mirror of
https://github.com/bitwarden/server.git
synced 2025-04-16 02:28:13 -05:00
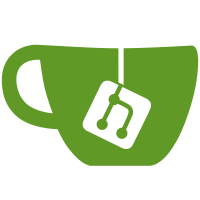
* Adjust email address checking to handle unicode * ASCII only in local part * allow unicode in second-level and top-level domain * Add PunyEncoding/Decoding methods and tests * Use PunyEncoding for outbound email recipients * Use MailKit for punycode, handle edge cases * Punyencode all email addresses in mailServices * Remove punyencoding from HandlebarsMailService * Add to punyencoding tests * Use more inclusive e-mail error * Fix comment wording * Apply StrictEmail checking to emergency access invite * Remove punyDecode helper
54 lines
1.7 KiB
C#
54 lines
1.7 KiB
C#
using System.ComponentModel.DataAnnotations;
|
|
using System.Text.RegularExpressions;
|
|
using MimeKit;
|
|
|
|
namespace Bit.Core.Utilities
|
|
{
|
|
public class StrictEmailAddressAttribute : ValidationAttribute
|
|
{
|
|
public StrictEmailAddressAttribute()
|
|
: base("The {0} field is not a supported e-mail address format.")
|
|
{}
|
|
|
|
public override bool IsValid(object value)
|
|
{
|
|
var emailAddress = value?.ToString();
|
|
if (emailAddress == null)
|
|
{
|
|
return false;
|
|
}
|
|
|
|
try
|
|
{
|
|
var parsedEmailAddress = MailboxAddress.Parse(emailAddress).Address;
|
|
if (parsedEmailAddress != emailAddress)
|
|
{
|
|
return false;
|
|
}
|
|
}
|
|
catch (ParseException)
|
|
{
|
|
return false;
|
|
}
|
|
|
|
/**
|
|
The regex below is intended to catch edge cases that are not handled by the general parsing check above.
|
|
This enforces the following rules:
|
|
* Requires ASCII only in the local-part (code points 0-127)
|
|
* Requires an @ symbol
|
|
* Allows any char in second-level domain name, including unicode and symbols
|
|
* Requires at least one period (.) separating SLD from TLD
|
|
* Must end in a letter (including unicode)
|
|
See the unit tests for examples of what is allowed.
|
|
**/
|
|
var emailFormat = @"[\x00-\x7F]+@.+\.\p{L}+$";
|
|
if (!Regex.IsMatch(emailAddress, emailFormat))
|
|
{
|
|
return false;
|
|
}
|
|
|
|
return new EmailAddressAttribute().IsValid(emailAddress);
|
|
}
|
|
}
|
|
}
|