mirror of
https://github.com/bitwarden/server.git
synced 2025-07-13 21:57:30 -05:00
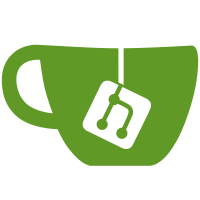
* Initial stubbing out of the phishing service * Add the phishing domain controller * Add changes for the phishing domain get * Add distributed cache to the phishing domain Signed-off-by: Cy Okeke <cokeke@bitwarden.com> * Rename the variable name Signed-off-by: Cy Okeke <cokeke@bitwarden.com> * Removed IPhishingDomainService * Feature/phishing detection cronjob (#5512) * Added caching to EF implementation. Added error handling and logging * Refactored update method to use sqlbulkcopy instead of performing a round trip for each new insert * Initial implementation for quartz job to get list of phishing domains * Updated phishing domain settings to be its own interface * Add phishing domain detection with checksum-based updates * Updated auth for phishing domain endpoints to either require api, or licensing claims to support both web and browser clients, and selfhost api clients * [Innovation Sprint] Updated Phishing domains to rely on blob storage (#5517) * Updated phishing detection data layer to rely on azure blob storage instead of sql server * dotnet format * Took rider refactors * Ensuring phishing.testcategory.com exists to test against * Added redis to dev's docker-compose * Removed redis from cloud profile * Remove the Authorize attribute * error whitespace fix whitespace formatting * error WHITESPACE: Fix whitespace formatting * Wrapped phishing detection feature behind feature flag (#5532) * Increased timeout for fetching source list a bunch * Removed PhishingDomains policy --------- Signed-off-by: Cy Okeke <cokeke@bitwarden.com> Co-authored-by: Cy Okeke <cokeke@bitwarden.com>
115 lines
4.6 KiB
C#
115 lines
4.6 KiB
C#
using Bit.Api.Auth.Jobs;
|
|
using Bit.Core.Jobs;
|
|
using Bit.Core.Settings;
|
|
using Quartz;
|
|
|
|
namespace Bit.Api.Jobs;
|
|
|
|
public class JobsHostedService : BaseJobsHostedService
|
|
{
|
|
public JobsHostedService(
|
|
GlobalSettings globalSettings,
|
|
IServiceProvider serviceProvider,
|
|
ILogger<JobsHostedService> logger,
|
|
ILogger<JobListener> listenerLogger)
|
|
: base(globalSettings, serviceProvider, logger, listenerLogger) { }
|
|
|
|
public override async Task StartAsync(CancellationToken cancellationToken)
|
|
{
|
|
var everyTopOfTheHourTrigger = TriggerBuilder.Create()
|
|
.WithIdentity("EveryTopOfTheHourTrigger")
|
|
.StartNow()
|
|
.WithCronSchedule("0 0 * * * ?")
|
|
.Build();
|
|
var emergencyAccessNotificationTrigger = TriggerBuilder.Create()
|
|
.WithIdentity("EmergencyAccessNotificationTrigger")
|
|
.StartNow()
|
|
.WithCronSchedule("0 0 * * * ?")
|
|
.Build();
|
|
var emergencyAccessTimeoutTrigger = TriggerBuilder.Create()
|
|
.WithIdentity("EmergencyAccessTimeoutTrigger")
|
|
.StartNow()
|
|
.WithCronSchedule("0 0 * * * ?")
|
|
.Build();
|
|
var everyTopOfTheSixthHourTrigger = TriggerBuilder.Create()
|
|
.WithIdentity("EveryTopOfTheSixthHourTrigger")
|
|
.StartNow()
|
|
.WithCronSchedule("0 0 */6 * * ?")
|
|
.Build();
|
|
var everyTwelfthHourAndThirtyMinutesTrigger = TriggerBuilder.Create()
|
|
.WithIdentity("EveryTwelfthHourAndThirtyMinutesTrigger")
|
|
.StartNow()
|
|
.WithCronSchedule("0 30 */12 * * ?")
|
|
.Build();
|
|
var smTrashCleanupTrigger = TriggerBuilder.Create()
|
|
.WithIdentity("SMTrashCleanupTrigger")
|
|
.StartNow()
|
|
.WithCronSchedule("0 0 22 * * ?")
|
|
.Build();
|
|
var randomDailySponsorshipSyncTrigger = TriggerBuilder.Create()
|
|
.WithIdentity("RandomDailySponsorshipSyncTrigger")
|
|
.StartAt(DateBuilder.FutureDate(new Random().Next(24), IntervalUnit.Hour))
|
|
.WithSimpleSchedule(x => x
|
|
.WithIntervalInHours(24)
|
|
.RepeatForever())
|
|
.Build();
|
|
var validateOrganizationDomainTrigger = TriggerBuilder.Create()
|
|
.WithIdentity("ValidateOrganizationDomainTrigger")
|
|
.StartNow()
|
|
.WithCronSchedule("0 0 * * * ?")
|
|
.Build();
|
|
var updatePhishingDomainsTrigger = TriggerBuilder.Create()
|
|
.WithIdentity("UpdatePhishingDomainsTrigger")
|
|
.StartNow()
|
|
.WithSimpleSchedule(x => x
|
|
.WithIntervalInHours(24)
|
|
.RepeatForever())
|
|
.Build();
|
|
|
|
|
|
var jobs = new List<Tuple<Type, ITrigger>>
|
|
{
|
|
new Tuple<Type, ITrigger>(typeof(AliveJob), everyTopOfTheHourTrigger),
|
|
new Tuple<Type, ITrigger>(typeof(EmergencyAccessNotificationJob), emergencyAccessNotificationTrigger),
|
|
new Tuple<Type, ITrigger>(typeof(EmergencyAccessTimeoutJob), emergencyAccessTimeoutTrigger),
|
|
new Tuple<Type, ITrigger>(typeof(ValidateUsersJob), everyTopOfTheSixthHourTrigger),
|
|
new Tuple<Type, ITrigger>(typeof(ValidateOrganizationsJob), everyTwelfthHourAndThirtyMinutesTrigger),
|
|
new Tuple<Type, ITrigger>(typeof(ValidateOrganizationDomainJob), validateOrganizationDomainTrigger),
|
|
new Tuple<Type, ITrigger>(typeof(UpdatePhishingDomainsJob), updatePhishingDomainsTrigger),
|
|
};
|
|
|
|
if (_globalSettings.SelfHosted && _globalSettings.EnableCloudCommunication)
|
|
{
|
|
jobs.Add(new Tuple<Type, ITrigger>(typeof(SelfHostedSponsorshipSyncJob), randomDailySponsorshipSyncTrigger));
|
|
}
|
|
|
|
#if !OSS
|
|
jobs.Add(new Tuple<Type, ITrigger>(typeof(EmptySecretsManagerTrashJob), smTrashCleanupTrigger));
|
|
#endif
|
|
|
|
Jobs = jobs;
|
|
|
|
await base.StartAsync(cancellationToken);
|
|
}
|
|
|
|
public static void AddJobsServices(IServiceCollection services, bool selfHosted)
|
|
{
|
|
if (selfHosted)
|
|
{
|
|
services.AddTransient<SelfHostedSponsorshipSyncJob>();
|
|
}
|
|
services.AddTransient<AliveJob>();
|
|
services.AddTransient<EmergencyAccessNotificationJob>();
|
|
services.AddTransient<EmergencyAccessTimeoutJob>();
|
|
services.AddTransient<ValidateUsersJob>();
|
|
services.AddTransient<ValidateOrganizationsJob>();
|
|
services.AddTransient<ValidateOrganizationDomainJob>();
|
|
services.AddTransient<UpdatePhishingDomainsJob>();
|
|
}
|
|
|
|
public static void AddCommercialSecretsManagerJobServices(IServiceCollection services)
|
|
{
|
|
services.AddTransient<EmptySecretsManagerTrashJob>();
|
|
}
|
|
}
|