mirror of
https://github.com/bitwarden/server.git
synced 2025-07-01 16:12:49 -05:00
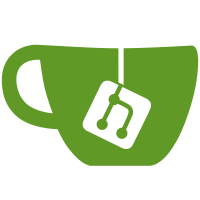
* captcha scores * some api fixes * check bot on captcha attribute * Update src/Core/Services/Implementations/HCaptchaValidationService.cs Co-authored-by: e271828- <e271828-@users.noreply.github.com> Co-authored-by: Chad Scharf <3904944+cscharf@users.noreply.github.com> Co-authored-by: e271828- <e271828-@users.noreply.github.com>
38 lines
1.6 KiB
C#
38 lines
1.6 KiB
C#
using Bit.Core.Context;
|
|
using Bit.Core.Exceptions;
|
|
using Bit.Core.Models.Api;
|
|
using Bit.Core.Services;
|
|
using Microsoft.AspNetCore.Mvc.Filters;
|
|
using Microsoft.Extensions.DependencyInjection;
|
|
|
|
namespace Bit.Core.Utilities
|
|
{
|
|
public class CaptchaProtectedAttribute : ActionFilterAttribute
|
|
{
|
|
public string ModelParameterName { get; set; } = "model";
|
|
|
|
public override void OnActionExecuting(ActionExecutingContext context)
|
|
{
|
|
var currentContext = context.HttpContext.RequestServices.GetRequiredService<ICurrentContext>();
|
|
var captchaValidationService = context.HttpContext.RequestServices.GetRequiredService<ICaptchaValidationService>();
|
|
|
|
if (captchaValidationService.RequireCaptchaValidation(currentContext, null))
|
|
{
|
|
var captchaResponse = (context.ActionArguments[ModelParameterName] as ICaptchaProtectedModel)?.CaptchaResponse;
|
|
|
|
if (string.IsNullOrWhiteSpace(captchaResponse))
|
|
{
|
|
throw new BadRequestException(captchaValidationService.SiteKeyResponseKeyName, captchaValidationService.SiteKey);
|
|
}
|
|
|
|
var captchaValidationResponse = captchaValidationService.ValidateCaptchaResponseAsync(captchaResponse,
|
|
currentContext.IpAddress, null).GetAwaiter().GetResult();
|
|
if (!captchaValidationResponse.Success || captchaValidationResponse.IsBot)
|
|
{
|
|
throw new BadRequestException("Captcha is invalid. Please refresh and try again");
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|