mirror of
https://github.com/bitwarden/server.git
synced 2025-04-16 10:38:17 -05:00
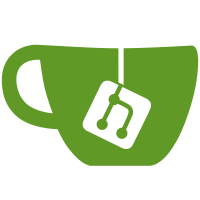
* Add new logic for validating encrypted strings * Add benchmarks * Formatting & Comments * Move Debug assertion to just be a test * Address PR feedback pt.1 * Address more PR feedback * Formatting * merge branch 'master' into 'encrypted-string-perf' * Revert "merge branch 'master' into 'encrypted-string-perf'" This reverts commit a20e127c9c8ba2563949a80218b3f787f0260a4b.
39 lines
939 B
C#
39 lines
939 B
C#
namespace Bit.Core.Utilities;
|
|
|
|
public static class SpanExtensions
|
|
{
|
|
public static bool TrySplitBy(this ReadOnlySpan<char> input,
|
|
char splitChar, out ReadOnlySpan<char> chunk, out ReadOnlySpan<char> rest)
|
|
{
|
|
var splitIndex = input.IndexOf(splitChar);
|
|
|
|
if (splitIndex == -1)
|
|
{
|
|
chunk = default;
|
|
rest = input;
|
|
return false;
|
|
}
|
|
|
|
chunk = input[..splitIndex];
|
|
rest = input[++splitIndex..];
|
|
return true;
|
|
}
|
|
|
|
// Replace with the implementation from .NET 8 when we upgrade
|
|
// Ref: https://github.com/dotnet/runtime/issues/59466
|
|
public static int Count<T>(this ReadOnlySpan<T> span, T value)
|
|
where T : IEquatable<T>
|
|
{
|
|
var count = 0;
|
|
int pos;
|
|
|
|
while ((pos = span.IndexOf(value)) >= 0)
|
|
{
|
|
span = span[++pos..];
|
|
count++;
|
|
}
|
|
|
|
return count;
|
|
}
|
|
}
|