mirror of
https://github.com/bitwarden/server.git
synced 2025-04-04 20:50:21 -05:00
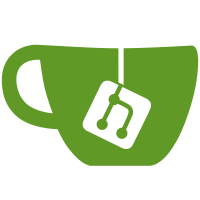
* Implement userkey rotation v2 * Update request models * Cleanup * Update tests * Improve test * Add tests * Fix formatting * Fix test * Remove whitespace * Fix namespace * Enable nullable on models * Fix build * Add tests and enable nullable on masterpasswordunlockdatamodel * Fix test * Remove rollback * Add tests * Make masterpassword hint optional * Update user query * Add EF test * Improve test * Cleanup * Set masterpassword hint * Remove connection close * Add tests for invalid kdf types * Update test/Core.Test/KeyManagement/UserKey/RotateUserAccountKeysCommandTests.cs Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com> * Fix formatting * Update src/Api/KeyManagement/Models/Requests/RotateAccountKeysAndDataRequestModel.cs Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com> * Update src/Api/Auth/Models/Request/Accounts/MasterPasswordUnlockDataModel.cs Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com> * Update src/Api/Auth/Models/Request/Accounts/MasterPasswordUnlockDataModel.cs Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com> * Update src/Api/KeyManagement/Models/Requests/AccountKeysRequestModel.cs Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com> * Fix imports * Fix tests * Add poc for tde rotation * Improve rotation transaction safety * Add validator tests * Clean up validator * Add newline * Add devicekey unlock data to integration test * Fix tests * Fix tests * Remove null check * Remove null check * Fix IsTrusted returning wrong result * Add rollback * Cleanup * Address feedback * Further renames --------- Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com>
146 lines
4.7 KiB
C#
146 lines
4.7 KiB
C#
using System.Data;
|
|
using Bit.Core.Auth.Models.Data;
|
|
using Bit.Core.Entities;
|
|
using Bit.Core.KeyManagement.UserKey;
|
|
using Bit.Core.Repositories;
|
|
using Bit.Core.Settings;
|
|
using Bit.Core.Utilities;
|
|
using Dapper;
|
|
using Microsoft.Data.SqlClient;
|
|
|
|
#nullable enable
|
|
|
|
namespace Bit.Infrastructure.Dapper.Repositories;
|
|
|
|
public class DeviceRepository : Repository<Device, Guid>, IDeviceRepository
|
|
{
|
|
private readonly IGlobalSettings _globalSettings;
|
|
|
|
public DeviceRepository(GlobalSettings globalSettings)
|
|
: this(globalSettings.SqlServer.ConnectionString, globalSettings.SqlServer.ReadOnlyConnectionString)
|
|
{
|
|
_globalSettings = globalSettings;
|
|
}
|
|
|
|
public DeviceRepository(string connectionString, string readOnlyConnectionString)
|
|
: base(connectionString, readOnlyConnectionString)
|
|
{ }
|
|
|
|
public async Task<Device?> GetByIdAsync(Guid id, Guid userId)
|
|
{
|
|
var device = await GetByIdAsync(id);
|
|
if (device == null || device.UserId != userId)
|
|
{
|
|
return null;
|
|
}
|
|
|
|
return device;
|
|
}
|
|
|
|
public async Task<Device?> GetByIdentifierAsync(string identifier)
|
|
{
|
|
using (var connection = new SqlConnection(ConnectionString))
|
|
{
|
|
var results = await connection.QueryAsync<Device>(
|
|
$"[{Schema}].[{Table}_ReadByIdentifier]",
|
|
new
|
|
{
|
|
Identifier = identifier
|
|
},
|
|
commandType: CommandType.StoredProcedure);
|
|
|
|
return results.FirstOrDefault();
|
|
}
|
|
}
|
|
|
|
public async Task<Device?> GetByIdentifierAsync(string identifier, Guid userId)
|
|
{
|
|
using (var connection = new SqlConnection(ConnectionString))
|
|
{
|
|
var results = await connection.QueryAsync<Device>(
|
|
$"[{Schema}].[{Table}_ReadByIdentifierUserId]",
|
|
new
|
|
{
|
|
UserId = userId,
|
|
Identifier = identifier
|
|
},
|
|
commandType: CommandType.StoredProcedure);
|
|
|
|
return results.FirstOrDefault();
|
|
}
|
|
}
|
|
|
|
public async Task<ICollection<Device>> GetManyByUserIdAsync(Guid userId)
|
|
{
|
|
using (var connection = new SqlConnection(ConnectionString))
|
|
{
|
|
var results = await connection.QueryAsync<Device>(
|
|
$"[{Schema}].[{Table}_ReadByUserId]",
|
|
new { UserId = userId },
|
|
commandType: CommandType.StoredProcedure);
|
|
|
|
return results.ToList();
|
|
}
|
|
}
|
|
|
|
public async Task<ICollection<DeviceAuthDetails>> GetManyByUserIdWithDeviceAuth(Guid userId)
|
|
{
|
|
var expirationMinutes = _globalSettings.PasswordlessAuth.UserRequestExpiration.TotalMinutes;
|
|
using (var connection = new SqlConnection(ConnectionString))
|
|
{
|
|
var results = await connection.QueryAsync<DeviceAuthDetails>(
|
|
$"[{Schema}].[{Table}_ReadActiveWithPendingAuthRequestsByUserId]",
|
|
new
|
|
{
|
|
UserId = userId,
|
|
ExpirationMinutes = expirationMinutes
|
|
},
|
|
commandType: CommandType.StoredProcedure);
|
|
|
|
return results.ToList();
|
|
}
|
|
}
|
|
|
|
public async Task ClearPushTokenAsync(Guid id)
|
|
{
|
|
using (var connection = new SqlConnection(ConnectionString))
|
|
{
|
|
await connection.ExecuteAsync(
|
|
$"[{Schema}].[{Table}_ClearPushTokenById]",
|
|
new { Id = id },
|
|
commandType: CommandType.StoredProcedure);
|
|
}
|
|
}
|
|
|
|
public UpdateEncryptedDataForKeyRotation UpdateKeysForRotationAsync(Guid userId, IEnumerable<Device> devices)
|
|
{
|
|
return async (SqlConnection connection, SqlTransaction transaction) =>
|
|
{
|
|
const string sql = @"
|
|
UPDATE D
|
|
SET
|
|
D.[EncryptedPublicKey] = UD.[encryptedPublicKey],
|
|
D.[EncryptedUserKey] = UD.[encryptedUserKey]
|
|
FROM
|
|
[dbo].[Device] D
|
|
INNER JOIN
|
|
OPENJSON(@DeviceCredentials)
|
|
WITH (
|
|
id UNIQUEIDENTIFIER,
|
|
encryptedPublicKey NVARCHAR(MAX),
|
|
encryptedUserKey NVARCHAR(MAX)
|
|
) UD
|
|
ON UD.[id] = D.[Id]
|
|
WHERE
|
|
D.[UserId] = @UserId";
|
|
var deviceCredentials = CoreHelpers.ClassToJsonData(devices);
|
|
|
|
await connection.ExecuteAsync(
|
|
sql,
|
|
new { UserId = userId, DeviceCredentials = deviceCredentials },
|
|
transaction: transaction,
|
|
commandType: CommandType.Text);
|
|
};
|
|
}
|
|
}
|