mirror of
https://github.com/bitwarden/server.git
synced 2025-04-14 01:28:14 -05:00
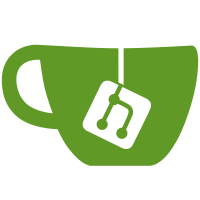
* [PM-17563] Add case for PushType.PendingSecurityTasks * [PM-17563] Add missing TaskId property to NotificationStatusDetails and NotificationResponseModel * [PM-17563] Add migration script to re-create NotificationStatusDetailsView to include TaskId column * [PM-17563] Select explicit columns for NotificationStatusDetailsView and fix migration script
50 lines
1.4 KiB
C#
50 lines
1.4 KiB
C#
#nullable enable
|
|
using Bit.Core.Models.Api;
|
|
using Bit.Core.NotificationCenter.Enums;
|
|
using Bit.Core.NotificationCenter.Models.Data;
|
|
|
|
namespace Bit.Api.NotificationCenter.Models.Response;
|
|
|
|
public class NotificationResponseModel : ResponseModel
|
|
{
|
|
private const string _objectName = "notification";
|
|
|
|
public NotificationResponseModel(NotificationStatusDetails notificationStatusDetails, string obj = _objectName)
|
|
: base(obj)
|
|
{
|
|
if (notificationStatusDetails == null)
|
|
{
|
|
throw new ArgumentNullException(nameof(notificationStatusDetails));
|
|
}
|
|
|
|
Id = notificationStatusDetails.Id;
|
|
Priority = notificationStatusDetails.Priority;
|
|
Title = notificationStatusDetails.Title;
|
|
Body = notificationStatusDetails.Body;
|
|
Date = notificationStatusDetails.RevisionDate;
|
|
TaskId = notificationStatusDetails.TaskId;
|
|
ReadDate = notificationStatusDetails.ReadDate;
|
|
DeletedDate = notificationStatusDetails.DeletedDate;
|
|
}
|
|
|
|
public NotificationResponseModel() : base(_objectName)
|
|
{
|
|
}
|
|
|
|
public Guid Id { get; set; }
|
|
|
|
public Priority Priority { get; set; }
|
|
|
|
public string? Title { get; set; }
|
|
|
|
public string? Body { get; set; }
|
|
|
|
public DateTime Date { get; set; }
|
|
|
|
public Guid? TaskId { get; set; }
|
|
|
|
public DateTime? ReadDate { get; set; }
|
|
|
|
public DateTime? DeletedDate { get; set; }
|
|
}
|