mirror of
https://github.com/bitwarden/server.git
synced 2025-04-07 14:08:13 -05:00
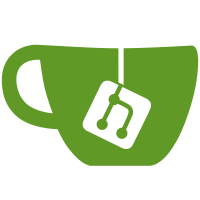
* Get limited life attachment download URL This change limits url download to a 1min lifetime. This requires moving to a new container to allow for non-public blob access. Clients will have to call GetAttachmentData api function to receive the download URL. For backwards compatibility, attachment URLs are still present, but will not work for attachments stored in non-public access blobs. * Make GlobalSettings interface for testing * Test LocalAttachmentStorageService equivalence * Remove comment * Add missing globalSettings using * Simplify default attachment container * Default to attachments containe for existing methods A new upload method will be made for uploading to attachments-v2. For compatibility for clients which don't use these new methods, we need to still use the old container. The new container will be used only for new uploads * Remove Default MetaData fixture. * Keep attachments container blob-level security for all instances * Close unclosed FileStream * Favor default value for noop services
103 lines
3.6 KiB
C#
103 lines
3.6 KiB
C#
using System;
|
|
using System.Threading.Tasks;
|
|
using Microsoft.AspNetCore.Mvc;
|
|
using Microsoft.AspNetCore.Authorization;
|
|
using System.Net.Http;
|
|
using System.Security.Cryptography;
|
|
using Bit.Core.Services;
|
|
using Bit.Core.Context;
|
|
using System.Net;
|
|
using Bit.Core.Exceptions;
|
|
using System.Linq;
|
|
using Bit.Core.Utilities;
|
|
using Bit.Core.Settings;
|
|
|
|
namespace Bit.Api.Controllers
|
|
{
|
|
[Route("hibp")]
|
|
[Authorize("Application")]
|
|
public class HibpController : Controller
|
|
{
|
|
private const string HibpBreachApi = "https://haveibeenpwned.com/api/v3/breachedaccount/{0}" +
|
|
"?truncateResponse=false&includeUnverified=false";
|
|
private static HttpClient _httpClient;
|
|
|
|
private readonly IUserService _userService;
|
|
private readonly ICurrentContext _currentContext;
|
|
private readonly GlobalSettings _globalSettings;
|
|
private readonly string _userAgent;
|
|
|
|
static HibpController()
|
|
{
|
|
_httpClient = new HttpClient();
|
|
}
|
|
|
|
public HibpController(
|
|
IUserService userService,
|
|
ICurrentContext currentContext,
|
|
GlobalSettings globalSettings)
|
|
{
|
|
_userService = userService;
|
|
_currentContext = currentContext;
|
|
_globalSettings = globalSettings;
|
|
_userAgent = _globalSettings.SelfHosted ? "Bitwarden Self-Hosted" : "Bitwarden";
|
|
}
|
|
|
|
[HttpGet("breach")]
|
|
public async Task<IActionResult> Get(string username)
|
|
{
|
|
return await SendAsync(WebUtility.UrlEncode(username), true);
|
|
}
|
|
|
|
private async Task<IActionResult> SendAsync(string username, bool retry)
|
|
{
|
|
if (!CoreHelpers.SettingHasValue(_globalSettings.HibpApiKey))
|
|
{
|
|
throw new BadRequestException("HaveIBeenPwned API key not set.");
|
|
}
|
|
var request = new HttpRequestMessage(HttpMethod.Get, string.Format(HibpBreachApi, username));
|
|
request.Headers.Add("hibp-api-key", _globalSettings.HibpApiKey);
|
|
request.Headers.Add("hibp-client-id", GetClientId());
|
|
request.Headers.Add("User-Agent", _userAgent);
|
|
var response = await _httpClient.SendAsync(request);
|
|
if (response.IsSuccessStatusCode)
|
|
{
|
|
var data = await response.Content.ReadAsStringAsync();
|
|
return Content(data, "application/json");
|
|
}
|
|
else if (response.StatusCode == HttpStatusCode.NotFound)
|
|
{
|
|
return new NotFoundResult();
|
|
}
|
|
else if (response.StatusCode == HttpStatusCode.TooManyRequests && retry)
|
|
{
|
|
var delay = 2000;
|
|
if (response.Headers.Contains("retry-after"))
|
|
{
|
|
var vals = response.Headers.GetValues("retry-after");
|
|
if (vals.Any() && int.TryParse(vals.FirstOrDefault(), out var secDelay))
|
|
{
|
|
delay = (secDelay * 1000) + 200;
|
|
}
|
|
}
|
|
await Task.Delay(delay);
|
|
return await SendAsync(username, false);
|
|
}
|
|
else
|
|
{
|
|
throw new BadRequestException("Request failed. Status code: " + response.StatusCode);
|
|
}
|
|
}
|
|
|
|
private string GetClientId()
|
|
{
|
|
var userId = _userService.GetProperUserId(User).Value;
|
|
using (var sha256 = SHA256.Create())
|
|
{
|
|
var hash = sha256.ComputeHash(userId.ToByteArray());
|
|
return Convert.ToBase64String(hash);
|
|
}
|
|
}
|
|
}
|
|
}
|