mirror of
https://github.com/bitwarden/server.git
synced 2025-07-18 16:11:28 -05:00
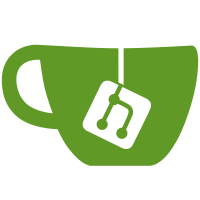
* [PM-17562] Slack Event Investigation * Refactored Slack and Webhook integrations to pull configurations dynamically from a new Repository * Added new TemplateProcessor and added/updated unit tests * SlackService improvements, testing, integration configurations * Refactor SlackService to use a dedicated model to parse responses * Refactored SlackOAuthController to use SlackService as an injected dependency; added tests for SlackService * Remove unnecessary methods from the IOrganizationIntegrationConfigurationRepository * Moved Slack OAuth to take into account the Organization it's being stored for. Added methods to store the top level integration for Slack * Organization integrations and configuration database schemas * Format EF files * Initial buildout of basic repositories * [PM-17562] Add Dapper Repositories For Organization Integrations and Configurations * Update Slack and Webhook handlers to use new Repositories * Update SlackOAuth tests to new signatures * Added EF Repositories * Update handlers to use latest repositories * [PM-17562] Add Dapper and EF Repositories For Ogranization Integrations and Configurations * Updated with changes from PR comments * Adjusted Handlers to new repository method names; updated tests to naming convention * Adjust URL structure; add delete for Slack, add tests * Added Webhook Integration Controller * Add tests for WebhookIntegrationController * Added Create/Delete for OrganizationIntegrationConfigurations * Prepend ConnectionTypes into IntegrationType so we don't run into issues later * Added Update to OrganizationIntegrationConfigurtionController * Moved Webhook-specific integration code to being a generic controller for everything but Slack * Removed delete from SlackController - Deletes should happen through the normal Integration controller * Fixed SlackController, reworked OIC Controller to use ids from URL and update the returned object * Added parse/type checking for integration and integration configuration JSONs, Cleaned up GlobalSettings to remove old values * Cleanup and fixes for Azure Service Bus support * Clean up naming on TemplateProcessorTests * Address SonarQube warnings/suggestions * Expanded test coverage; Cleaned up tests * Respond to PR Feedback * Rename TemplateProcessor to IntegrationTemplateProcessor --------- Co-authored-by: Matt Bishop <mbishop@bitwarden.com>
202 lines
8.6 KiB
C#
202 lines
8.6 KiB
C#
using Bit.Api.AdminConsole.Controllers;
|
|
using Bit.Api.AdminConsole.Models.Request.Organizations;
|
|
using Bit.Api.AdminConsole.Models.Response.Organizations;
|
|
using Bit.Core.AdminConsole.Entities;
|
|
using Bit.Core.Context;
|
|
using Bit.Core.Enums;
|
|
using Bit.Core.Exceptions;
|
|
using Bit.Core.Repositories;
|
|
using Bit.Test.Common.AutoFixture;
|
|
using Bit.Test.Common.AutoFixture.Attributes;
|
|
using Microsoft.AspNetCore.Mvc;
|
|
using NSubstitute;
|
|
using NSubstitute.ReturnsExtensions;
|
|
using Xunit;
|
|
|
|
namespace Bit.Api.Test.AdminConsole.Controllers;
|
|
|
|
[ControllerCustomize(typeof(OrganizationIntegrationController))]
|
|
[SutProviderCustomize]
|
|
public class OrganizationIntegrationControllerTests
|
|
{
|
|
private OrganizationIntegrationRequestModel _webhookRequestModel = new OrganizationIntegrationRequestModel()
|
|
{
|
|
Configuration = null,
|
|
Type = IntegrationType.Webhook
|
|
};
|
|
|
|
[Theory, BitAutoData]
|
|
public async Task CreateAsync_Webhook_AllParamsProvided_Succeeds(
|
|
SutProvider<OrganizationIntegrationController> sutProvider,
|
|
Guid organizationId)
|
|
{
|
|
sutProvider.Sut.Url = Substitute.For<IUrlHelper>();
|
|
sutProvider.GetDependency<ICurrentContext>()
|
|
.OrganizationOwner(organizationId)
|
|
.Returns(true);
|
|
sutProvider.GetDependency<IOrganizationIntegrationRepository>()
|
|
.CreateAsync(Arg.Any<OrganizationIntegration>())
|
|
.Returns(callInfo => callInfo.Arg<OrganizationIntegration>());
|
|
var response = await sutProvider.Sut.CreateAsync(organizationId, _webhookRequestModel);
|
|
|
|
await sutProvider.GetDependency<IOrganizationIntegrationRepository>().Received(1)
|
|
.CreateAsync(Arg.Any<OrganizationIntegration>());
|
|
Assert.IsType<OrganizationIntegrationResponseModel>(response);
|
|
Assert.Equal(IntegrationType.Webhook, response.Type);
|
|
}
|
|
|
|
[Theory, BitAutoData]
|
|
public async Task CreateAsync_UserIsNotOrganizationAdmin_ThrowsNotFound(SutProvider<OrganizationIntegrationController> sutProvider, Guid organizationId)
|
|
{
|
|
sutProvider.Sut.Url = Substitute.For<IUrlHelper>();
|
|
sutProvider.GetDependency<ICurrentContext>()
|
|
.OrganizationOwner(organizationId)
|
|
.Returns(false);
|
|
|
|
await Assert.ThrowsAsync<NotFoundException>(async () => await sutProvider.Sut.CreateAsync(organizationId, _webhookRequestModel));
|
|
}
|
|
|
|
[Theory, BitAutoData]
|
|
public async Task DeleteAsync_AllParamsProvided_Succeeds(
|
|
SutProvider<OrganizationIntegrationController> sutProvider,
|
|
Guid organizationId,
|
|
OrganizationIntegration organizationIntegration)
|
|
{
|
|
organizationIntegration.OrganizationId = organizationId;
|
|
sutProvider.Sut.Url = Substitute.For<IUrlHelper>();
|
|
sutProvider.GetDependency<ICurrentContext>()
|
|
.OrganizationOwner(organizationId)
|
|
.Returns(true);
|
|
sutProvider.GetDependency<IOrganizationIntegrationRepository>()
|
|
.GetByIdAsync(Arg.Any<Guid>())
|
|
.Returns(organizationIntegration);
|
|
|
|
await sutProvider.Sut.DeleteAsync(organizationId, organizationIntegration.Id);
|
|
|
|
await sutProvider.GetDependency<IOrganizationIntegrationRepository>().Received(1)
|
|
.GetByIdAsync(organizationIntegration.Id);
|
|
await sutProvider.GetDependency<IOrganizationIntegrationRepository>().Received(1)
|
|
.DeleteAsync(organizationIntegration);
|
|
}
|
|
|
|
[Theory, BitAutoData]
|
|
public async Task DeleteAsync_IntegrationDoesNotBelongToOrganization_ThrowsNotFound(
|
|
SutProvider<OrganizationIntegrationController> sutProvider,
|
|
Guid organizationId,
|
|
OrganizationIntegration organizationIntegration)
|
|
{
|
|
organizationIntegration.OrganizationId = Guid.NewGuid();
|
|
sutProvider.Sut.Url = Substitute.For<IUrlHelper>();
|
|
sutProvider.GetDependency<ICurrentContext>()
|
|
.OrganizationOwner(organizationId)
|
|
.Returns(true);
|
|
sutProvider.GetDependency<IOrganizationIntegrationRepository>()
|
|
.GetByIdAsync(Arg.Any<Guid>())
|
|
.ReturnsNull();
|
|
|
|
await Assert.ThrowsAsync<NotFoundException>(async () => await sutProvider.Sut.DeleteAsync(organizationId, Guid.Empty));
|
|
}
|
|
|
|
[Theory, BitAutoData]
|
|
public async Task DeleteAsync_IntegrationDoesNotExist_ThrowsNotFound(
|
|
SutProvider<OrganizationIntegrationController> sutProvider,
|
|
Guid organizationId)
|
|
{
|
|
sutProvider.Sut.Url = Substitute.For<IUrlHelper>();
|
|
sutProvider.GetDependency<ICurrentContext>()
|
|
.OrganizationOwner(organizationId)
|
|
.Returns(true);
|
|
sutProvider.GetDependency<IOrganizationIntegrationRepository>()
|
|
.GetByIdAsync(Arg.Any<Guid>())
|
|
.ReturnsNull();
|
|
|
|
await Assert.ThrowsAsync<NotFoundException>(async () => await sutProvider.Sut.DeleteAsync(organizationId, Guid.Empty));
|
|
}
|
|
|
|
[Theory, BitAutoData]
|
|
public async Task DeleteAsync_UserIsNotOrganizationAdmin_ThrowsNotFound(
|
|
SutProvider<OrganizationIntegrationController> sutProvider,
|
|
Guid organizationId)
|
|
{
|
|
sutProvider.Sut.Url = Substitute.For<IUrlHelper>();
|
|
sutProvider.GetDependency<ICurrentContext>()
|
|
.OrganizationOwner(organizationId)
|
|
.Returns(false);
|
|
|
|
await Assert.ThrowsAsync<NotFoundException>(async () => await sutProvider.Sut.DeleteAsync(organizationId, Guid.Empty));
|
|
}
|
|
|
|
[Theory, BitAutoData]
|
|
public async Task UpdateAsync_AllParamsProvided_Succeeds(
|
|
SutProvider<OrganizationIntegrationController> sutProvider,
|
|
Guid organizationId,
|
|
OrganizationIntegration organizationIntegration)
|
|
{
|
|
organizationIntegration.OrganizationId = organizationId;
|
|
organizationIntegration.Type = IntegrationType.Webhook;
|
|
sutProvider.Sut.Url = Substitute.For<IUrlHelper>();
|
|
sutProvider.GetDependency<ICurrentContext>()
|
|
.OrganizationOwner(organizationId)
|
|
.Returns(true);
|
|
sutProvider.GetDependency<IOrganizationIntegrationRepository>()
|
|
.GetByIdAsync(Arg.Any<Guid>())
|
|
.Returns(organizationIntegration);
|
|
|
|
var response = await sutProvider.Sut.UpdateAsync(organizationId, organizationIntegration.Id, _webhookRequestModel);
|
|
|
|
await sutProvider.GetDependency<IOrganizationIntegrationRepository>().Received(1)
|
|
.GetByIdAsync(organizationIntegration.Id);
|
|
await sutProvider.GetDependency<IOrganizationIntegrationRepository>().Received(1)
|
|
.ReplaceAsync(organizationIntegration);
|
|
Assert.IsType<OrganizationIntegrationResponseModel>(response);
|
|
Assert.Equal(IntegrationType.Webhook, response.Type);
|
|
}
|
|
|
|
[Theory, BitAutoData]
|
|
public async Task UpdateAsync_IntegrationDoesNotBelongToOrganization_ThrowsNotFound(
|
|
SutProvider<OrganizationIntegrationController> sutProvider,
|
|
Guid organizationId,
|
|
OrganizationIntegration organizationIntegration)
|
|
{
|
|
organizationIntegration.OrganizationId = Guid.NewGuid();
|
|
sutProvider.Sut.Url = Substitute.For<IUrlHelper>();
|
|
sutProvider.GetDependency<ICurrentContext>()
|
|
.OrganizationOwner(organizationId)
|
|
.Returns(true);
|
|
sutProvider.GetDependency<IOrganizationIntegrationRepository>()
|
|
.GetByIdAsync(Arg.Any<Guid>())
|
|
.ReturnsNull();
|
|
|
|
await Assert.ThrowsAsync<NotFoundException>(async () => await sutProvider.Sut.UpdateAsync(organizationId, Guid.Empty, _webhookRequestModel));
|
|
}
|
|
|
|
[Theory, BitAutoData]
|
|
public async Task UpdateAsync_IntegrationDoesNotExist_ThrowsNotFound(
|
|
SutProvider<OrganizationIntegrationController> sutProvider,
|
|
Guid organizationId)
|
|
{
|
|
sutProvider.Sut.Url = Substitute.For<IUrlHelper>();
|
|
sutProvider.GetDependency<ICurrentContext>()
|
|
.OrganizationOwner(organizationId)
|
|
.Returns(true);
|
|
sutProvider.GetDependency<IOrganizationIntegrationRepository>()
|
|
.GetByIdAsync(Arg.Any<Guid>())
|
|
.ReturnsNull();
|
|
|
|
await Assert.ThrowsAsync<NotFoundException>(async () => await sutProvider.Sut.UpdateAsync(organizationId, Guid.Empty, _webhookRequestModel));
|
|
}
|
|
|
|
[Theory, BitAutoData]
|
|
public async Task UpdateAsync_UserIsNotOrganizationAdmin_ThrowsNotFound(
|
|
SutProvider<OrganizationIntegrationController> sutProvider,
|
|
Guid organizationId)
|
|
{
|
|
sutProvider.Sut.Url = Substitute.For<IUrlHelper>();
|
|
sutProvider.GetDependency<ICurrentContext>()
|
|
.OrganizationOwner(organizationId)
|
|
.Returns(false);
|
|
|
|
await Assert.ThrowsAsync<NotFoundException>(async () => await sutProvider.Sut.UpdateAsync(organizationId, Guid.Empty, _webhookRequestModel));
|
|
}
|
|
}
|