mirror of
https://github.com/bitwarden/server.git
synced 2025-04-05 05:00:19 -05:00
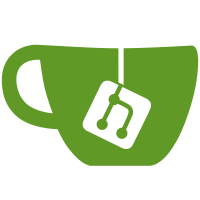
* [PM-1188] add sso project to auth * [PM-1188] move sso api models to auth * [PM-1188] fix sso api model namespace & imports * [PM-1188] move core files to auth * [PM-1188] fix core sso namespace & models * [PM-1188] move sso repository files to auth * [PM-1188] fix sso repo files namespace & imports * [PM-1188] move sso sql files to auth folder * [PM-1188] move sso test files to auth folders * [PM-1188] fix sso tests namespace & imports * [PM-1188] move auth api files to auth folder * [PM-1188] fix auth api files namespace & imports * [PM-1188] move auth core files to auth folder * [PM-1188] fix auth core files namespace & imports * [PM-1188] move auth email templates to auth folder * [PM-1188] move auth email folder back into shared directory * [PM-1188] fix auth email names * [PM-1188] move auth core models to auth folder * [PM-1188] fix auth model namespace & imports * [PM-1188] add entire Identity project to auth codeowners * [PM-1188] fix auth orm files namespace & imports * [PM-1188] move auth orm files to auth folder * [PM-1188] move auth sql files to auth folder * [PM-1188] move auth tests to auth folder * [PM-1188] fix auth test files namespace & imports * [PM-1188] move emergency access api files to auth folder * [PM-1188] fix emergencyaccess api files namespace & imports * [PM-1188] move emergency access core files to auth folder * [PM-1188] fix emergency access core files namespace & imports * [PM-1188] move emergency access orm files to auth folder * [PM-1188] fix emergency access orm files namespace & imports * [PM-1188] move emergency access sql files to auth folder * [PM-1188] move emergencyaccess test files to auth folder * [PM-1188] fix emergency access test files namespace & imports * [PM-1188] move captcha files to auth folder * [PM-1188] fix captcha files namespace & imports * [PM-1188] move auth admin files into auth folder * [PM-1188] fix admin auth files namespace & imports - configure mvc to look in auth folders for views * [PM-1188] remove extra imports and formatting * [PM-1188] fix ef auth model imports * [PM-1188] fix DatabaseContextModelSnapshot paths * [PM-1188] fix grant import in ef * [PM-1188] update sqlproj * [PM-1188] move missed sqlproj files * [PM-1188] move auth ef models out of auth folder * [PM-1188] fix auth ef models namespace * [PM-1188] remove auth ef models unused imports * [PM-1188] fix imports for auth ef models * [PM-1188] fix more ef model imports * [PM-1188] fix file encodings
93 lines
2.4 KiB
C#
93 lines
2.4 KiB
C#
using Bit.Admin.Auth.IdentityServer;
|
|
using Bit.Admin.Auth.Models;
|
|
using Microsoft.AspNetCore.Identity;
|
|
using Microsoft.AspNetCore.Mvc;
|
|
|
|
namespace Bit.Admin.Auth.Controllers;
|
|
|
|
public class LoginController : Controller
|
|
{
|
|
private readonly PasswordlessSignInManager<IdentityUser> _signInManager;
|
|
|
|
public LoginController(
|
|
PasswordlessSignInManager<IdentityUser> signInManager)
|
|
{
|
|
_signInManager = signInManager;
|
|
}
|
|
|
|
public IActionResult Index(string returnUrl = null, int? error = null, int? success = null,
|
|
bool accessDenied = false)
|
|
{
|
|
if (!error.HasValue && accessDenied)
|
|
{
|
|
error = 4;
|
|
}
|
|
|
|
return View(new LoginModel
|
|
{
|
|
ReturnUrl = returnUrl,
|
|
Error = GetMessage(error),
|
|
Success = GetMessage(success)
|
|
});
|
|
}
|
|
|
|
[HttpPost]
|
|
[ValidateAntiForgeryToken]
|
|
public async Task<IActionResult> Index(LoginModel model)
|
|
{
|
|
if (ModelState.IsValid)
|
|
{
|
|
await _signInManager.PasswordlessSignInAsync(model.Email, model.ReturnUrl);
|
|
return RedirectToAction("Index", new
|
|
{
|
|
success = 3
|
|
});
|
|
}
|
|
|
|
return View(model);
|
|
}
|
|
|
|
public async Task<IActionResult> Confirm(string email, string token, string returnUrl)
|
|
{
|
|
var result = await _signInManager.PasswordlessSignInAsync(email, token, true);
|
|
if (!result.Succeeded)
|
|
{
|
|
return RedirectToAction("Index", new
|
|
{
|
|
error = 2
|
|
});
|
|
}
|
|
|
|
if (!string.IsNullOrWhiteSpace(returnUrl) && Url.IsLocalUrl(returnUrl))
|
|
{
|
|
return Redirect(returnUrl);
|
|
}
|
|
|
|
return RedirectToAction("Index", "Home");
|
|
}
|
|
|
|
[HttpPost]
|
|
[ValidateAntiForgeryToken]
|
|
public async Task<IActionResult> Logout()
|
|
{
|
|
await _signInManager.SignOutAsync();
|
|
return RedirectToAction("Index", new
|
|
{
|
|
success = 1
|
|
});
|
|
}
|
|
|
|
private string GetMessage(int? messageCode)
|
|
{
|
|
return messageCode switch
|
|
{
|
|
1 => "You have been logged out.",
|
|
2 => "This login confirmation link is invalid. Try logging in again.",
|
|
3 => "If a valid admin user with this email address exists, " +
|
|
"we've sent you an email with a secure link to log in.",
|
|
4 => "Access denied. Please log in.",
|
|
_ => null,
|
|
};
|
|
}
|
|
}
|