mirror of
https://github.com/bitwarden/server.git
synced 2025-07-01 16:12:49 -05:00
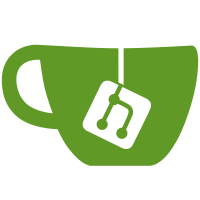
* Move sub-subscription classes to a separate files * Refactor the sub-class to a separate files * format whitespace * remove directive that is unnecessary * Remove the baseSeat class
51 lines
1.6 KiB
C#
51 lines
1.6 KiB
C#
using Bit.Core.Entities;
|
|
using Stripe;
|
|
|
|
namespace Bit.Core.Models.Business;
|
|
|
|
public class SmSeatSubscriptionUpdate : SubscriptionUpdate
|
|
{
|
|
private readonly int _previousSeats;
|
|
private readonly StaticStore.Plan _plan;
|
|
private readonly long? _additionalSeats;
|
|
protected override List<string> PlanIds => new() { _plan.SecretsManager.StripeSeatPlanId };
|
|
|
|
public SmSeatSubscriptionUpdate(Organization organization, StaticStore.Plan plan, long? additionalSeats)
|
|
{
|
|
_plan = plan;
|
|
_additionalSeats = additionalSeats;
|
|
_previousSeats = organization.SmSeats.GetValueOrDefault();
|
|
}
|
|
|
|
public override List<SubscriptionItemOptions> UpgradeItemsOptions(Subscription subscription)
|
|
{
|
|
var item = SubscriptionItem(subscription, PlanIds.Single());
|
|
return new()
|
|
{
|
|
new SubscriptionItemOptions
|
|
{
|
|
Id = item?.Id,
|
|
Plan = PlanIds.Single(),
|
|
Quantity = _additionalSeats,
|
|
Deleted = (item?.Id != null && _additionalSeats == 0) ? true : (bool?)null,
|
|
}
|
|
};
|
|
}
|
|
|
|
public override List<SubscriptionItemOptions> RevertItemsOptions(Subscription subscription)
|
|
{
|
|
|
|
var item = SubscriptionItem(subscription, PlanIds.Single());
|
|
return new()
|
|
{
|
|
new SubscriptionItemOptions
|
|
{
|
|
Id = item?.Id,
|
|
Plan = PlanIds.Single(),
|
|
Quantity = _previousSeats,
|
|
Deleted = _previousSeats == 0 ? true : (bool?)null,
|
|
}
|
|
};
|
|
}
|
|
}
|