mirror of
https://github.com/bitwarden/server.git
synced 2025-04-05 05:00:19 -05:00
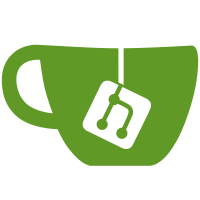
* Upgrade to Duende.Identity * Linting * Get rid of last IdentityServer4 package * Fix identity test since Duende returns additional configuration * Use Configure PostConfigure is ran after ASP.NET's PostConfigure so ConfigurationManager was already configured and our HttpHandler wasn't being respected. * Regenerate lockfiles * Move to 6.0.4 for patches * fixes with testing * Add additional grant type supported in 6.0.4 and beautify * Lockfile refresh * Reapply lockfiles * Apply change to new WebAuthn logic * When automated merging fails me --------- Co-authored-by: Justin Baur <19896123+justindbaur@users.noreply.github.com> Co-authored-by: Kyle Spearrin <kyle.spearrin@gmail.com>
78 lines
2.8 KiB
C#
78 lines
2.8 KiB
C#
using Bit.Core.Settings;
|
|
using Duende.IdentityServer.Models;
|
|
|
|
namespace Bit.Identity.IdentityServer;
|
|
|
|
public class ApiClient : Client
|
|
{
|
|
public ApiClient(
|
|
GlobalSettings globalSettings,
|
|
string id,
|
|
int refreshTokenSlidingDays,
|
|
int accessTokenLifetimeHours,
|
|
string[] scopes = null)
|
|
{
|
|
ClientId = id;
|
|
AllowedGrantTypes = new[] { GrantType.ResourceOwnerPassword, GrantType.AuthorizationCode, WebAuthnGrantValidator.GrantType };
|
|
RefreshTokenExpiration = TokenExpiration.Sliding;
|
|
RefreshTokenUsage = TokenUsage.ReUse;
|
|
SlidingRefreshTokenLifetime = 86400 * refreshTokenSlidingDays;
|
|
AbsoluteRefreshTokenLifetime = 0; // forever
|
|
UpdateAccessTokenClaimsOnRefresh = true;
|
|
AccessTokenLifetime = 3600 * accessTokenLifetimeHours;
|
|
AllowOfflineAccess = true;
|
|
|
|
RequireConsent = false;
|
|
RequirePkce = true;
|
|
RequireClientSecret = false;
|
|
if (id == "web")
|
|
{
|
|
RedirectUris = new[] { $"{globalSettings.BaseServiceUri.Vault}/sso-connector.html" };
|
|
PostLogoutRedirectUris = new[] { globalSettings.BaseServiceUri.Vault };
|
|
AllowedCorsOrigins = new[] { globalSettings.BaseServiceUri.Vault };
|
|
}
|
|
else if (id == "desktop")
|
|
{
|
|
RedirectUris = new[] { "bitwarden://sso-callback" };
|
|
PostLogoutRedirectUris = new[] { "bitwarden://logged-out" };
|
|
}
|
|
else if (id == "connector")
|
|
{
|
|
var connectorUris = new List<string>();
|
|
for (var port = 8065; port <= 8070; port++)
|
|
{
|
|
connectorUris.Add(string.Format("http://localhost:{0}", port));
|
|
}
|
|
RedirectUris = connectorUris.Append("bwdc://sso-callback").ToList();
|
|
PostLogoutRedirectUris = connectorUris.Append("bwdc://logged-out").ToList();
|
|
}
|
|
else if (id == "browser")
|
|
{
|
|
RedirectUris = new[] { $"{globalSettings.BaseServiceUri.Vault}/sso-connector.html" };
|
|
PostLogoutRedirectUris = new[] { globalSettings.BaseServiceUri.Vault };
|
|
AllowedCorsOrigins = new[] { globalSettings.BaseServiceUri.Vault };
|
|
}
|
|
else if (id == "cli")
|
|
{
|
|
var cliUris = new List<string>();
|
|
for (var port = 8065; port <= 8070; port++)
|
|
{
|
|
cliUris.Add(string.Format("http://localhost:{0}", port));
|
|
}
|
|
RedirectUris = cliUris;
|
|
PostLogoutRedirectUris = cliUris;
|
|
}
|
|
else if (id == "mobile")
|
|
{
|
|
RedirectUris = new[] { "bitwarden://sso-callback" };
|
|
PostLogoutRedirectUris = new[] { "bitwarden://logged-out" };
|
|
}
|
|
|
|
if (scopes == null)
|
|
{
|
|
scopes = new string[] { "api" };
|
|
}
|
|
AllowedScopes = scopes;
|
|
}
|
|
}
|