mirror of
https://github.com/bitwarden/server.git
synced 2025-04-04 20:50:21 -05:00
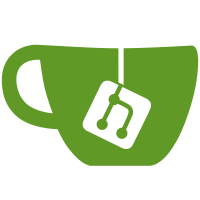
* Implement userkey rotation v2 * Update request models * Cleanup * Update tests * Improve test * Add tests * Fix formatting * Fix test * Remove whitespace * Fix namespace * Enable nullable on models * Fix build * Add tests and enable nullable on masterpasswordunlockdatamodel * Fix test * Remove rollback * Add tests * Make masterpassword hint optional * Update user query * Add EF test * Improve test * Cleanup * Set masterpassword hint * Remove connection close * Add tests for invalid kdf types * Update test/Core.Test/KeyManagement/UserKey/RotateUserAccountKeysCommandTests.cs Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com> * Fix formatting * Update src/Api/KeyManagement/Models/Requests/RotateAccountKeysAndDataRequestModel.cs Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com> * Update src/Api/Auth/Models/Request/Accounts/MasterPasswordUnlockDataModel.cs Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com> * Update src/Api/Auth/Models/Request/Accounts/MasterPasswordUnlockDataModel.cs Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com> * Update src/Api/KeyManagement/Models/Requests/AccountKeysRequestModel.cs Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com> * Fix imports * Fix tests * Add poc for tde rotation * Improve rotation transaction safety * Add validator tests * Clean up validator * Add newline * Add devicekey unlock data to integration test * Fix tests * Fix tests * Remove null check * Remove null check * Fix IsTrusted returning wrong result * Add rollback * Cleanup * Address feedback * Further renames --------- Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com>
54 lines
1.8 KiB
C#
54 lines
1.8 KiB
C#
using Bit.Core.Auth.Models.Api.Request;
|
|
using Bit.Core.Auth.Utilities;
|
|
using Bit.Core.Entities;
|
|
using Bit.Core.Exceptions;
|
|
using Bit.Core.Repositories;
|
|
|
|
namespace Bit.Api.KeyManagement.Validators;
|
|
|
|
/// <summary>
|
|
/// Device implementation for <see cref="IRotationValidator{T,R}"/>
|
|
/// </summary>
|
|
public class DeviceRotationValidator : IRotationValidator<IEnumerable<OtherDeviceKeysUpdateRequestModel>, IEnumerable<Device>>
|
|
{
|
|
private readonly IDeviceRepository _deviceRepository;
|
|
|
|
/// <summary>
|
|
/// Instantiates a new <see cref="DeviceRotationValidator"/>
|
|
/// </summary>
|
|
/// <param name="deviceRepository">Retrieves all user <see cref="Device"/>s</param>
|
|
public DeviceRotationValidator(IDeviceRepository deviceRepository)
|
|
{
|
|
_deviceRepository = deviceRepository;
|
|
}
|
|
|
|
public async Task<IEnumerable<Device>> ValidateAsync(User user, IEnumerable<OtherDeviceKeysUpdateRequestModel> devices)
|
|
{
|
|
var result = new List<Device>();
|
|
|
|
var existingTrustedDevices = (await _deviceRepository.GetManyByUserIdAsync(user.Id)).Where(d => d.IsTrusted()).ToList();
|
|
if (existingTrustedDevices.Count == 0)
|
|
{
|
|
return result;
|
|
}
|
|
|
|
foreach (var existing in existingTrustedDevices)
|
|
{
|
|
var device = devices.FirstOrDefault(c => c.DeviceId == existing.Id);
|
|
if (device == null)
|
|
{
|
|
throw new BadRequestException("All existing trusted devices must be included in the rotation.");
|
|
}
|
|
|
|
if (device.EncryptedUserKey == null || device.EncryptedPublicKey == null)
|
|
{
|
|
throw new BadRequestException("Rotated encryption keys must be provided for all devices that are trusted.");
|
|
}
|
|
|
|
result.Add(device.ToDevice(existing));
|
|
}
|
|
|
|
return result;
|
|
}
|
|
}
|