mirror of
https://github.com/bitwarden/server.git
synced 2025-06-30 07:36:14 -05:00
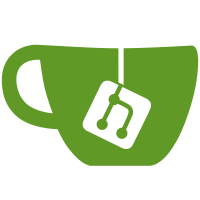
* [EC-634] Extract GenerateLicenseAsync to a query (#2373) * [EC-637] Add license sync to server (#2453) * [EC-1036] Show correct license sync date (#2626) * Update method name per new pattern
52 lines
1.5 KiB
C#
52 lines
1.5 KiB
C#
using System.Text.Json;
|
|
using Bit.Core.Enums;
|
|
using Bit.Core.Exceptions;
|
|
using Bit.Core.Models.Data.Organizations.OrganizationConnections;
|
|
using Bit.Core.Models.OrganizationConnectionConfigs;
|
|
using Bit.Core.Utilities;
|
|
|
|
namespace Bit.Api.Models.Request.Organizations;
|
|
|
|
public class OrganizationConnectionRequestModel
|
|
{
|
|
public OrganizationConnectionType Type { get; set; }
|
|
public Guid OrganizationId { get; set; }
|
|
public bool Enabled { get; set; }
|
|
public JsonDocument Config { get; set; }
|
|
|
|
public OrganizationConnectionRequestModel() { }
|
|
}
|
|
|
|
|
|
public class OrganizationConnectionRequestModel<T> : OrganizationConnectionRequestModel where T : IConnectionConfig
|
|
{
|
|
public T ParsedConfig { get; private set; }
|
|
|
|
public OrganizationConnectionRequestModel(OrganizationConnectionRequestModel model)
|
|
{
|
|
Type = model.Type;
|
|
OrganizationId = model.OrganizationId;
|
|
Enabled = model.Enabled;
|
|
Config = model.Config;
|
|
|
|
try
|
|
{
|
|
ParsedConfig = model.Config.ToObject<T>(JsonHelpers.IgnoreCase);
|
|
}
|
|
catch (JsonException)
|
|
{
|
|
throw new BadRequestException("Organization Connection configuration malformed");
|
|
}
|
|
}
|
|
|
|
public OrganizationConnectionData<T> ToData(Guid? id = null) =>
|
|
new()
|
|
{
|
|
Id = id,
|
|
Type = Type,
|
|
OrganizationId = OrganizationId,
|
|
Enabled = Enabled,
|
|
Config = ParsedConfig,
|
|
};
|
|
}
|