mirror of
https://github.com/bitwarden/server.git
synced 2025-05-22 20:11:04 -05:00
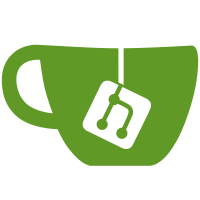
* PM-18939 refactoring send service to 'cqrs' * PM-18939 fixing import issue with sendValidationService * PM-18939 fixing code based on PR comments * PM-18339 reverting to previous code in test * PM-18939 adding XMLdocs to services * PM-18939 reverting send validation methods * PM-18939 updating code to match main * PM-18939 reverting validateUserCanSaveAsync to match main * PM-18939 fill our param and return sections of XMLdocs * PM-18939 updating XMLdocs based on PR comments * Update src/Core/Tools/SendFeatures/Commands/Interfaces/IAnonymousSendCommand.cs Co-authored-by: ✨ Audrey ✨ <ajensen@bitwarden.com> * Update src/Core/Tools/SendFeatures/Commands/Interfaces/INonAnonymousSendCommand.cs Co-authored-by: ✨ Audrey ✨ <ajensen@bitwarden.com> * Update src/Core/Tools/SendFeatures/Commands/Interfaces/INonAnonymousSendCommand.cs Co-authored-by: ✨ Audrey ✨ <ajensen@bitwarden.com> * Update src/Core/Tools/SendFeatures/Services/Interfaces/ISendStorageService.cs Co-authored-by: ✨ Audrey ✨ <ajensen@bitwarden.com> * PM-18939 adding commits to change tuple to enum type * PM-18939 resetting stream position to 0 when uploading file * PM-18939 updating XMLdocs based on PR comments * PM-18939 updating XMLdocs * PM-18939 removing circular dependency * PM-18939 fixing based on comments * PM-18939 updating method name and documentation --------- Co-authored-by: ✨ Audrey ✨ <ajensen@bitwarden.com>
176 lines
5.3 KiB
C#
176 lines
5.3 KiB
C#
using Bit.Core.Context;
|
|
using Bit.Core.Platform.Push;
|
|
using Bit.Core.Tools.Entities;
|
|
using Bit.Core.Tools.Models.Data;
|
|
using Bit.Core.Tools.Repositories;
|
|
using Bit.Core.Tools.Services;
|
|
using Microsoft.AspNetCore.Identity;
|
|
using NSubstitute;
|
|
using Xunit;
|
|
|
|
namespace Bit.Core.Test.Tools.Services;
|
|
|
|
public class SendAuthorizationServiceTests
|
|
{
|
|
private readonly ISendRepository _sendRepository;
|
|
private readonly IPasswordHasher<Bit.Core.Entities.User> _passwordHasher;
|
|
private readonly IPushNotificationService _pushNotificationService;
|
|
private readonly IReferenceEventService _referenceEventService;
|
|
private readonly ICurrentContext _currentContext;
|
|
private readonly SendAuthorizationService _sendAuthorizationService;
|
|
|
|
public SendAuthorizationServiceTests()
|
|
{
|
|
_sendRepository = Substitute.For<ISendRepository>();
|
|
_passwordHasher = Substitute.For<IPasswordHasher<Bit.Core.Entities.User>>();
|
|
_pushNotificationService = Substitute.For<IPushNotificationService>();
|
|
_referenceEventService = Substitute.For<IReferenceEventService>();
|
|
_currentContext = Substitute.For<ICurrentContext>();
|
|
|
|
_sendAuthorizationService = new SendAuthorizationService(
|
|
_sendRepository,
|
|
_passwordHasher,
|
|
_pushNotificationService,
|
|
_referenceEventService,
|
|
_currentContext);
|
|
}
|
|
|
|
|
|
[Fact]
|
|
public void SendCanBeAccessed_Success_ReturnsTrue()
|
|
{
|
|
// Arrange
|
|
var send = new Send
|
|
{
|
|
Id = Guid.NewGuid(),
|
|
UserId = Guid.NewGuid(),
|
|
MaxAccessCount = 10,
|
|
AccessCount = 5,
|
|
ExpirationDate = DateTime.UtcNow.AddYears(1),
|
|
DeletionDate = DateTime.UtcNow.AddYears(1),
|
|
Disabled = false,
|
|
Password = "hashedPassword123"
|
|
};
|
|
|
|
const string password = "TEST";
|
|
|
|
_passwordHasher
|
|
.VerifyHashedPassword(Arg.Any<Bit.Core.Entities.User>(), send.Password, password)
|
|
.Returns(PasswordVerificationResult.Success);
|
|
|
|
// Act
|
|
var result =
|
|
_sendAuthorizationService.SendCanBeAccessed(send, password);
|
|
|
|
// Assert
|
|
Assert.Equal(SendAccessResult.Granted, result);
|
|
}
|
|
|
|
[Fact]
|
|
public void SendCanBeAccessed_NullMaxAccess_Success()
|
|
{
|
|
// Arrange
|
|
var send = new Send
|
|
{
|
|
Id = Guid.NewGuid(),
|
|
UserId = Guid.NewGuid(),
|
|
MaxAccessCount = null,
|
|
AccessCount = 5,
|
|
ExpirationDate = DateTime.UtcNow.AddYears(1),
|
|
DeletionDate = DateTime.UtcNow.AddYears(1),
|
|
Disabled = false,
|
|
Password = "hashedPassword123"
|
|
};
|
|
|
|
const string password = "TEST";
|
|
|
|
_passwordHasher
|
|
.VerifyHashedPassword(Arg.Any<Bit.Core.Entities.User>(), send.Password, password)
|
|
.Returns(PasswordVerificationResult.Success);
|
|
|
|
// Act
|
|
var result = _sendAuthorizationService.SendCanBeAccessed(send, password);
|
|
|
|
// Assert
|
|
Assert.Equal(SendAccessResult.Granted, result);
|
|
}
|
|
|
|
[Fact]
|
|
public void SendCanBeAccessed_NullSend_DoesNotGrantAccess()
|
|
{
|
|
// Arrange
|
|
_passwordHasher
|
|
.VerifyHashedPassword(Arg.Any<Bit.Core.Entities.User>(), "TEST", "TEST")
|
|
.Returns(PasswordVerificationResult.Success);
|
|
|
|
// Act
|
|
var result =
|
|
_sendAuthorizationService.SendCanBeAccessed(null, "TEST");
|
|
|
|
// Assert
|
|
Assert.Equal(SendAccessResult.Denied, result);
|
|
}
|
|
|
|
[Fact]
|
|
public void SendCanBeAccessed_RehashNeeded_RehashesPassword()
|
|
{
|
|
// Arrange
|
|
var now = DateTime.UtcNow;
|
|
var send = new Send
|
|
{
|
|
Id = Guid.NewGuid(),
|
|
UserId = Guid.NewGuid(),
|
|
MaxAccessCount = null,
|
|
AccessCount = 5,
|
|
ExpirationDate = now.AddYears(1),
|
|
DeletionDate = now.AddYears(1),
|
|
Disabled = false,
|
|
Password = "TEST"
|
|
};
|
|
|
|
_passwordHasher
|
|
.VerifyHashedPassword(Arg.Any<Bit.Core.Entities.User>(), "TEST", "TEST")
|
|
.Returns(PasswordVerificationResult.SuccessRehashNeeded);
|
|
|
|
// Act
|
|
var result =
|
|
_sendAuthorizationService.SendCanBeAccessed(send, "TEST");
|
|
|
|
// Assert
|
|
_passwordHasher
|
|
.Received(1)
|
|
.HashPassword(Arg.Any<Bit.Core.Entities.User>(), "TEST");
|
|
|
|
Assert.Equal(SendAccessResult.Granted, result);
|
|
}
|
|
|
|
[Fact]
|
|
public void SendCanBeAccessed_VerifyFailed_PasswordInvalidReturnsTrue()
|
|
{
|
|
// Arrange
|
|
var now = DateTime.UtcNow;
|
|
var send = new Send
|
|
{
|
|
Id = Guid.NewGuid(),
|
|
UserId = Guid.NewGuid(),
|
|
MaxAccessCount = null,
|
|
AccessCount = 5,
|
|
ExpirationDate = now.AddYears(1),
|
|
DeletionDate = now.AddYears(1),
|
|
Disabled = false,
|
|
Password = "TEST"
|
|
};
|
|
|
|
_passwordHasher
|
|
.VerifyHashedPassword(Arg.Any<Bit.Core.Entities.User>(), "TEST", "TEST")
|
|
.Returns(PasswordVerificationResult.Failed);
|
|
|
|
// Act
|
|
var result =
|
|
_sendAuthorizationService.SendCanBeAccessed(send, "TEST");
|
|
|
|
// Assert
|
|
Assert.Equal(SendAccessResult.PasswordInvalid, result);
|
|
}
|
|
}
|