mirror of
https://github.com/bitwarden/server.git
synced 2025-07-05 10:02:47 -05:00
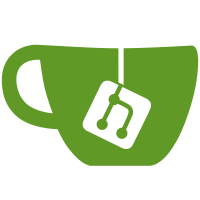
* [PM-1188] add sso project to auth * [PM-1188] move sso api models to auth * [PM-1188] fix sso api model namespace & imports * [PM-1188] move core files to auth * [PM-1188] fix core sso namespace & models * [PM-1188] move sso repository files to auth * [PM-1188] fix sso repo files namespace & imports * [PM-1188] move sso sql files to auth folder * [PM-1188] move sso test files to auth folders * [PM-1188] fix sso tests namespace & imports * [PM-1188] move auth api files to auth folder * [PM-1188] fix auth api files namespace & imports * [PM-1188] move auth core files to auth folder * [PM-1188] fix auth core files namespace & imports * [PM-1188] move auth email templates to auth folder * [PM-1188] move auth email folder back into shared directory * [PM-1188] fix auth email names * [PM-1188] move auth core models to auth folder * [PM-1188] fix auth model namespace & imports * [PM-1188] add entire Identity project to auth codeowners * [PM-1188] fix auth orm files namespace & imports * [PM-1188] move auth orm files to auth folder * [PM-1188] move auth sql files to auth folder * [PM-1188] move auth tests to auth folder * [PM-1188] fix auth test files namespace & imports * [PM-1188] move emergency access api files to auth folder * [PM-1188] fix emergencyaccess api files namespace & imports * [PM-1188] move emergency access core files to auth folder * [PM-1188] fix emergency access core files namespace & imports * [PM-1188] move emergency access orm files to auth folder * [PM-1188] fix emergency access orm files namespace & imports * [PM-1188] move emergency access sql files to auth folder * [PM-1188] move emergencyaccess test files to auth folder * [PM-1188] fix emergency access test files namespace & imports * [PM-1188] move captcha files to auth folder * [PM-1188] fix captcha files namespace & imports * [PM-1188] move auth admin files into auth folder * [PM-1188] fix admin auth files namespace & imports - configure mvc to look in auth folders for views * [PM-1188] remove extra imports and formatting * [PM-1188] fix ef auth model imports * [PM-1188] fix DatabaseContextModelSnapshot paths * [PM-1188] fix grant import in ef * [PM-1188] update sqlproj * [PM-1188] move missed sqlproj files * [PM-1188] move auth ef models out of auth folder * [PM-1188] fix auth ef models namespace * [PM-1188] remove auth ef models unused imports * [PM-1188] fix imports for auth ef models * [PM-1188] fix more ef model imports * [PM-1188] fix file encodings
114 lines
5.3 KiB
C#
114 lines
5.3 KiB
C#
using Bit.Core.Auth.Models.Data;
|
|
using Bit.Core.Enums;
|
|
using Bit.Core.Enums.Provider;
|
|
using Bit.Core.Models.Api;
|
|
using Bit.Core.Models.Data;
|
|
using Bit.Core.Models.Data.Organizations.OrganizationUsers;
|
|
using Bit.Core.Utilities;
|
|
|
|
namespace Bit.Api.Models.Response;
|
|
|
|
public class ProfileOrganizationResponseModel : ResponseModel
|
|
{
|
|
public ProfileOrganizationResponseModel(string str) : base(str) { }
|
|
|
|
public ProfileOrganizationResponseModel(OrganizationUserOrganizationDetails organization) : this("profileOrganization")
|
|
{
|
|
Id = organization.OrganizationId.ToString();
|
|
Name = organization.Name;
|
|
UsePolicies = organization.UsePolicies;
|
|
UseSso = organization.UseSso;
|
|
UseKeyConnector = organization.UseKeyConnector;
|
|
UseScim = organization.UseScim;
|
|
UseGroups = organization.UseGroups;
|
|
UseDirectory = organization.UseDirectory;
|
|
UseEvents = organization.UseEvents;
|
|
UseTotp = organization.UseTotp;
|
|
Use2fa = organization.Use2fa;
|
|
UseApi = organization.UseApi;
|
|
UseResetPassword = organization.UseResetPassword;
|
|
UseSecretsManager = organization.UseSecretsManager;
|
|
UsersGetPremium = organization.UsersGetPremium;
|
|
UseCustomPermissions = organization.UseCustomPermissions;
|
|
UseActivateAutofillPolicy = organization.PlanType == PlanType.EnterpriseAnnually ||
|
|
organization.PlanType == PlanType.EnterpriseMonthly;
|
|
SelfHost = organization.SelfHost;
|
|
Seats = organization.Seats;
|
|
MaxCollections = organization.MaxCollections;
|
|
MaxStorageGb = organization.MaxStorageGb;
|
|
Key = organization.Key;
|
|
HasPublicAndPrivateKeys = organization.PublicKey != null && organization.PrivateKey != null;
|
|
Status = organization.Status;
|
|
Type = organization.Type;
|
|
Enabled = organization.Enabled;
|
|
SsoBound = !string.IsNullOrWhiteSpace(organization.SsoExternalId);
|
|
Identifier = organization.Identifier;
|
|
Permissions = CoreHelpers.LoadClassFromJsonData<Permissions>(organization.Permissions);
|
|
ResetPasswordEnrolled = organization.ResetPasswordKey != null;
|
|
UserId = organization.UserId?.ToString();
|
|
ProviderId = organization.ProviderId?.ToString();
|
|
ProviderName = organization.ProviderName;
|
|
ProviderType = organization.ProviderType;
|
|
FamilySponsorshipFriendlyName = organization.FamilySponsorshipFriendlyName;
|
|
FamilySponsorshipAvailable = FamilySponsorshipFriendlyName == null &&
|
|
StaticStore.GetSponsoredPlan(PlanSponsorshipType.FamiliesForEnterprise)
|
|
.UsersCanSponsor(organization);
|
|
PlanProductType = StaticStore.GetPlan(organization.PlanType).Product;
|
|
FamilySponsorshipLastSyncDate = organization.FamilySponsorshipLastSyncDate;
|
|
FamilySponsorshipToDelete = organization.FamilySponsorshipToDelete;
|
|
FamilySponsorshipValidUntil = organization.FamilySponsorshipValidUntil;
|
|
AccessSecretsManager = organization.AccessSecretsManager;
|
|
|
|
if (organization.SsoConfig != null)
|
|
{
|
|
var ssoConfigData = SsoConfigurationData.Deserialize(organization.SsoConfig);
|
|
KeyConnectorEnabled = ssoConfigData.KeyConnectorEnabled && !string.IsNullOrEmpty(ssoConfigData.KeyConnectorUrl);
|
|
KeyConnectorUrl = ssoConfigData.KeyConnectorUrl;
|
|
}
|
|
}
|
|
|
|
public string Id { get; set; }
|
|
public string Name { get; set; }
|
|
public bool UsePolicies { get; set; }
|
|
public bool UseSso { get; set; }
|
|
public bool UseKeyConnector { get; set; }
|
|
public bool UseScim { get; set; }
|
|
public bool UseGroups { get; set; }
|
|
public bool UseDirectory { get; set; }
|
|
public bool UseEvents { get; set; }
|
|
public bool UseTotp { get; set; }
|
|
public bool Use2fa { get; set; }
|
|
public bool UseApi { get; set; }
|
|
public bool UseResetPassword { get; set; }
|
|
public bool UseSecretsManager { get; set; }
|
|
public bool UsersGetPremium { get; set; }
|
|
public bool UseCustomPermissions { get; set; }
|
|
public bool UseActivateAutofillPolicy { get; set; }
|
|
public bool SelfHost { get; set; }
|
|
public int? Seats { get; set; }
|
|
public short? MaxCollections { get; set; }
|
|
public short? MaxStorageGb { get; set; }
|
|
public string Key { get; set; }
|
|
public OrganizationUserStatusType Status { get; set; }
|
|
public OrganizationUserType Type { get; set; }
|
|
public bool Enabled { get; set; }
|
|
public bool SsoBound { get; set; }
|
|
public string Identifier { get; set; }
|
|
public Permissions Permissions { get; set; }
|
|
public bool ResetPasswordEnrolled { get; set; }
|
|
public string UserId { get; set; }
|
|
public bool HasPublicAndPrivateKeys { get; set; }
|
|
public string ProviderId { get; set; }
|
|
public string ProviderName { get; set; }
|
|
public ProviderType? ProviderType { get; set; }
|
|
public string FamilySponsorshipFriendlyName { get; set; }
|
|
public bool FamilySponsorshipAvailable { get; set; }
|
|
public ProductType PlanProductType { get; set; }
|
|
public bool KeyConnectorEnabled { get; set; }
|
|
public string KeyConnectorUrl { get; set; }
|
|
public DateTime? FamilySponsorshipLastSyncDate { get; set; }
|
|
public DateTime? FamilySponsorshipValidUntil { get; set; }
|
|
public bool? FamilySponsorshipToDelete { get; set; }
|
|
public bool AccessSecretsManager { get; set; }
|
|
}
|