mirror of
https://github.com/bitwarden/server.git
synced 2025-04-05 05:00:19 -05:00
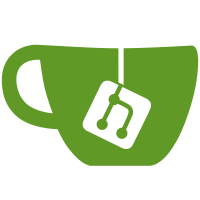
* Add autoscale fields to Organization * Add autoscale setting changes * Autoscale organizations updates InviteUsersAsync to support all invite sources. sends an email to org owners when organization autoscaled * All organizations autoscale Disabling autoscaling can be done by setting max seats to current seats. We only warn about autoscaling on the first autoscaling event. * Fix tests * Bug fixes * Simplify subscription update logic * Void invoices that fail to delete Stripe no longer allows deletion of draft invoices that were created as part of subscription updates. It's necessary to void out these invoices without sending tem to the client. * Notify org owners when their subscription runs out of seats * Use datetime for notifications Allows for later re-sending email if we want to periodically remind owners * Do not update subscription if it already matches new quatity * Include all migrations * Remove unnecessary inline styling * SubscriptionUpdate handles update decisions * Remove unnecessary html setter * PR review * Use minimum access for class methods
205 lines
6.2 KiB
C#
205 lines
6.2 KiB
C#
using System;
|
|
using Bit.Core.Utilities;
|
|
using Bit.Core.Enums;
|
|
using System.Collections.Generic;
|
|
using Newtonsoft.Json;
|
|
using System.Linq;
|
|
using System.ComponentModel.DataAnnotations;
|
|
|
|
namespace Bit.Core.Models.Table
|
|
{
|
|
public class Organization : ITableObject<Guid>, ISubscriber, IStorable, IStorableSubscriber, IRevisable, IReferenceable
|
|
{
|
|
private Dictionary<TwoFactorProviderType, TwoFactorProvider> _twoFactorProviders;
|
|
|
|
public Guid Id { get; set; }
|
|
[MaxLength(50)]
|
|
public string Identifier { get; set; }
|
|
[MaxLength(50)]
|
|
public string Name { get; set; }
|
|
[MaxLength(50)]
|
|
public string BusinessName { get; set; }
|
|
[MaxLength(50)]
|
|
public string BusinessAddress1 { get; set; }
|
|
[MaxLength(50)]
|
|
public string BusinessAddress2 { get; set; }
|
|
[MaxLength(50)]
|
|
public string BusinessAddress3 { get; set; }
|
|
[MaxLength(2)]
|
|
public string BusinessCountry { get; set; }
|
|
[MaxLength(30)]
|
|
public string BusinessTaxNumber { get; set; }
|
|
[MaxLength(256)]
|
|
public string BillingEmail { get; set; }
|
|
[MaxLength(50)]
|
|
public string Plan { get; set; }
|
|
public PlanType PlanType { get; set; }
|
|
public int? Seats { get; set; }
|
|
public short? MaxCollections { get; set; }
|
|
public bool UsePolicies { get; set; }
|
|
public bool UseSso { get; set; }
|
|
public bool UseGroups { get; set; }
|
|
public bool UseDirectory { get; set; }
|
|
public bool UseEvents { get; set; }
|
|
public bool UseTotp { get; set; }
|
|
public bool Use2fa { get; set; }
|
|
public bool UseApi { get; set; }
|
|
public bool UseResetPassword { get; set; }
|
|
public bool SelfHost { get; set; }
|
|
public bool UsersGetPremium { get; set; }
|
|
public long? Storage { get; set; }
|
|
public short? MaxStorageGb { get; set; }
|
|
public GatewayType? Gateway { get; set; }
|
|
[MaxLength(50)]
|
|
public string GatewayCustomerId { get; set; }
|
|
[MaxLength(50)]
|
|
public string GatewaySubscriptionId { get; set; }
|
|
public string ReferenceData { get; set; }
|
|
public bool Enabled { get; set; } = true;
|
|
[MaxLength(100)]
|
|
public string LicenseKey { get; set; }
|
|
[MaxLength(30)]
|
|
public string ApiKey { get; set; }
|
|
public string PublicKey { get; set; }
|
|
public string PrivateKey { get; set; }
|
|
public string TwoFactorProviders { get; set; }
|
|
public DateTime? ExpirationDate { get; set; }
|
|
public DateTime CreationDate { get; internal set; } = DateTime.UtcNow;
|
|
public DateTime RevisionDate { get; internal set; } = DateTime.UtcNow;
|
|
public int? MaxAutoscaleSeats { get; set; } = null;
|
|
public DateTime? OwnersNotifiedOfAutoscaling { get; set; } = null;
|
|
|
|
public void SetNewId()
|
|
{
|
|
if (Id == default(Guid))
|
|
{
|
|
Id = CoreHelpers.GenerateComb();
|
|
}
|
|
}
|
|
|
|
public string BillingEmailAddress()
|
|
{
|
|
return BillingEmail?.ToLowerInvariant()?.Trim();
|
|
}
|
|
|
|
public string BillingName()
|
|
{
|
|
return BusinessName;
|
|
}
|
|
|
|
public string BraintreeCustomerIdPrefix()
|
|
{
|
|
return "o";
|
|
}
|
|
|
|
public string BraintreeIdField()
|
|
{
|
|
return "organization_id";
|
|
}
|
|
|
|
public string GatewayIdField()
|
|
{
|
|
return "organizationId";
|
|
}
|
|
|
|
public bool IsUser()
|
|
{
|
|
return false;
|
|
}
|
|
|
|
public long StorageBytesRemaining()
|
|
{
|
|
if (!MaxStorageGb.HasValue)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
return StorageBytesRemaining(MaxStorageGb.Value);
|
|
}
|
|
|
|
public long StorageBytesRemaining(short maxStorageGb)
|
|
{
|
|
var maxStorageBytes = maxStorageGb * 1073741824L;
|
|
if (!Storage.HasValue)
|
|
{
|
|
return maxStorageBytes;
|
|
}
|
|
|
|
return maxStorageBytes - Storage.Value;
|
|
}
|
|
|
|
public Dictionary<TwoFactorProviderType, TwoFactorProvider> GetTwoFactorProviders()
|
|
{
|
|
if (string.IsNullOrWhiteSpace(TwoFactorProviders))
|
|
{
|
|
return null;
|
|
}
|
|
|
|
try
|
|
{
|
|
if (_twoFactorProviders == null)
|
|
{
|
|
_twoFactorProviders =
|
|
JsonConvert.DeserializeObject<Dictionary<TwoFactorProviderType, TwoFactorProvider>>(
|
|
TwoFactorProviders);
|
|
}
|
|
|
|
return _twoFactorProviders;
|
|
}
|
|
catch (JsonSerializationException)
|
|
{
|
|
return null;
|
|
}
|
|
}
|
|
|
|
public void SetTwoFactorProviders(Dictionary<TwoFactorProviderType, TwoFactorProvider> providers)
|
|
{
|
|
if (!providers.Any())
|
|
{
|
|
TwoFactorProviders = null;
|
|
_twoFactorProviders = null;
|
|
return;
|
|
}
|
|
|
|
TwoFactorProviders = JsonConvert.SerializeObject(providers, new JsonSerializerSettings
|
|
{
|
|
ContractResolver = new EnumKeyResolver<byte>()
|
|
});
|
|
_twoFactorProviders = providers;
|
|
}
|
|
|
|
public bool TwoFactorProviderIsEnabled(TwoFactorProviderType provider)
|
|
{
|
|
var providers = GetTwoFactorProviders();
|
|
if (providers == null || !providers.ContainsKey(provider))
|
|
{
|
|
return false;
|
|
}
|
|
|
|
return providers[provider].Enabled && Use2fa;
|
|
}
|
|
|
|
public bool TwoFactorIsEnabled()
|
|
{
|
|
var providers = GetTwoFactorProviders();
|
|
if (providers == null)
|
|
{
|
|
return false;
|
|
}
|
|
|
|
return providers.Any(p => (p.Value?.Enabled ?? false) && Use2fa);
|
|
}
|
|
|
|
public TwoFactorProvider GetTwoFactorProvider(TwoFactorProviderType provider)
|
|
{
|
|
var providers = GetTwoFactorProviders();
|
|
if (providers == null || !providers.ContainsKey(provider))
|
|
{
|
|
return null;
|
|
}
|
|
|
|
return providers[provider];
|
|
}
|
|
}
|
|
}
|