mirror of
https://github.com/bitwarden/server.git
synced 2025-07-14 14:17:35 -05:00
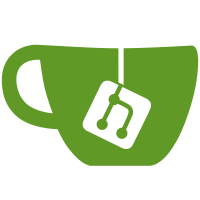
* [EC-390] Added Scim.Test unit tests project * [EC-390] Added ConflictException type. Updated BadRequestException to have parameterless constructor. Updated NotFoundException to have constructor with a message parameter * [EC-534] Implemented CQRS for Users Get and added unit tests * [EC-508] Renamed GetUserCommand to GetUserQuery * [EC-508] Created ScimServiceCollectionExtensions * [EC-508] Renamed AddScimCommands to AddScimUserQueries * [EC-508] Created ExceptionHandlerFilterAttribute on SCIM project Co-authored-by: Thomas Rittson <31796059+eliykat@users.noreply.github.com>
67 lines
2.8 KiB
C#
67 lines
2.8 KiB
C#
using Bit.Core.Entities;
|
|
using Bit.Core.Exceptions;
|
|
using Bit.Core.Models.Data.Organizations.OrganizationUsers;
|
|
using Bit.Core.Repositories;
|
|
using Bit.Scim.Queries.Users;
|
|
using Bit.Scim.Utilities;
|
|
using Bit.Test.Common.AutoFixture;
|
|
using Bit.Test.Common.AutoFixture.Attributes;
|
|
using Bit.Test.Common.Helpers;
|
|
using NSubstitute;
|
|
using Xunit;
|
|
|
|
namespace Bit.Scim.Test.Queries.Users;
|
|
|
|
[SutProviderCustomize]
|
|
public class GetUserQueryTests
|
|
{
|
|
[Theory]
|
|
[BitAutoData]
|
|
public async Task GetUser_Success(SutProvider<GetUserQuery> sutProvider, OrganizationUserUserDetails organizationUserUserDetails)
|
|
{
|
|
var expectedResult = new Models.ScimUserResponseModel
|
|
{
|
|
Id = organizationUserUserDetails.Id.ToString(),
|
|
UserName = organizationUserUserDetails.Email,
|
|
Name = new Models.BaseScimUserModel.NameModel(organizationUserUserDetails.Name),
|
|
Emails = new List<Models.BaseScimUserModel.EmailModel> { new Models.BaseScimUserModel.EmailModel(organizationUserUserDetails.Email) },
|
|
DisplayName = organizationUserUserDetails.Name,
|
|
Active = organizationUserUserDetails.Status != Core.Enums.OrganizationUserStatusType.Revoked ? true : false,
|
|
Groups = new List<string>(),
|
|
ExternalId = organizationUserUserDetails.ExternalId,
|
|
Schemas = new List<string> { ScimConstants.Scim2SchemaUser }
|
|
};
|
|
|
|
sutProvider.GetDependency<IOrganizationUserRepository>()
|
|
.GetDetailsByIdAsync(organizationUserUserDetails.Id)
|
|
.Returns(organizationUserUserDetails);
|
|
|
|
var result = await sutProvider.Sut.GetUserAsync(organizationUserUserDetails.OrganizationId, organizationUserUserDetails.Id);
|
|
|
|
await sutProvider.GetDependency<IOrganizationUserRepository>().Received(1).GetDetailsByIdAsync(organizationUserUserDetails.Id);
|
|
AssertHelper.AssertPropertyEqual(expectedResult, result);
|
|
}
|
|
|
|
[Theory]
|
|
[BitAutoData]
|
|
public async Task GetUser_NotFound_Throws(SutProvider<GetUserQuery> sutProvider, Guid organizationId, Guid organizationUserId)
|
|
{
|
|
await Assert.ThrowsAsync<NotFoundException>(async () => await sutProvider.Sut.GetUserAsync(organizationId, organizationUserId));
|
|
}
|
|
|
|
[Theory]
|
|
[BitAutoData]
|
|
public async Task GetUser_MismatchingOrganizationId_Throws(SutProvider<GetUserQuery> sutProvider, Guid organizationId, Guid organizationUserId)
|
|
{
|
|
sutProvider.GetDependency<IOrganizationUserRepository>()
|
|
.GetByIdAsync(organizationUserId)
|
|
.Returns(new OrganizationUser
|
|
{
|
|
Id = organizationUserId,
|
|
OrganizationId = Guid.NewGuid()
|
|
});
|
|
|
|
await Assert.ThrowsAsync<NotFoundException>(async () => await sutProvider.Sut.GetUserAsync(organizationId, organizationUserId));
|
|
}
|
|
}
|