mirror of
https://github.com/bitwarden/server.git
synced 2025-04-04 20:50:21 -05:00
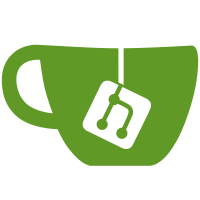
* Added CQRS pattern * Added the GetManyByUserIdAsync signature to the repositiory * Added sql sproc Created user defined type to hold status Created migration file * Added ef core query * Added absract and concrete implementation for GetManyByUserIdStatusAsync * Added integration tests * Updated params to status * Implemented new query to utilize repository method * Added controller for the security task endpoint * Fixed lint issues * Added documentation * simplified to require single status modified script to check for users with edit rights * Updated ef core query * Added new assertions * simplified to require single status * fixed formatting * Fixed sql script * Removed default null * Added security tasks feature flag
29 lines
1.2 KiB
C#
29 lines
1.2 KiB
C#
using AutoMapper;
|
|
using Bit.Core.Vault.Enums;
|
|
using Bit.Core.Vault.Repositories;
|
|
using Bit.Infrastructure.EntityFramework.Repositories;
|
|
using Bit.Infrastructure.EntityFramework.Vault.Models;
|
|
using Bit.Infrastructure.EntityFramework.Vault.Repositories.Queries;
|
|
using Microsoft.EntityFrameworkCore;
|
|
using Microsoft.Extensions.DependencyInjection;
|
|
|
|
namespace Bit.Infrastructure.EntityFramework.Vault.Repositories;
|
|
|
|
public class SecurityTaskRepository : Repository<Core.Vault.Entities.SecurityTask, SecurityTask, Guid>, ISecurityTaskRepository
|
|
{
|
|
public SecurityTaskRepository(IServiceScopeFactory serviceScopeFactory, IMapper mapper)
|
|
: base(serviceScopeFactory, mapper, (context) => context.SecurityTasks)
|
|
{ }
|
|
|
|
/// <inheritdoc />
|
|
public async Task<ICollection<Core.Vault.Entities.SecurityTask>> GetManyByUserIdStatusAsync(Guid userId,
|
|
SecurityTaskStatus? status = null)
|
|
{
|
|
using var scope = ServiceScopeFactory.CreateScope();
|
|
var dbContext = GetDatabaseContext(scope);
|
|
var query = new SecurityTaskReadByUserIdStatusQuery(userId, status);
|
|
var data = await query.Run(dbContext).ToListAsync();
|
|
return data;
|
|
}
|
|
}
|