mirror of
https://github.com/bitwarden/server.git
synced 2025-05-22 20:11:04 -05:00
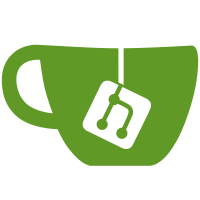
* [NO LOGIC] Organize Billing provider code * Run dotnet format * Run dotnet format' * Fixed using after merge * Fixed test usings after merge
33 lines
1.1 KiB
C#
33 lines
1.1 KiB
C#
using AutoMapper;
|
|
using Bit.Core.Billing.Providers.Entities;
|
|
using Bit.Core.Billing.Providers.Repositories;
|
|
using Bit.Infrastructure.EntityFramework.Repositories;
|
|
using Microsoft.EntityFrameworkCore;
|
|
using Microsoft.Extensions.DependencyInjection;
|
|
using EFProviderPlan = Bit.Infrastructure.EntityFramework.Billing.Models.ProviderPlan;
|
|
|
|
namespace Bit.Infrastructure.EntityFramework.Billing.Repositories;
|
|
|
|
public class ProviderPlanRepository(
|
|
IMapper mapper,
|
|
IServiceScopeFactory serviceScopeFactory)
|
|
: Repository<ProviderPlan, EFProviderPlan, Guid>(
|
|
serviceScopeFactory,
|
|
mapper,
|
|
context => context.ProviderPlans), IProviderPlanRepository
|
|
{
|
|
public async Task<ICollection<ProviderPlan>> GetByProviderId(Guid providerId)
|
|
{
|
|
using var serviceScope = ServiceScopeFactory.CreateScope();
|
|
|
|
var databaseContext = GetDatabaseContext(serviceScope);
|
|
|
|
var query =
|
|
from providerPlan in databaseContext.ProviderPlans
|
|
where providerPlan.ProviderId == providerId
|
|
select providerPlan;
|
|
|
|
return await query.ToArrayAsync();
|
|
}
|
|
}
|