mirror of
https://github.com/bitwarden/server.git
synced 2025-07-02 00:22:50 -05:00
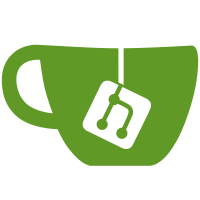
* Implement userkey rotation v2 * Update request models * Cleanup * Update tests * Improve test * Add tests * Fix formatting * Fix test * Remove whitespace * Fix namespace * Enable nullable on models * Fix build * Add tests and enable nullable on masterpasswordunlockdatamodel * Fix test * Remove rollback * Add tests * Make masterpassword hint optional * Update user query * Add EF test * Improve test * Cleanup * Set masterpassword hint * Remove connection close * Add tests for invalid kdf types * Update test/Core.Test/KeyManagement/UserKey/RotateUserAccountKeysCommandTests.cs Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com> * Fix formatting * Update src/Api/KeyManagement/Models/Requests/RotateAccountKeysAndDataRequestModel.cs Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com> * Update src/Api/Auth/Models/Request/Accounts/MasterPasswordUnlockDataModel.cs Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com> * Update src/Api/Auth/Models/Request/Accounts/MasterPasswordUnlockDataModel.cs Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com> * Update src/Api/KeyManagement/Models/Requests/AccountKeysRequestModel.cs Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com> * Fix imports * Fix tests * Remove null check * Add rollback --------- Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com>
121 lines
5.0 KiB
C#
121 lines
5.0 KiB
C#
using Bit.Core.Entities;
|
|
using Bit.Core.KeyManagement.Models.Data;
|
|
using Bit.Core.KeyManagement.UserKey.Implementations;
|
|
using Bit.Core.Services;
|
|
using Bit.Test.Common.AutoFixture;
|
|
using Bit.Test.Common.AutoFixture.Attributes;
|
|
using Microsoft.AspNetCore.Identity;
|
|
using NSubstitute;
|
|
using Xunit;
|
|
|
|
namespace Bit.Core.Test.KeyManagement.UserKey;
|
|
|
|
[SutProviderCustomize]
|
|
public class RotateUserAccountKeysCommandTests
|
|
{
|
|
[Theory, BitAutoData]
|
|
public async Task RejectsWrongOldMasterPassword(SutProvider<RotateUserAccountKeysCommand> sutProvider, User user,
|
|
RotateUserAccountKeysData model)
|
|
{
|
|
user.Email = model.MasterPasswordUnlockData.Email;
|
|
sutProvider.GetDependency<IUserService>().CheckPasswordAsync(user, model.OldMasterKeyAuthenticationHash)
|
|
.Returns(false);
|
|
|
|
var result = await sutProvider.Sut.RotateUserAccountKeysAsync(user, model);
|
|
|
|
Assert.NotEqual(IdentityResult.Success, result);
|
|
}
|
|
[Theory, BitAutoData]
|
|
public async Task ThrowsWhenUserIsNull(SutProvider<RotateUserAccountKeysCommand> sutProvider,
|
|
RotateUserAccountKeysData model)
|
|
{
|
|
await Assert.ThrowsAsync<ArgumentNullException>(async () => await sutProvider.Sut.RotateUserAccountKeysAsync(null, model));
|
|
}
|
|
[Theory, BitAutoData]
|
|
public async Task RejectsEmailChange(SutProvider<RotateUserAccountKeysCommand> sutProvider, User user,
|
|
RotateUserAccountKeysData model)
|
|
{
|
|
user.Kdf = Enums.KdfType.Argon2id;
|
|
user.KdfIterations = 3;
|
|
user.KdfMemory = 64;
|
|
user.KdfParallelism = 4;
|
|
|
|
model.MasterPasswordUnlockData.Email = user.Email + ".different-domain";
|
|
model.MasterPasswordUnlockData.KdfType = Enums.KdfType.Argon2id;
|
|
model.MasterPasswordUnlockData.KdfIterations = 3;
|
|
model.MasterPasswordUnlockData.KdfMemory = 64;
|
|
model.MasterPasswordUnlockData.KdfParallelism = 4;
|
|
sutProvider.GetDependency<IUserService>().CheckPasswordAsync(user, model.OldMasterKeyAuthenticationHash)
|
|
.Returns(true);
|
|
await Assert.ThrowsAsync<InvalidOperationException>(async () => await sutProvider.Sut.RotateUserAccountKeysAsync(user, model));
|
|
}
|
|
|
|
[Theory, BitAutoData]
|
|
public async Task RejectsKdfChange(SutProvider<RotateUserAccountKeysCommand> sutProvider, User user,
|
|
RotateUserAccountKeysData model)
|
|
{
|
|
user.Kdf = Enums.KdfType.Argon2id;
|
|
user.KdfIterations = 3;
|
|
user.KdfMemory = 64;
|
|
user.KdfParallelism = 4;
|
|
|
|
model.MasterPasswordUnlockData.Email = user.Email;
|
|
model.MasterPasswordUnlockData.KdfType = Enums.KdfType.PBKDF2_SHA256;
|
|
model.MasterPasswordUnlockData.KdfIterations = 600000;
|
|
model.MasterPasswordUnlockData.KdfMemory = null;
|
|
model.MasterPasswordUnlockData.KdfParallelism = null;
|
|
sutProvider.GetDependency<IUserService>().CheckPasswordAsync(user, model.OldMasterKeyAuthenticationHash)
|
|
.Returns(true);
|
|
await Assert.ThrowsAsync<InvalidOperationException>(async () => await sutProvider.Sut.RotateUserAccountKeysAsync(user, model));
|
|
}
|
|
|
|
|
|
[Theory, BitAutoData]
|
|
public async Task RejectsPublicKeyChange(SutProvider<RotateUserAccountKeysCommand> sutProvider, User user,
|
|
RotateUserAccountKeysData model)
|
|
{
|
|
user.PublicKey = "old-public";
|
|
user.Kdf = Enums.KdfType.Argon2id;
|
|
user.KdfIterations = 3;
|
|
user.KdfMemory = 64;
|
|
user.KdfParallelism = 4;
|
|
|
|
model.AccountPublicKey = "new-public";
|
|
model.MasterPasswordUnlockData.Email = user.Email;
|
|
model.MasterPasswordUnlockData.KdfType = Enums.KdfType.Argon2id;
|
|
model.MasterPasswordUnlockData.KdfIterations = 3;
|
|
model.MasterPasswordUnlockData.KdfMemory = 64;
|
|
model.MasterPasswordUnlockData.KdfParallelism = 4;
|
|
|
|
sutProvider.GetDependency<IUserService>().CheckPasswordAsync(user, model.OldMasterKeyAuthenticationHash)
|
|
.Returns(true);
|
|
|
|
await Assert.ThrowsAsync<InvalidOperationException>(async () => await sutProvider.Sut.RotateUserAccountKeysAsync(user, model));
|
|
}
|
|
|
|
[Theory, BitAutoData]
|
|
public async Task RotatesCorrectly(SutProvider<RotateUserAccountKeysCommand> sutProvider, User user,
|
|
RotateUserAccountKeysData model)
|
|
{
|
|
user.Kdf = Enums.KdfType.Argon2id;
|
|
user.KdfIterations = 3;
|
|
user.KdfMemory = 64;
|
|
user.KdfParallelism = 4;
|
|
|
|
model.MasterPasswordUnlockData.Email = user.Email;
|
|
model.MasterPasswordUnlockData.KdfType = Enums.KdfType.Argon2id;
|
|
model.MasterPasswordUnlockData.KdfIterations = 3;
|
|
model.MasterPasswordUnlockData.KdfMemory = 64;
|
|
model.MasterPasswordUnlockData.KdfParallelism = 4;
|
|
|
|
model.AccountPublicKey = user.PublicKey;
|
|
|
|
sutProvider.GetDependency<IUserService>().CheckPasswordAsync(user, model.OldMasterKeyAuthenticationHash)
|
|
.Returns(true);
|
|
|
|
var result = await sutProvider.Sut.RotateUserAccountKeysAsync(user, model);
|
|
|
|
Assert.Equal(IdentityResult.Success, result);
|
|
}
|
|
}
|