mirror of
https://github.com/bitwarden/server.git
synced 2025-04-04 12:40:22 -05:00
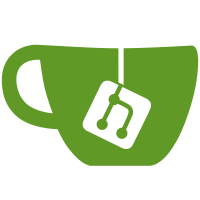
* Allow for binning of comb IDs by date and value * Introduce notification hub pool * Replace device type sharding with comb + range sharding * Fix proxy interface * Use enumerable services for multiServiceNotificationHub * Fix push interface usage * Fix push notification service dependencies * Fix push notification keys * Fixup documentation * Remove deprecated settings * Fix tests * PascalCase method names * Remove unused request model properties * Remove unused setting * Improve DateFromComb precision * Prefer readonly service enumerable * Pascal case template holes * Name TryParse methods TryParse * Apply suggestions from code review Co-authored-by: Justin Baur <19896123+justindbaur@users.noreply.github.com> * Include preferred push technology in config response SignalR will be the fallback, but clients should attempt web push first if offered and available to the client. * Register web push devices * Working signing and content encrypting * update to RFC-8291 and RFC-8188 * Notification hub is now working, no need to create our own * Fix body * Flip Success Check * use nifty json attribute * Remove vapid private key This is only needed to encrypt data for transmission along webpush -- it's handled by NotificationHub for us * Add web push feature flag to control config response * Update src/Core/NotificationHub/NotificationHubConnection.cs Co-authored-by: Justin Baur <19896123+justindbaur@users.noreply.github.com> * Update src/Core/NotificationHub/NotificationHubConnection.cs Co-authored-by: Justin Baur <19896123+justindbaur@users.noreply.github.com> * fixup! Update src/Core/NotificationHub/NotificationHubConnection.cs * Move to platform ownership * Remove debugging extension * Remove unused dependencies * Set json content directly * Name web push registration data * Fix FCM type typo * Determine specific feature flag from set of flags * Fixup merged tests * Fixup tests * Code quality suggestions * Fix merged tests * Fix test --------- Co-authored-by: Justin Baur <19896123+justindbaur@users.noreply.github.com>
133 lines
4.5 KiB
C#
133 lines
4.5 KiB
C#
using Bit.Core.Context;
|
|
using Bit.Core.Exceptions;
|
|
using Bit.Core.Models.Api;
|
|
using Bit.Core.NotificationHub;
|
|
using Bit.Core.Platform.Push;
|
|
using Bit.Core.Settings;
|
|
using Bit.Core.Utilities;
|
|
using Microsoft.AspNetCore.Authorization;
|
|
using Microsoft.AspNetCore.Mvc;
|
|
|
|
namespace Bit.Api.Platform.Push;
|
|
|
|
/// <summary>
|
|
/// Routes for push relay: functionality that facilitates communication
|
|
/// between self hosted organizations and Bitwarden cloud.
|
|
/// </summary>
|
|
[Route("push")]
|
|
[Authorize("Push")]
|
|
[SelfHosted(NotSelfHostedOnly = true)]
|
|
public class PushController : Controller
|
|
{
|
|
private readonly IPushRegistrationService _pushRegistrationService;
|
|
private readonly IPushNotificationService _pushNotificationService;
|
|
private readonly IWebHostEnvironment _environment;
|
|
private readonly ICurrentContext _currentContext;
|
|
private readonly IGlobalSettings _globalSettings;
|
|
|
|
public PushController(
|
|
IPushRegistrationService pushRegistrationService,
|
|
IPushNotificationService pushNotificationService,
|
|
IWebHostEnvironment environment,
|
|
ICurrentContext currentContext,
|
|
IGlobalSettings globalSettings)
|
|
{
|
|
_currentContext = currentContext;
|
|
_environment = environment;
|
|
_pushRegistrationService = pushRegistrationService;
|
|
_pushNotificationService = pushNotificationService;
|
|
_globalSettings = globalSettings;
|
|
}
|
|
|
|
[HttpPost("register")]
|
|
public async Task RegisterAsync([FromBody] PushRegistrationRequestModel model)
|
|
{
|
|
CheckUsage();
|
|
await _pushRegistrationService.CreateOrUpdateRegistrationAsync(new PushRegistrationData(model.PushToken), Prefix(model.DeviceId),
|
|
Prefix(model.UserId), Prefix(model.Identifier), model.Type, model.OrganizationIds.Select(Prefix), model.InstallationId);
|
|
}
|
|
|
|
[HttpPost("delete")]
|
|
public async Task DeleteAsync([FromBody] PushDeviceRequestModel model)
|
|
{
|
|
CheckUsage();
|
|
await _pushRegistrationService.DeleteRegistrationAsync(Prefix(model.Id));
|
|
}
|
|
|
|
[HttpPut("add-organization")]
|
|
public async Task AddOrganizationAsync([FromBody] PushUpdateRequestModel model)
|
|
{
|
|
CheckUsage();
|
|
await _pushRegistrationService.AddUserRegistrationOrganizationAsync(
|
|
model.Devices.Select(d => Prefix(d.Id)),
|
|
Prefix(model.OrganizationId));
|
|
}
|
|
|
|
[HttpPut("delete-organization")]
|
|
public async Task DeleteOrganizationAsync([FromBody] PushUpdateRequestModel model)
|
|
{
|
|
CheckUsage();
|
|
await _pushRegistrationService.DeleteUserRegistrationOrganizationAsync(
|
|
model.Devices.Select(d => Prefix(d.Id)),
|
|
Prefix(model.OrganizationId));
|
|
}
|
|
|
|
[HttpPost("send")]
|
|
public async Task SendAsync([FromBody] PushSendRequestModel model)
|
|
{
|
|
CheckUsage();
|
|
|
|
if (!string.IsNullOrWhiteSpace(model.InstallationId))
|
|
{
|
|
if (_currentContext.InstallationId!.Value.ToString() != model.InstallationId!)
|
|
{
|
|
throw new BadRequestException("InstallationId does not match current context.");
|
|
}
|
|
|
|
await _pushNotificationService.SendPayloadToInstallationAsync(
|
|
_currentContext.InstallationId.Value.ToString(), model.Type, model.Payload, Prefix(model.Identifier),
|
|
Prefix(model.DeviceId), model.ClientType);
|
|
}
|
|
else if (!string.IsNullOrWhiteSpace(model.UserId))
|
|
{
|
|
await _pushNotificationService.SendPayloadToUserAsync(Prefix(model.UserId),
|
|
model.Type, model.Payload, Prefix(model.Identifier), Prefix(model.DeviceId), model.ClientType);
|
|
}
|
|
else if (!string.IsNullOrWhiteSpace(model.OrganizationId))
|
|
{
|
|
await _pushNotificationService.SendPayloadToOrganizationAsync(Prefix(model.OrganizationId),
|
|
model.Type, model.Payload, Prefix(model.Identifier), Prefix(model.DeviceId), model.ClientType);
|
|
}
|
|
}
|
|
|
|
private string Prefix(string value)
|
|
{
|
|
if (string.IsNullOrWhiteSpace(value))
|
|
{
|
|
return null;
|
|
}
|
|
|
|
return $"{_currentContext.InstallationId!.Value}_{value}";
|
|
}
|
|
|
|
private void CheckUsage()
|
|
{
|
|
if (CanUse())
|
|
{
|
|
return;
|
|
}
|
|
|
|
throw new BadRequestException("Not correctly configured for push relays.");
|
|
}
|
|
|
|
private bool CanUse()
|
|
{
|
|
if (_environment.IsDevelopment())
|
|
{
|
|
return true;
|
|
}
|
|
|
|
return _currentContext.InstallationId.HasValue && !_globalSettings.SelfHosted;
|
|
}
|
|
}
|