mirror of
https://github.com/bitwarden/server.git
synced 2025-05-22 20:11:04 -05:00
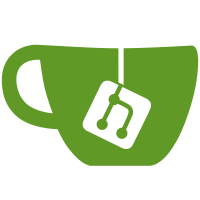
* PM-18939 refactoring send service to 'cqrs' * PM-18939 fixing import issue with sendValidationService * PM-18939 fixing code based on PR comments * PM-18339 reverting to previous code in test * PM-18939 adding XMLdocs to services * PM-18939 reverting send validation methods * PM-18939 updating code to match main * PM-18939 reverting validateUserCanSaveAsync to match main * PM-18939 fill our param and return sections of XMLdocs * PM-18939 updating XMLdocs based on PR comments * Update src/Core/Tools/SendFeatures/Commands/Interfaces/IAnonymousSendCommand.cs Co-authored-by: ✨ Audrey ✨ <ajensen@bitwarden.com> * Update src/Core/Tools/SendFeatures/Commands/Interfaces/INonAnonymousSendCommand.cs Co-authored-by: ✨ Audrey ✨ <ajensen@bitwarden.com> * Update src/Core/Tools/SendFeatures/Commands/Interfaces/INonAnonymousSendCommand.cs Co-authored-by: ✨ Audrey ✨ <ajensen@bitwarden.com> * Update src/Core/Tools/SendFeatures/Services/Interfaces/ISendStorageService.cs Co-authored-by: ✨ Audrey ✨ <ajensen@bitwarden.com> * PM-18939 adding commits to change tuple to enum type * PM-18939 resetting stream position to 0 when uploading file * PM-18939 updating XMLdocs based on PR comments * PM-18939 updating XMLdocs * PM-18939 removing circular dependency * PM-18939 fixing based on comments * PM-18939 updating method name and documentation --------- Co-authored-by: ✨ Audrey ✨ <ajensen@bitwarden.com>
119 lines
3.8 KiB
C#
119 lines
3.8 KiB
C#
using System.Text.Json;
|
|
using Bit.Core.Exceptions;
|
|
using Bit.Core.Platform.Push;
|
|
using Bit.Core.Tools.Entities;
|
|
using Bit.Core.Tools.Enums;
|
|
using Bit.Core.Tools.Models.Data;
|
|
using Bit.Core.Tools.Repositories;
|
|
using Bit.Core.Tools.SendFeatures.Commands;
|
|
using Bit.Core.Tools.Services;
|
|
using NSubstitute;
|
|
using Xunit;
|
|
|
|
namespace Bit.Core.Test.Tools.Services;
|
|
|
|
public class AnonymousSendCommandTests
|
|
{
|
|
private readonly ISendRepository _sendRepository;
|
|
private readonly ISendFileStorageService _sendFileStorageService;
|
|
private readonly IPushNotificationService _pushNotificationService;
|
|
private readonly ISendAuthorizationService _sendAuthorizationService;
|
|
private readonly AnonymousSendCommand _anonymousSendCommand;
|
|
|
|
public AnonymousSendCommandTests()
|
|
{
|
|
_sendRepository = Substitute.For<ISendRepository>();
|
|
_sendFileStorageService = Substitute.For<ISendFileStorageService>();
|
|
_pushNotificationService = Substitute.For<IPushNotificationService>();
|
|
_sendAuthorizationService = Substitute.For<ISendAuthorizationService>();
|
|
|
|
_anonymousSendCommand = new AnonymousSendCommand(
|
|
_sendRepository,
|
|
_sendFileStorageService,
|
|
_pushNotificationService,
|
|
_sendAuthorizationService);
|
|
}
|
|
|
|
[Fact]
|
|
public async Task GetSendFileDownloadUrlAsync_Success_ReturnsDownloadUrl()
|
|
{
|
|
// Arrange
|
|
var send = new Send
|
|
{
|
|
Id = Guid.NewGuid(),
|
|
Type = SendType.File,
|
|
AccessCount = 0,
|
|
Data = JsonSerializer.Serialize(new { Id = "fileId123" })
|
|
};
|
|
var fileId = "fileId123";
|
|
var password = "testPassword";
|
|
var expectedUrl = "https://example.com/download";
|
|
|
|
_sendAuthorizationService
|
|
.SendCanBeAccessed(send, password)
|
|
.Returns(SendAccessResult.Granted);
|
|
|
|
_sendFileStorageService
|
|
.GetSendFileDownloadUrlAsync(send, fileId)
|
|
.Returns(expectedUrl);
|
|
|
|
// Act
|
|
var result =
|
|
await _anonymousSendCommand.GetSendFileDownloadUrlAsync(send, fileId, password);
|
|
|
|
// Assert
|
|
Assert.Equal(expectedUrl, result.Item1);
|
|
Assert.Equal(1, send.AccessCount);
|
|
|
|
await _sendRepository.Received(1).ReplaceAsync(send);
|
|
await _pushNotificationService.Received(1).PushSyncSendUpdateAsync(send);
|
|
}
|
|
|
|
[Fact]
|
|
public async Task GetSendFileDownloadUrlAsync_AccessDenied_ReturnsNullWithReasons()
|
|
{
|
|
// Arrange
|
|
var send = new Send
|
|
{
|
|
Id = Guid.NewGuid(),
|
|
Type = SendType.File,
|
|
AccessCount = 0
|
|
};
|
|
var fileId = "fileId123";
|
|
var password = "wrongPassword";
|
|
|
|
_sendAuthorizationService
|
|
.SendCanBeAccessed(send, password)
|
|
.Returns(SendAccessResult.Denied);
|
|
|
|
// Act
|
|
var result =
|
|
await _anonymousSendCommand.GetSendFileDownloadUrlAsync(send, fileId, password);
|
|
|
|
// Assert
|
|
Assert.Null(result.Item1);
|
|
Assert.Equal(SendAccessResult.Denied, result.Item2);
|
|
Assert.Equal(0, send.AccessCount);
|
|
|
|
await _sendRepository.DidNotReceiveWithAnyArgs().ReplaceAsync(default);
|
|
await _pushNotificationService.DidNotReceiveWithAnyArgs().PushSyncSendUpdateAsync(default);
|
|
}
|
|
|
|
[Fact]
|
|
public async Task GetSendFileDownloadUrlAsync_NotFileSend_ThrowsBadRequestException()
|
|
{
|
|
// Arrange
|
|
var send = new Send
|
|
{
|
|
Id = Guid.NewGuid(),
|
|
Type = SendType.Text
|
|
};
|
|
var fileId = "fileId123";
|
|
var password = "testPassword";
|
|
|
|
// Act & Assert
|
|
await Assert.ThrowsAsync<BadRequestException>(() =>
|
|
_anonymousSendCommand.GetSendFileDownloadUrlAsync(send, fileId, password));
|
|
}
|
|
}
|