mirror of
https://github.com/bitwarden/server.git
synced 2025-04-11 08:08:14 -05:00
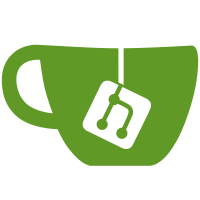
* Start switch to System.Text.Json * Work on switching to System.Text.Json * Main work on STJ refactor * Fix build errors * Run formatting * Delete unused file * Use legacy for two factor providers * Run formatter * Add TokenProviderTests * Run formatting * Fix merge issues * Switch to use JsonSerializer * Address PR feedback * Fix formatting * Ran formatter * Switch to async * Ensure Enums are serialized as strings * Fix formatting * Enqueue single items as arrays * Remove CreateAsync method on AzureQueueService
52 lines
1.5 KiB
C#
52 lines
1.5 KiB
C#
using System;
|
|
using System.Text;
|
|
using System.Text.Json;
|
|
using System.Threading.Tasks;
|
|
using Azure.Storage.Queues;
|
|
using Bit.Core.Models.Business;
|
|
using Bit.Core.Settings;
|
|
using Bit.Core.Utilities;
|
|
|
|
namespace Bit.Core.Services
|
|
{
|
|
public class AzureQueueReferenceEventService : IReferenceEventService
|
|
{
|
|
private const string _queueName = "reference-events";
|
|
|
|
private readonly QueueClient _queueClient;
|
|
private readonly GlobalSettings _globalSettings;
|
|
|
|
public AzureQueueReferenceEventService(
|
|
GlobalSettings globalSettings)
|
|
{
|
|
_queueClient = new QueueClient(globalSettings.Events.ConnectionString, _queueName);
|
|
_globalSettings = globalSettings;
|
|
}
|
|
|
|
public async Task RaiseEventAsync(ReferenceEvent referenceEvent)
|
|
{
|
|
await SendMessageAsync(referenceEvent);
|
|
}
|
|
|
|
private async Task SendMessageAsync(ReferenceEvent referenceEvent)
|
|
{
|
|
if (_globalSettings.SelfHosted)
|
|
{
|
|
// Ignore for self-hosted
|
|
return;
|
|
}
|
|
try
|
|
{
|
|
var message = JsonSerializer.Serialize(referenceEvent, JsonHelpers.IgnoreWritingNullAndCamelCase);
|
|
// Messages need to be base64 encoded
|
|
var encodedMessage = Convert.ToBase64String(Encoding.UTF8.GetBytes(message));
|
|
await _queueClient.SendMessageAsync(encodedMessage);
|
|
}
|
|
catch
|
|
{
|
|
// Ignore failure
|
|
}
|
|
}
|
|
}
|
|
}
|