mirror of
https://github.com/bitwarden/server.git
synced 2025-07-14 14:17:35 -05:00
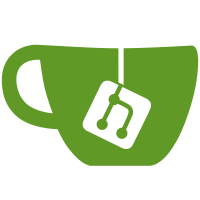
* Allow for binning of comb IDs by date and value * Introduce notification hub pool * Replace device type sharding with comb + range sharding * Fix proxy interface * Use enumerable services for multiServiceNotificationHub * Fix push interface usage * Fix push notification service dependencies * Fix push notification keys * Fixup documentation * Remove deprecated settings * Fix tests * PascalCase method names * Remove unused request model properties * Remove unused setting * Improve DateFromComb precision * Prefer readonly service enumerable * Pascal case template holes * Name TryParse methods TryParse * Apply suggestions from code review Co-authored-by: Justin Baur <19896123+justindbaur@users.noreply.github.com> * Include preferred push technology in config response SignalR will be the fallback, but clients should attempt web push first if offered and available to the client. * Register web push devices * Working signing and content encrypting * update to RFC-8291 and RFC-8188 * Notification hub is now working, no need to create our own * Fix body * Flip Success Check * use nifty json attribute * Remove vapid private key This is only needed to encrypt data for transmission along webpush -- it's handled by NotificationHub for us * Add web push feature flag to control config response * Update src/Core/NotificationHub/NotificationHubConnection.cs Co-authored-by: Justin Baur <19896123+justindbaur@users.noreply.github.com> * Update src/Core/NotificationHub/NotificationHubConnection.cs Co-authored-by: Justin Baur <19896123+justindbaur@users.noreply.github.com> * fixup! Update src/Core/NotificationHub/NotificationHubConnection.cs * Move to platform ownership * Remove debugging extension * Remove unused dependencies * Set json content directly * Name web push registration data * Fix FCM type typo * Determine specific feature flag from set of flags * Fixup merged tests * Fixup tests * Code quality suggestions * Fix merged tests * Fix test --------- Co-authored-by: Justin Baur <19896123+justindbaur@users.noreply.github.com>
92 lines
3.1 KiB
C#
92 lines
3.1 KiB
C#
using Bit.Core;
|
|
using Bit.Core.Enums;
|
|
using Bit.Core.Models.Api;
|
|
using Bit.Core.Services;
|
|
using Bit.Core.Settings;
|
|
using Bit.Core.Utilities;
|
|
|
|
namespace Bit.Api.Models.Response;
|
|
|
|
public class ConfigResponseModel : ResponseModel
|
|
{
|
|
public string Version { get; set; }
|
|
public string GitHash { get; set; }
|
|
public ServerConfigResponseModel Server { get; set; }
|
|
public EnvironmentConfigResponseModel Environment { get; set; }
|
|
public IDictionary<string, object> FeatureStates { get; set; }
|
|
public PushSettings Push { get; set; }
|
|
public ServerSettingsResponseModel Settings { get; set; }
|
|
|
|
public ConfigResponseModel() : base("config")
|
|
{
|
|
Version = AssemblyHelpers.GetVersion();
|
|
GitHash = AssemblyHelpers.GetGitHash();
|
|
Environment = new EnvironmentConfigResponseModel();
|
|
FeatureStates = new Dictionary<string, object>();
|
|
Settings = new ServerSettingsResponseModel();
|
|
}
|
|
|
|
public ConfigResponseModel(
|
|
IFeatureService featureService,
|
|
IGlobalSettings globalSettings
|
|
) : base("config")
|
|
{
|
|
Version = AssemblyHelpers.GetVersion();
|
|
GitHash = AssemblyHelpers.GetGitHash();
|
|
Environment = new EnvironmentConfigResponseModel
|
|
{
|
|
CloudRegion = globalSettings.BaseServiceUri.CloudRegion,
|
|
Vault = globalSettings.BaseServiceUri.Vault,
|
|
Api = globalSettings.BaseServiceUri.Api,
|
|
Identity = globalSettings.BaseServiceUri.Identity,
|
|
Notifications = globalSettings.BaseServiceUri.Notifications,
|
|
Sso = globalSettings.BaseServiceUri.Sso
|
|
};
|
|
FeatureStates = featureService.GetAll();
|
|
var webPushEnabled = FeatureStates.TryGetValue(FeatureFlagKeys.WebPush, out var webPushEnabledValue) ? (bool)webPushEnabledValue : false;
|
|
Push = PushSettings.Build(webPushEnabled, globalSettings);
|
|
Settings = new ServerSettingsResponseModel
|
|
{
|
|
DisableUserRegistration = globalSettings.DisableUserRegistration
|
|
};
|
|
}
|
|
}
|
|
|
|
public class ServerConfigResponseModel
|
|
{
|
|
public string Name { get; set; }
|
|
public string Url { get; set; }
|
|
}
|
|
|
|
public class EnvironmentConfigResponseModel
|
|
{
|
|
public string CloudRegion { get; set; }
|
|
public string Vault { get; set; }
|
|
public string Api { get; set; }
|
|
public string Identity { get; set; }
|
|
public string Notifications { get; set; }
|
|
public string Sso { get; set; }
|
|
}
|
|
|
|
public class PushSettings
|
|
{
|
|
public PushTechnologyType PushTechnology { get; private init; }
|
|
public string VapidPublicKey { get; private init; }
|
|
|
|
public static PushSettings Build(bool webPushEnabled, IGlobalSettings globalSettings)
|
|
{
|
|
var vapidPublicKey = webPushEnabled ? globalSettings.WebPush.VapidPublicKey : null;
|
|
var pushTechnology = vapidPublicKey != null ? PushTechnologyType.WebPush : PushTechnologyType.SignalR;
|
|
return new()
|
|
{
|
|
VapidPublicKey = vapidPublicKey,
|
|
PushTechnology = pushTechnology
|
|
};
|
|
}
|
|
}
|
|
|
|
public class ServerSettingsResponseModel
|
|
{
|
|
public bool DisableUserRegistration { get; set; }
|
|
}
|