mirror of
https://github.com/bitwarden/server.git
synced 2025-04-23 14:05:10 -05:00
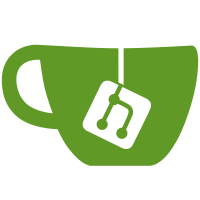
* Enable `nullable` For Collection * Enable `nullable` For `CollectionCipher` * Enable `nullable` For `CollectionGroup` * Enable `nullable` For `CollectionUser` * Enable `nullable` For `Device` * Enable `nullable` For `Event` * Enable `nullable` For `Folder` * Enable `nullable` For `Installation` * Enable `nullable` For `IRevisable` * Enable `nullable` For `IStorable` * Enable `nullable` For `IStorableSubscriber` * Enable `nullable` For `ITableObject` * Enable `nullable` For `OrganizationApiKey` * Enable `nullable` For `OrganizationConnection` * Enable `nullable` For `OrganizationDomain` * Enable `nullable` For `OrganizationSponsorship` * Enable `nullable` For `Role` * Enable `nullable` For `TaxRate` * Enable `nullable` For `Transaction` * Enable `nullable` For `User`
80 lines
1.9 KiB
C#
80 lines
1.9 KiB
C#
using System.Diagnostics.CodeAnalysis;
|
|
using System.Text.Json;
|
|
using Bit.Core.Enums;
|
|
using Bit.Core.Models.OrganizationConnectionConfigs;
|
|
using Bit.Core.Utilities;
|
|
|
|
#nullable enable
|
|
|
|
namespace Bit.Core.Entities;
|
|
|
|
public class OrganizationConnection<T> : OrganizationConnection where T : IConnectionConfig
|
|
{
|
|
[DisallowNull]
|
|
public new T? Config
|
|
{
|
|
get => base.GetConfig<T>();
|
|
set => base.SetConfig(value);
|
|
}
|
|
}
|
|
|
|
public class OrganizationConnection : ITableObject<Guid>
|
|
{
|
|
public Guid Id { get; set; }
|
|
public OrganizationConnectionType Type { get; set; }
|
|
public Guid OrganizationId { get; set; }
|
|
public bool Enabled { get; set; }
|
|
public string? Config { get; set; }
|
|
|
|
public void SetNewId()
|
|
{
|
|
Id = CoreHelpers.GenerateComb();
|
|
}
|
|
|
|
public T? GetConfig<T>() where T : IConnectionConfig
|
|
{
|
|
try
|
|
{
|
|
if (Config is null)
|
|
{
|
|
return default;
|
|
}
|
|
|
|
return JsonSerializer.Deserialize<T>(Config);
|
|
}
|
|
catch (JsonException)
|
|
{
|
|
return default;
|
|
}
|
|
}
|
|
|
|
public void SetConfig<T>(T config) where T : IConnectionConfig
|
|
{
|
|
Config = JsonSerializer.Serialize(config);
|
|
}
|
|
|
|
public bool Validate<T>(out string exception) where T : IConnectionConfig
|
|
{
|
|
if (!Enabled)
|
|
{
|
|
exception = $"Connection disabled for organization {OrganizationId}";
|
|
return false;
|
|
}
|
|
|
|
if (string.IsNullOrWhiteSpace(Config))
|
|
{
|
|
exception = $"No saved Connection config for organization {OrganizationId}";
|
|
return false;
|
|
}
|
|
|
|
var config = GetConfig<T>();
|
|
if (config == null)
|
|
{
|
|
exception = $"Error parsing Connection config for organization {OrganizationId}";
|
|
return false;
|
|
}
|
|
|
|
return config.Validate(out exception);
|
|
}
|
|
}
|