mirror of
https://github.com/bitwarden/server.git
synced 2025-07-06 10:32:49 -05:00
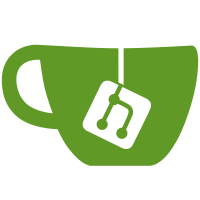
* [PM-4619] feat: scaffold new create options command * [PM-4169] feat: implement credential create options command * [PM-4619] feat: create command for credential creation * [PM-4619] feat: create assertion options command * [PM-4619] chore: clean-up unused argument * [PM-4619] feat: implement assertion command * [PM-4619] feat: migrate to commands * [PM-4619] fix: lint * [PM-4169] fix: use constant * [PM-4619] fix: lint I have no idea what this commit acutally changes, but the file seems to have some character encoding issues. This fix was generated by `dotnet format`
108 lines
5.0 KiB
C#
108 lines
5.0 KiB
C#
using System.Text;
|
|
using Bit.Core.Auth.Entities;
|
|
using Bit.Core.Auth.Repositories;
|
|
using Bit.Core.Auth.UserFeatures.WebAuthnLogin.Implementations;
|
|
using Bit.Core.Entities;
|
|
using Bit.Core.Exceptions;
|
|
using Bit.Core.Repositories;
|
|
using Bit.Core.Utilities;
|
|
using Bit.Test.Common.AutoFixture;
|
|
using Bit.Test.Common.AutoFixture.Attributes;
|
|
using Fido2NetLib;
|
|
using Fido2NetLib.Objects;
|
|
using NSubstitute;
|
|
using NSubstitute.ReturnsExtensions;
|
|
using Xunit;
|
|
|
|
namespace Bit.Core.Test.Auth.UserFeatures.WebAuthnLogin;
|
|
|
|
[SutProviderCustomize]
|
|
public class AssertWebAuthnLoginCredentialCommandTests
|
|
{
|
|
[Theory, BitAutoData]
|
|
internal async void InvalidUserHandle_ThrowsBadRequestException(SutProvider<AssertWebAuthnLoginCredentialCommand> sutProvider, AssertionOptions options, AuthenticatorAssertionRawResponse response)
|
|
{
|
|
// Arrange
|
|
response.Response.UserHandle = Encoding.UTF8.GetBytes("invalid-user-handle");
|
|
|
|
// Act
|
|
var result = async () => await sutProvider.Sut.AssertWebAuthnLoginCredential(options, response);
|
|
|
|
// Assert
|
|
await Assert.ThrowsAsync<BadRequestException>(result);
|
|
}
|
|
|
|
[Theory, BitAutoData]
|
|
internal async void UserNotFound_ThrowsBadRequestException(SutProvider<AssertWebAuthnLoginCredentialCommand> sutProvider, User user, AssertionOptions options, AuthenticatorAssertionRawResponse response)
|
|
{
|
|
// Arrange
|
|
response.Response.UserHandle = user.Id.ToByteArray();
|
|
sutProvider.GetDependency<IUserRepository>().GetByIdAsync(user.Id).ReturnsNull();
|
|
|
|
// Act
|
|
var result = async () => await sutProvider.Sut.AssertWebAuthnLoginCredential(options, response);
|
|
|
|
// Assert
|
|
await Assert.ThrowsAsync<BadRequestException>(result);
|
|
}
|
|
|
|
[Theory, BitAutoData]
|
|
internal async void NoMatchingCredentialExists_ThrowsBadRequestException(SutProvider<AssertWebAuthnLoginCredentialCommand> sutProvider, User user, AssertionOptions options, AuthenticatorAssertionRawResponse response)
|
|
{
|
|
// Arrange
|
|
response.Response.UserHandle = user.Id.ToByteArray();
|
|
sutProvider.GetDependency<IUserRepository>().GetByIdAsync(user.Id).Returns(user);
|
|
sutProvider.GetDependency<IWebAuthnCredentialRepository>().GetManyByUserIdAsync(user.Id).Returns(new WebAuthnCredential[] { });
|
|
|
|
// Act
|
|
var result = async () => await sutProvider.Sut.AssertWebAuthnLoginCredential(options, response);
|
|
|
|
// Assert
|
|
await Assert.ThrowsAsync<BadRequestException>(result);
|
|
}
|
|
|
|
[Theory, BitAutoData]
|
|
internal async void AssertionFails_ThrowsBadRequestException(SutProvider<AssertWebAuthnLoginCredentialCommand> sutProvider, User user, AssertionOptions options, AuthenticatorAssertionRawResponse response, WebAuthnCredential credential, AssertionVerificationResult assertionResult)
|
|
{
|
|
// Arrange
|
|
var credentialId = Guid.NewGuid().ToByteArray();
|
|
credential.CredentialId = CoreHelpers.Base64UrlEncode(credentialId);
|
|
response.Id = credentialId;
|
|
response.Response.UserHandle = user.Id.ToByteArray();
|
|
assertionResult.Status = "Not ok";
|
|
sutProvider.GetDependency<IUserRepository>().GetByIdAsync(user.Id).Returns(user);
|
|
sutProvider.GetDependency<IWebAuthnCredentialRepository>().GetManyByUserIdAsync(user.Id).Returns(new WebAuthnCredential[] { credential });
|
|
sutProvider.GetDependency<IFido2>().MakeAssertionAsync(response, options, Arg.Any<byte[]>(), Arg.Any<uint>(), Arg.Any<IsUserHandleOwnerOfCredentialIdAsync>())
|
|
.Returns(assertionResult);
|
|
|
|
// Act
|
|
var result = async () => await sutProvider.Sut.AssertWebAuthnLoginCredential(options, response);
|
|
|
|
// Assert
|
|
await Assert.ThrowsAsync<BadRequestException>(result);
|
|
}
|
|
|
|
[Theory, BitAutoData]
|
|
internal async void AssertionSucceeds_ReturnsUserAndCredential(SutProvider<AssertWebAuthnLoginCredentialCommand> sutProvider, User user, AssertionOptions options, AuthenticatorAssertionRawResponse response, WebAuthnCredential credential, AssertionVerificationResult assertionResult)
|
|
{
|
|
// Arrange
|
|
var credentialId = Guid.NewGuid().ToByteArray();
|
|
credential.CredentialId = CoreHelpers.Base64UrlEncode(credentialId);
|
|
response.Id = credentialId;
|
|
response.Response.UserHandle = user.Id.ToByteArray();
|
|
assertionResult.Status = "ok";
|
|
sutProvider.GetDependency<IUserRepository>().GetByIdAsync(user.Id).Returns(user);
|
|
sutProvider.GetDependency<IWebAuthnCredentialRepository>().GetManyByUserIdAsync(user.Id).Returns(new WebAuthnCredential[] { credential });
|
|
sutProvider.GetDependency<IFido2>().MakeAssertionAsync(response, options, Arg.Any<byte[]>(), Arg.Any<uint>(), Arg.Any<IsUserHandleOwnerOfCredentialIdAsync>())
|
|
.Returns(assertionResult);
|
|
|
|
// Act
|
|
var result = await sutProvider.Sut.AssertWebAuthnLoginCredential(options, response);
|
|
|
|
// Assert
|
|
var (userResult, credentialResult) = result;
|
|
Assert.Equal(user, userResult);
|
|
Assert.Equal(credential, credentialResult);
|
|
}
|
|
}
|