mirror of
https://github.com/bitwarden/server.git
synced 2025-04-05 21:18:13 -05:00
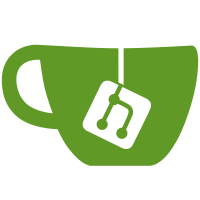
* Remove gRPC and convert PricingClient to HttpClient wrapper * Add PlanType.GetProductTier extension Many instances of StaticStore use are just to get the ProductTierType of a PlanType, but this can be derived from the PlanType itself without having to fetch the entire plan. * Remove invocations of the StaticStore in non-Test code * Deprecate StaticStore entry points * Run dotnet format * Matt's feedback * Run dotnet format * Rui's feedback * Run dotnet format * Replacements since approval * Run dotnet format
39 lines
2.2 KiB
C#
39 lines
2.2 KiB
C#
using Bit.Core.Billing.Enums;
|
|
using Bit.Core.Exceptions;
|
|
using Bit.Core.Models.StaticStore;
|
|
using Bit.Core.Utilities;
|
|
|
|
#nullable enable
|
|
|
|
namespace Bit.Core.Billing.Pricing;
|
|
|
|
public interface IPricingClient
|
|
{
|
|
/// <summary>
|
|
/// Retrieve a Bitwarden plan by its <paramref name="planType"/>. If the feature flag 'use-pricing-service' is enabled,
|
|
/// this will trigger a request to the Bitwarden Pricing Service. Otherwise, it will use the existing <see cref="StaticStore"/>.
|
|
/// </summary>
|
|
/// <param name="planType">The type of plan to retrieve.</param>
|
|
/// <returns>A Bitwarden <see cref="Plan"/> record or null in the case the plan could not be found or the method was executed from a self-hosted instance.</returns>
|
|
/// <exception cref="BillingException">Thrown when the request to the Pricing Service fails unexpectedly.</exception>
|
|
Task<Plan?> GetPlan(PlanType planType);
|
|
|
|
/// <summary>
|
|
/// Retrieve a Bitwarden plan by its <paramref name="planType"/>. If the feature flag 'use-pricing-service' is enabled,
|
|
/// this will trigger a request to the Bitwarden Pricing Service. Otherwise, it will use the existing <see cref="StaticStore"/>.
|
|
/// </summary>
|
|
/// <param name="planType">The type of plan to retrieve.</param>
|
|
/// <returns>A Bitwarden <see cref="Plan"/> record.</returns>
|
|
/// <exception cref="NotFoundException">Thrown when the <see cref="Plan"/> for the provided <paramref name="planType"/> could not be found or the method was executed from a self-hosted instance.</exception>
|
|
/// <exception cref="BillingException">Thrown when the request to the Pricing Service fails unexpectedly.</exception>
|
|
Task<Plan> GetPlanOrThrow(PlanType planType);
|
|
|
|
/// <summary>
|
|
/// Retrieve all the Bitwarden plans. If the feature flag 'use-pricing-service' is enabled,
|
|
/// this will trigger a request to the Bitwarden Pricing Service. Otherwise, it will use the existing <see cref="StaticStore"/>.
|
|
/// </summary>
|
|
/// <returns>A list of Bitwarden <see cref="Plan"/> records or an empty list in the case the method is executed from a self-hosted instance.</returns>
|
|
/// <exception cref="BillingException">Thrown when the request to the Pricing Service fails unexpectedly.</exception>
|
|
Task<List<Plan>> ListPlans();
|
|
}
|