mirror of
https://github.com/bitwarden/server.git
synced 2025-04-09 23:28:12 -05:00
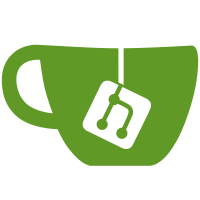
* Add command interface and implementation for disabling organizations * Register organization disable command for dependency injection * Add unit tests for OrganizationDisableCommand * Refactor subscription handlers to use IOrganizationDisableCommand for disabling organizations * Remove DisableAsync method from IOrganizationService and its implementation in OrganizationService * Remove IOrganizationService dependency from SubscriptionDeletedHandler * Remove commented TODO for sending email to owners in OrganizationDisableCommand
34 lines
1.2 KiB
C#
34 lines
1.2 KiB
C#
using Bit.Core.AdminConsole.OrganizationFeatures.Organizations.Interfaces;
|
|
using Bit.Core.Repositories;
|
|
using Bit.Core.Services;
|
|
|
|
namespace Bit.Core.AdminConsole.OrganizationFeatures.Organizations;
|
|
|
|
public class OrganizationDisableCommand : IOrganizationDisableCommand
|
|
{
|
|
private readonly IOrganizationRepository _organizationRepository;
|
|
private readonly IApplicationCacheService _applicationCacheService;
|
|
|
|
public OrganizationDisableCommand(
|
|
IOrganizationRepository organizationRepository,
|
|
IApplicationCacheService applicationCacheService)
|
|
{
|
|
_organizationRepository = organizationRepository;
|
|
_applicationCacheService = applicationCacheService;
|
|
}
|
|
|
|
public async Task DisableAsync(Guid organizationId, DateTime? expirationDate)
|
|
{
|
|
var organization = await _organizationRepository.GetByIdAsync(organizationId);
|
|
if (organization is { Enabled: true })
|
|
{
|
|
organization.Enabled = false;
|
|
organization.ExpirationDate = expirationDate;
|
|
organization.RevisionDate = DateTime.UtcNow;
|
|
|
|
await _organizationRepository.ReplaceAsync(organization);
|
|
await _applicationCacheService.UpsertOrganizationAbilityAsync(organization);
|
|
}
|
|
}
|
|
}
|