mirror of
https://github.com/bitwarden/server.git
synced 2025-04-05 05:00:19 -05:00
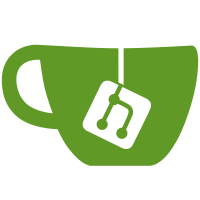
* Add send HideEmail to tables and models * Respect HideEmail setting for Sends * Recreate SendView to include new HideEmail column * Enforce new Send policy * Insert default value for new HideEmail column * Delete c95d7598-71cc-4eab-8b08-aced0045198b.json * Remove unrelated files * Revert disableSendPolicy, add sendOptionsPolicy * Minor style fixes * Update SQL project with Send.HideEmail column * unit test SendOptionsPolicy.DisableHideEmail * Add SendOptionsPolicy to Portal * Make HideEmail nullable, fix migrator script * Remove NOT NULL constraint from HideEmail * Fix style * Make HideEmail nullable * minor fixes to model and error message * Move SendOptionsExemption banner Co-authored-by: Chad Scharf <3904944+cscharf@users.noreply.github.com>
128 lines
4.9 KiB
C#
128 lines
4.9 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text.Json;
|
|
using Bit.Core.Enums;
|
|
using Bit.Core.Models.Table;
|
|
using Bit.Core.Models.Data;
|
|
using Bit.Core.Services;
|
|
using Microsoft.AspNetCore.Mvc.Rendering;
|
|
|
|
namespace Bit.Portal.Models
|
|
{
|
|
public class PolicyEditModel : PolicyModel
|
|
{
|
|
public PolicyEditModel() { }
|
|
|
|
public PolicyEditModel(PolicyType type, II18nService i18nService)
|
|
: base(type, false)
|
|
{
|
|
// Inject service and create static lists
|
|
TranslateStrings(i18nService);
|
|
}
|
|
|
|
public PolicyEditModel(Policy model, II18nService i18nService)
|
|
: base(model)
|
|
{
|
|
if (model == null)
|
|
{
|
|
return;
|
|
}
|
|
|
|
// Inject service and create static lists
|
|
TranslateStrings(i18nService);
|
|
|
|
if (model.Data != null)
|
|
{
|
|
var options = new JsonSerializerOptions
|
|
{
|
|
PropertyNameCaseInsensitive = true,
|
|
};
|
|
|
|
switch (model.Type)
|
|
{
|
|
case PolicyType.MasterPassword:
|
|
MasterPasswordDataModel = JsonSerializer.Deserialize<MasterPasswordDataModel>(model.Data, options);
|
|
break;
|
|
case PolicyType.PasswordGenerator:
|
|
PasswordGeneratorDataModel = JsonSerializer.Deserialize<PasswordGeneratorDataModel>(model.Data, options);
|
|
break;
|
|
case PolicyType.SendOptions:
|
|
SendOptionsDataModel = JsonSerializer.Deserialize<SendOptionsPolicyData>(model.Data, options);
|
|
break;
|
|
default:
|
|
throw new ArgumentOutOfRangeException();
|
|
}
|
|
}
|
|
}
|
|
|
|
public MasterPasswordDataModel MasterPasswordDataModel { get; set; }
|
|
public PasswordGeneratorDataModel PasswordGeneratorDataModel { get; set; }
|
|
public SendOptionsPolicyData SendOptionsDataModel { get; set; }
|
|
public List<SelectListItem> Complexities { get; set; }
|
|
public List<SelectListItem> DefaultTypes { get; set; }
|
|
public string EnableCheckboxText { get; set; }
|
|
|
|
public Policy ToPolicy(PolicyType type, Guid organizationId)
|
|
{
|
|
return ToPolicy(new Policy
|
|
{
|
|
Type = type,
|
|
OrganizationId = organizationId
|
|
});
|
|
}
|
|
|
|
public Policy ToPolicy(Policy existingPolicy)
|
|
{
|
|
existingPolicy.Enabled = Enabled;
|
|
|
|
var options = new JsonSerializerOptions
|
|
{
|
|
PropertyNamingPolicy = JsonNamingPolicy.CamelCase,
|
|
};
|
|
switch (existingPolicy.Type)
|
|
{
|
|
case PolicyType.MasterPassword:
|
|
existingPolicy.Data = JsonSerializer.Serialize(MasterPasswordDataModel, options);
|
|
break;
|
|
case PolicyType.PasswordGenerator:
|
|
existingPolicy.Data = JsonSerializer.Serialize(PasswordGeneratorDataModel, options);
|
|
break;
|
|
case PolicyType.SingleOrg:
|
|
case PolicyType.TwoFactorAuthentication:
|
|
case PolicyType.RequireSso:
|
|
case PolicyType.PersonalOwnership:
|
|
case PolicyType.DisableSend:
|
|
break;
|
|
case PolicyType.SendOptions:
|
|
existingPolicy.Data = JsonSerializer.Serialize(SendOptionsDataModel, options);
|
|
break;
|
|
default:
|
|
throw new ArgumentOutOfRangeException();
|
|
}
|
|
|
|
return existingPolicy;
|
|
}
|
|
|
|
public void TranslateStrings(II18nService i18nService)
|
|
{
|
|
Complexities = new List<SelectListItem>
|
|
{
|
|
new SelectListItem { Value = null, Text = "--" + i18nService.T("Select") + "--"},
|
|
new SelectListItem { Value = "0", Text = i18nService.T("Weak") + " (0)" },
|
|
new SelectListItem { Value = "1", Text = i18nService.T("Weak") + " (1)" },
|
|
new SelectListItem { Value = "2", Text = i18nService.T("Weak") + " (2)" },
|
|
new SelectListItem { Value = "3", Text = i18nService.T("Good") + " (3)" },
|
|
new SelectListItem { Value = "4", Text = i18nService.T("Strong") + " (4)" },
|
|
};
|
|
DefaultTypes = new List<SelectListItem>
|
|
{
|
|
new SelectListItem { Value = null, Text = i18nService.T("UserPreference") },
|
|
new SelectListItem { Value = "password", Text = i18nService.T("Password") },
|
|
new SelectListItem { Value = "passphrase", Text = i18nService.T("Passphrase") },
|
|
};
|
|
EnableCheckboxText = PolicyType == PolicyType.PersonalOwnership
|
|
? i18nService.T("PersonalOwnershipCheckboxDesc") : i18nService.T("Enabled");
|
|
}
|
|
}
|
|
}
|