mirror of
https://github.com/bitwarden/server.git
synced 2025-07-06 02:22:49 -05:00
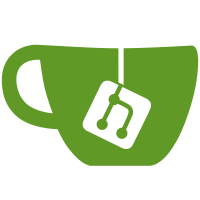
* Upgrade AspNetCoreRateLimiter and enable redis distributed cache for rate limiting. - Upgrades AspNetCoreRateLimiter to 4.0.2, which required updating NewtonSoft.Json to 13.0.1. - Replaces Microsoft.Extensions.Caching.Redis with Microsoft.Extensions.Caching.StackExchangeRedis as the original was deprecated and conflicted with the latest AspNetCoreRateLimiter - Adds startup task to Program.cs for Api/Identity projects to support AspNetCoreRateLimiters breaking changes for seeding its stores. - Adds a Redis connection string option to GlobalSettings Signed-off-by: Shane Melton <smelton@bitwarden.com> * Cleanup Redis distributed cache registration - Add new AddDistributedCache service collection extension to add either a Memory or Redis distributed cache. - Remove distributed cache registration from Identity service collection extension. - Add IpRateLimitSeedStartupService.cs to run at application startup to seed the Ip rate limiting policies. Signed-off-by: Shane Melton <smelton@bitwarden.com> * Add caching configuration to SSO Startup.cs Signed-off-by: Shane Melton <smelton@bitwarden.com> * Add ProjectName as an instance name for Redis options Signed-off-by: Shane Melton <smelton@bitwarden.com> * Use distributed cache in CustomIpRateLimitMiddleware.cs Signed-off-by: Shane Melton <smelton@bitwarden.com> * Undo changes to Program.cs and launchSettings.json * Move new service collection extensions to SharedWeb * Upgrade Caching.StackExchangeRedis package to v6 * Cleanup and fix leftover merge conflicts * Remove use of Newtonsoft.Json in distributed cache extensions * Cleanup more formatting * Fix formatting * Fix startup issue caused by merge and fix integration test Signed-off-by: Shane Melton <smelton@bitwarden.com> * Linting fix Signed-off-by: Shane Melton <smelton@bitwarden.com>
42 lines
1.6 KiB
C#
42 lines
1.6 KiB
C#
using AspNetCoreRateLimit;
|
|
using Microsoft.Extensions.Hosting;
|
|
|
|
namespace Bit.Core.HostedServices
|
|
{
|
|
/// <summary>
|
|
/// A startup service that will seed the IP rate limiting stores with any values in the
|
|
/// GlobalSettings configuration.
|
|
/// </summary>
|
|
/// <remarks>
|
|
/// <para>Using an <see cref="IHostedService"/> here because it runs before the request processing pipeline
|
|
/// is configured, so that any rate limiting configuration is seeded/applied before any requests come in.
|
|
/// </para>
|
|
/// <para>
|
|
/// This is a cleaner alternative to modifying Program.cs in every project that requires rate limiting as
|
|
/// described/suggested here:
|
|
/// https://github.com/stefanprodan/AspNetCoreRateLimit/wiki/Version-3.0.0-Breaking-Changes
|
|
/// </para>
|
|
/// </remarks>
|
|
public class IpRateLimitSeedStartupService : IHostedService
|
|
{
|
|
private readonly IIpPolicyStore _ipPolicyStore;
|
|
private readonly IClientPolicyStore _clientPolicyStore;
|
|
|
|
public IpRateLimitSeedStartupService(IIpPolicyStore ipPolicyStore, IClientPolicyStore clientPolicyStore)
|
|
{
|
|
_ipPolicyStore = ipPolicyStore;
|
|
_clientPolicyStore = clientPolicyStore;
|
|
}
|
|
|
|
public async Task StartAsync(CancellationToken cancellationToken)
|
|
{
|
|
// Seed the policies from GlobalSettings
|
|
await _ipPolicyStore.SeedAsync();
|
|
await _clientPolicyStore.SeedAsync();
|
|
}
|
|
|
|
// noop
|
|
public Task StopAsync(CancellationToken cancellationToken) => Task.CompletedTask;
|
|
}
|
|
}
|