mirror of
https://github.com/bitwarden/server.git
synced 2025-07-02 00:22:50 -05:00
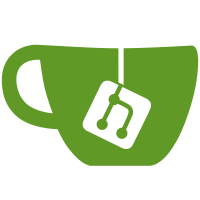
* PS-2390 Adding Id to the Collection/Folder RequestModel replacing folder/collection when they already exist instead of creating a new one Adding items to existing collections if the id matches * PS-2390 Improved Folder/Collection RequestModel code design * PS-2390 Removed whitespaces from FolderRequestModel * PS-2390 Verifying if folder/collection belongs to user/organization when updating or creating a new one * PS-2390 - Removed unnecessary null validation for Id on Folder/CollectionRequestModel * PS-2390 - Added bulk methods to get and update folders at import * PS-2390 - Added bulk methods to get and update collections at import org * PS-2390 - Corrected sqlproj path to Folder_ReadByIdsAndUserId * PS-2390 - Improved code readibility * PS-2390 - Added newlines to EOF * PS-2390 Remove logic to update folders/collections at import * PS-2390 - removed unnecessary methods and imports * PS-2390 - Removed unnecessary formatting change * PS-2390 - Removed unused variable
51 lines
1.3 KiB
C#
51 lines
1.3 KiB
C#
using System.ComponentModel.DataAnnotations;
|
|
using Bit.Core.Entities;
|
|
using Bit.Core.Utilities;
|
|
|
|
namespace Bit.Api.Models.Request;
|
|
|
|
public class CollectionRequestModel
|
|
{
|
|
[Required]
|
|
[EncryptedString]
|
|
[EncryptedStringLength(1000)]
|
|
public string Name { get; set; }
|
|
[StringLength(300)]
|
|
public string ExternalId { get; set; }
|
|
public IEnumerable<SelectionReadOnlyRequestModel> Groups { get; set; }
|
|
public IEnumerable<SelectionReadOnlyRequestModel> Users { get; set; }
|
|
|
|
public Collection ToCollection(Guid orgId)
|
|
{
|
|
return ToCollection(new Collection
|
|
{
|
|
OrganizationId = orgId
|
|
});
|
|
}
|
|
|
|
public virtual Collection ToCollection(Collection existingCollection)
|
|
{
|
|
existingCollection.Name = Name;
|
|
existingCollection.ExternalId = ExternalId;
|
|
return existingCollection;
|
|
}
|
|
}
|
|
|
|
public class CollectionBulkDeleteRequestModel
|
|
{
|
|
[Required]
|
|
public IEnumerable<string> Ids { get; set; }
|
|
public string OrganizationId { get; set; }
|
|
}
|
|
|
|
public class CollectionWithIdRequestModel : CollectionRequestModel
|
|
{
|
|
public Guid? Id { get; set; }
|
|
|
|
public override Collection ToCollection(Collection existingCollection)
|
|
{
|
|
existingCollection.Id = Id ?? Guid.Empty;
|
|
return base.ToCollection(existingCollection);
|
|
}
|
|
}
|