mirror of
https://github.com/bitwarden/server.git
synced 2025-07-05 10:02:47 -05:00
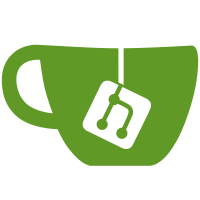
* [PM-4619] feat: scaffold new create options command * [PM-4169] feat: implement credential create options command * [PM-4619] feat: create command for credential creation * [PM-4619] feat: create assertion options command * [PM-4619] chore: clean-up unused argument * [PM-4619] feat: implement assertion command * [PM-4619] feat: migrate to commands * [PM-4619] fix: lint * [PM-4169] fix: use constant * [PM-4619] fix: lint I have no idea what this commit acutally changes, but the file seems to have some character encoding issues. This fix was generated by `dotnet format`
66 lines
3.0 KiB
C#
66 lines
3.0 KiB
C#
using AutoFixture;
|
|
using Bit.Core.Auth.Entities;
|
|
using Bit.Core.Auth.Repositories;
|
|
using Bit.Core.Auth.UserFeatures.WebAuthnLogin.Implementations;
|
|
using Bit.Core.Entities;
|
|
using Bit.Test.Common.AutoFixture;
|
|
using Bit.Test.Common.AutoFixture.Attributes;
|
|
using Fido2NetLib;
|
|
using Fido2NetLib.Objects;
|
|
using NSubstitute;
|
|
using Xunit;
|
|
using static Fido2NetLib.Fido2;
|
|
|
|
namespace Bit.Core.Test.Auth.UserFeatures.WebAuthnLogin;
|
|
|
|
[SutProviderCustomize]
|
|
public class CreateWebAuthnLoginCredentialCommandTests
|
|
{
|
|
[Theory, BitAutoData]
|
|
internal async void ExceedsExistingCredentialsLimit_ReturnsFalse(SutProvider<CreateWebAuthnLoginCredentialCommand> sutProvider, User user, CredentialCreateOptions options, AuthenticatorAttestationRawResponse response, Generator<WebAuthnCredential> credentialGenerator)
|
|
{
|
|
// Arrange
|
|
var existingCredentials = credentialGenerator.Take(CreateWebAuthnLoginCredentialCommand.MaxCredentialsPerUser).ToList();
|
|
sutProvider.GetDependency<IWebAuthnCredentialRepository>().GetManyByUserIdAsync(user.Id).Returns(existingCredentials);
|
|
|
|
// Act
|
|
var result = await sutProvider.Sut.CreateWebAuthnLoginCredentialAsync(user, "name", options, response, false, null, null, null);
|
|
|
|
// Assert
|
|
Assert.False(result);
|
|
await sutProvider.GetDependency<IWebAuthnCredentialRepository>().DidNotReceive().CreateAsync(Arg.Any<WebAuthnCredential>());
|
|
}
|
|
|
|
[Theory, BitAutoData]
|
|
internal async void DoesNotExceedExistingCredentialsLimit_CreatesCredential(SutProvider<CreateWebAuthnLoginCredentialCommand> sutProvider, User user, CredentialCreateOptions options, AuthenticatorAttestationRawResponse response, Generator<WebAuthnCredential> credentialGenerator)
|
|
{
|
|
// Arrange
|
|
var existingCredentials = credentialGenerator.Take(CreateWebAuthnLoginCredentialCommand.MaxCredentialsPerUser - 1).ToList();
|
|
sutProvider.GetDependency<IWebAuthnCredentialRepository>().GetManyByUserIdAsync(user.Id).Returns(existingCredentials);
|
|
sutProvider.GetDependency<IFido2>().MakeNewCredentialAsync(
|
|
response, options, Arg.Any<IsCredentialIdUniqueToUserAsyncDelegate>(), Arg.Any<byte[]>(), Arg.Any<CancellationToken>()
|
|
).Returns(MakeCredentialResult());
|
|
|
|
// Act
|
|
var result = await sutProvider.Sut.CreateWebAuthnLoginCredentialAsync(user, "name", options, response, false, null, null, null);
|
|
|
|
// Assert
|
|
Assert.True(result);
|
|
await sutProvider.GetDependency<IWebAuthnCredentialRepository>().Received().CreateAsync(Arg.Any<WebAuthnCredential>());
|
|
}
|
|
|
|
private CredentialMakeResult MakeCredentialResult()
|
|
{
|
|
return new CredentialMakeResult("ok", "", new AttestationVerificationSuccess
|
|
{
|
|
Aaguid = new Guid(),
|
|
Counter = 0,
|
|
CredentialId = new Guid().ToByteArray(),
|
|
CredType = "public-key",
|
|
PublicKey = new byte[0],
|
|
Status = "ok",
|
|
User = new Fido2User(),
|
|
});
|
|
}
|
|
}
|