mirror of
https://github.com/bitwarden/server.git
synced 2025-07-09 11:54:41 -05:00
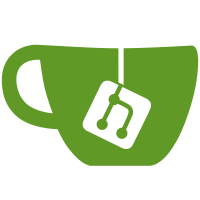
* Enable `nullable` For Collection * Enable `nullable` For `CollectionCipher` * Enable `nullable` For `CollectionGroup` * Enable `nullable` For `CollectionUser` * Enable `nullable` For `Device` * Enable `nullable` For `Event` * Enable `nullable` For `Folder` * Enable `nullable` For `Installation` * Enable `nullable` For `IRevisable` * Enable `nullable` For `IStorable` * Enable `nullable` For `IStorableSubscriber` * Enable `nullable` For `ITableObject` * Enable `nullable` For `OrganizationApiKey` * Enable `nullable` For `OrganizationConnection` * Enable `nullable` For `OrganizationDomain` * Enable `nullable` For `OrganizationSponsorship` * Enable `nullable` For `Role` * Enable `nullable` For `TaxRate` * Enable `nullable` For `Transaction` * Enable `nullable` For `User`
51 lines
1.3 KiB
C#
51 lines
1.3 KiB
C#
using System.ComponentModel.DataAnnotations;
|
|
using Bit.Core.Utilities;
|
|
|
|
#nullable enable
|
|
|
|
namespace Bit.Core.Entities;
|
|
|
|
public class OrganizationDomain : ITableObject<Guid>
|
|
{
|
|
public Guid Id { get; set; }
|
|
public Guid OrganizationId { get; set; }
|
|
public string Txt { get; set; } = null!;
|
|
[MaxLength(255)]
|
|
public string DomainName { get; set; } = null!;
|
|
public DateTime CreationDate { get; set; } = DateTime.UtcNow;
|
|
public DateTime? VerifiedDate { get; private set; }
|
|
public DateTime NextRunDate { get; private set; }
|
|
public DateTime? LastCheckedDate { get; private set; }
|
|
public int JobRunCount { get; private set; }
|
|
public void SetNewId() => Id = CoreHelpers.GenerateComb();
|
|
|
|
public void SetNextRunDate(int interval)
|
|
{
|
|
//verification can take up to 72 hours
|
|
//1st job runs after 12hrs, 2nd after 24hrs and 3rd after 36hrs
|
|
NextRunDate = JobRunCount == 0
|
|
? CreationDate.AddHours(interval)
|
|
: NextRunDate.AddHours((JobRunCount + 1) * interval);
|
|
}
|
|
|
|
public void SetJobRunCount()
|
|
{
|
|
if (JobRunCount == 3)
|
|
{
|
|
return;
|
|
}
|
|
|
|
JobRunCount++;
|
|
}
|
|
|
|
public void SetVerifiedDate()
|
|
{
|
|
VerifiedDate = DateTime.UtcNow;
|
|
}
|
|
|
|
public void SetLastCheckedDate()
|
|
{
|
|
LastCheckedDate = DateTime.UtcNow;
|
|
}
|
|
}
|