mirror of
https://github.com/bitwarden/server.git
synced 2025-04-05 05:00:19 -05:00
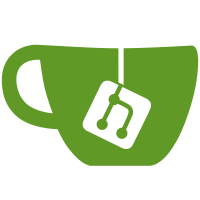
* Renamed ProductType to ProductTierType * Renamed Product properties to ProductTier * Moved ProductTierType to Bit.Core.Billing.Enums namespace from Bit.Core.Enums * Moved PlanType enum to Bit.Core.Billing.Enums * Moved StaticStore to Bit.Core.Billing.Models.StaticStore namespace * Added ProductType enum * dotnet format
62 lines
1.8 KiB
C#
62 lines
1.8 KiB
C#
using Bit.Core.Billing.Enums;
|
|
using Bit.Core.Billing.Extensions;
|
|
using Stripe;
|
|
|
|
using static Bit.Core.Billing.Utilities;
|
|
|
|
namespace Bit.Core.Models.Business;
|
|
|
|
public class ProviderSubscriptionUpdate : SubscriptionUpdate
|
|
{
|
|
private readonly string _planId;
|
|
private readonly int _previouslyPurchasedSeats;
|
|
private readonly int _newlyPurchasedSeats;
|
|
|
|
protected override List<string> PlanIds => [_planId];
|
|
|
|
public ProviderSubscriptionUpdate(
|
|
PlanType planType,
|
|
int previouslyPurchasedSeats,
|
|
int newlyPurchasedSeats)
|
|
{
|
|
if (!planType.SupportsConsolidatedBilling())
|
|
{
|
|
throw ContactSupport($"Cannot create a {nameof(ProviderSubscriptionUpdate)} for {nameof(PlanType)} that doesn't support consolidated billing");
|
|
}
|
|
|
|
_planId = GetPasswordManagerPlanId(Utilities.StaticStore.GetPlan(planType));
|
|
_previouslyPurchasedSeats = previouslyPurchasedSeats;
|
|
_newlyPurchasedSeats = newlyPurchasedSeats;
|
|
}
|
|
|
|
public override List<SubscriptionItemOptions> RevertItemsOptions(Subscription subscription)
|
|
{
|
|
var subscriptionItem = FindSubscriptionItem(subscription, _planId);
|
|
|
|
return
|
|
[
|
|
new SubscriptionItemOptions
|
|
{
|
|
Id = subscriptionItem.Id,
|
|
Price = _planId,
|
|
Quantity = _previouslyPurchasedSeats
|
|
}
|
|
];
|
|
}
|
|
|
|
public override List<SubscriptionItemOptions> UpgradeItemsOptions(Subscription subscription)
|
|
{
|
|
var subscriptionItem = FindSubscriptionItem(subscription, _planId);
|
|
|
|
return
|
|
[
|
|
new SubscriptionItemOptions
|
|
{
|
|
Id = subscriptionItem.Id,
|
|
Price = _planId,
|
|
Quantity = _newlyPurchasedSeats
|
|
}
|
|
];
|
|
}
|
|
}
|