mirror of
https://github.com/bitwarden/server.git
synced 2025-07-12 21:27:35 -05:00
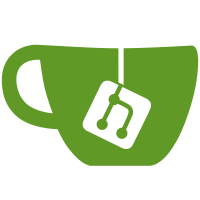
* Add PremiumUserSale * Add PremiumUserBillingService * Integrate into UserService behind FF * Update invoice.created handler to bill newly created PayPal customers * Run dotnet format
50 lines
1.2 KiB
C#
50 lines
1.2 KiB
C#
using Bit.Core.Entities;
|
|
using Bit.Core.Enums;
|
|
using Bit.Core.Models.Business;
|
|
|
|
namespace Bit.Core.Billing.Models.Sales;
|
|
|
|
#nullable enable
|
|
|
|
public class PremiumUserSale
|
|
{
|
|
private PremiumUserSale() { }
|
|
|
|
public required User User { get; set; }
|
|
public required CustomerSetup CustomerSetup { get; set; }
|
|
public short? Storage { get; set; }
|
|
|
|
public void Deconstruct(
|
|
out User user,
|
|
out CustomerSetup customerSetup,
|
|
out short? storage)
|
|
{
|
|
user = User;
|
|
customerSetup = CustomerSetup;
|
|
storage = Storage;
|
|
}
|
|
|
|
public static PremiumUserSale From(
|
|
User user,
|
|
PaymentMethodType paymentMethodType,
|
|
string paymentMethodToken,
|
|
TaxInfo taxInfo,
|
|
short? storage)
|
|
{
|
|
var tokenizedPaymentSource = new TokenizedPaymentSource(paymentMethodType, paymentMethodToken);
|
|
|
|
var taxInformation = TaxInformation.From(taxInfo);
|
|
|
|
return new PremiumUserSale
|
|
{
|
|
User = user,
|
|
CustomerSetup = new CustomerSetup
|
|
{
|
|
TokenizedPaymentSource = tokenizedPaymentSource,
|
|
TaxInformation = taxInformation
|
|
},
|
|
Storage = storage
|
|
};
|
|
}
|
|
}
|