task ValidateAdminRequest(Guid orgId)
|
|
{
|
|
if (!await _currentContext.ManageResetPassword(orgId))
|
|
{
|
|
throw new UnauthorizedAccessException();
|
|
}
|
|
}
|
|
}
|
|
|