mirror of
https://github.com/bitwarden/server.git
synced 2025-07-05 10:02:47 -05:00
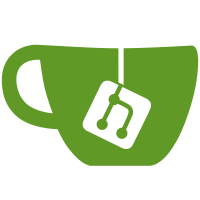
* refactor the plan and create new objects * initial commit * Add new plan types * continue the refactoring by adding new plantypes * changes for plans * Refactoring continues * making changes for plan * Fixing the failing test * Fixing whitespace * Fix some in correct values * Resolve the plan data * rearranging the plan * Make the plan more immutable * Resolve the lint errors * Fix the failing test * Add custom plan * Fix the failing test * Fix the failing test * resolve the failing addons after refactoring * Refactoring * Merge branch 'master' into ac-1451/refactor-staticstore-plans-and-consuming-logic * merge from master * Merge branch 'master' into ac-1451/refactor-staticstore-plans-and-consuming-logic * format whitespace * resolve the conflict * Fix some pr comments * Fixing some of the pr comments * fixing some of the pr comments * Resolve some pr comments * Resolve pr comments * Resolves some pr comments * Resolving some or comments * Resolve a failing test * fix the failing test * Resolving some pr comments * Fix the failing test * resolve pr comment * add a using statement fir a failing test --------- Co-authored-by: Thomas Rittson <trittson@bitwarden.com>
122 lines
4.3 KiB
C#
122 lines
4.3 KiB
C#
using Bit.Core.Entities;
|
|
using Bit.Core.Models.Api;
|
|
using Bit.Core.Models.Business;
|
|
using Bit.Core.Utilities;
|
|
|
|
namespace Bit.Api.Models.Response;
|
|
|
|
public class SubscriptionResponseModel : ResponseModel
|
|
{
|
|
public SubscriptionResponseModel(User user, SubscriptionInfo subscription, UserLicense license)
|
|
: base("subscription")
|
|
{
|
|
Subscription = subscription.Subscription != null ? new BillingSubscription(subscription.Subscription) : null;
|
|
UpcomingInvoice = subscription.UpcomingInvoice != null ?
|
|
new BillingSubscriptionUpcomingInvoice(subscription.UpcomingInvoice) : null;
|
|
Discount = subscription.Discount != null ? new BillingCustomerDiscount(subscription.Discount) : null;
|
|
StorageName = user.Storage.HasValue ? CoreHelpers.ReadableBytesSize(user.Storage.Value) : null;
|
|
StorageGb = user.Storage.HasValue ? Math.Round(user.Storage.Value / 1073741824D, 2) : 0; // 1 GB
|
|
MaxStorageGb = user.MaxStorageGb;
|
|
License = license;
|
|
Expiration = License.Expires;
|
|
UsingInAppPurchase = subscription.UsingInAppPurchase;
|
|
}
|
|
|
|
public SubscriptionResponseModel(User user, UserLicense license = null)
|
|
: base("subscription")
|
|
{
|
|
StorageName = user.Storage.HasValue ? CoreHelpers.ReadableBytesSize(user.Storage.Value) : null;
|
|
StorageGb = user.Storage.HasValue ? Math.Round(user.Storage.Value / 1073741824D, 2) : 0; // 1 GB
|
|
MaxStorageGb = user.MaxStorageGb;
|
|
Expiration = user.PremiumExpirationDate;
|
|
|
|
if (license != null)
|
|
{
|
|
License = license;
|
|
}
|
|
}
|
|
|
|
public string StorageName { get; set; }
|
|
public double? StorageGb { get; set; }
|
|
public short? MaxStorageGb { get; set; }
|
|
public BillingSubscriptionUpcomingInvoice UpcomingInvoice { get; set; }
|
|
public BillingSubscription Subscription { get; set; }
|
|
public BillingCustomerDiscount Discount { get; set; }
|
|
public UserLicense License { get; set; }
|
|
public DateTime? Expiration { get; set; }
|
|
public bool UsingInAppPurchase { get; set; }
|
|
}
|
|
|
|
public class BillingCustomerDiscount
|
|
{
|
|
public BillingCustomerDiscount(SubscriptionInfo.BillingCustomerDiscount discount)
|
|
{
|
|
Id = discount.Id;
|
|
Active = discount.Active;
|
|
}
|
|
|
|
public string Id { get; set; }
|
|
public bool Active { get; set; }
|
|
}
|
|
|
|
public class BillingSubscription
|
|
{
|
|
public BillingSubscription(SubscriptionInfo.BillingSubscription sub)
|
|
{
|
|
Status = sub.Status;
|
|
TrialStartDate = sub.TrialStartDate;
|
|
TrialEndDate = sub.TrialEndDate;
|
|
PeriodStartDate = sub.PeriodStartDate;
|
|
PeriodEndDate = sub.PeriodEndDate;
|
|
CancelledDate = sub.CancelledDate;
|
|
CancelAtEndDate = sub.CancelAtEndDate;
|
|
Cancelled = sub.Cancelled;
|
|
if (sub.Items != null)
|
|
{
|
|
Items = sub.Items.Select(i => new BillingSubscriptionItem(i));
|
|
}
|
|
}
|
|
|
|
public DateTime? TrialStartDate { get; set; }
|
|
public DateTime? TrialEndDate { get; set; }
|
|
public DateTime? PeriodStartDate { get; set; }
|
|
public DateTime? PeriodEndDate { get; set; }
|
|
public DateTime? CancelledDate { get; set; }
|
|
public bool CancelAtEndDate { get; set; }
|
|
public string Status { get; set; }
|
|
public bool Cancelled { get; set; }
|
|
public IEnumerable<BillingSubscriptionItem> Items { get; set; } = new List<BillingSubscriptionItem>();
|
|
|
|
public class BillingSubscriptionItem
|
|
{
|
|
public BillingSubscriptionItem(SubscriptionInfo.BillingSubscription.BillingSubscriptionItem item)
|
|
{
|
|
Name = item.Name;
|
|
Amount = item.Amount;
|
|
Interval = item.Interval;
|
|
Quantity = item.Quantity;
|
|
SponsoredSubscriptionItem = item.SponsoredSubscriptionItem;
|
|
AddonSubscriptionItem = item.AddonSubscriptionItem;
|
|
}
|
|
|
|
public string Name { get; set; }
|
|
public decimal Amount { get; set; }
|
|
public int Quantity { get; set; }
|
|
public string Interval { get; set; }
|
|
public bool SponsoredSubscriptionItem { get; set; }
|
|
public bool AddonSubscriptionItem { get; set; }
|
|
}
|
|
}
|
|
|
|
public class BillingSubscriptionUpcomingInvoice
|
|
{
|
|
public BillingSubscriptionUpcomingInvoice(SubscriptionInfo.BillingUpcomingInvoice inv)
|
|
{
|
|
Amount = inv.Amount;
|
|
Date = inv.Date;
|
|
}
|
|
|
|
public decimal? Amount { get; set; }
|
|
public DateTime? Date { get; set; }
|
|
}
|