mirror of
https://github.com/bitwarden/server.git
synced 2025-07-13 05:38:25 -05:00
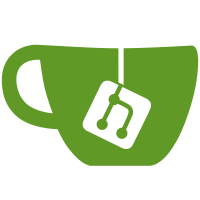
* Remove flag from CreateProviderCommand * Remove flag from OrganizationsController * Consolidate provider extensions * Remove flag from ProvidersController * Remove flag from CreateMsp.cshtml * Remove flag from Provider Edit.cshtml Also ensured the editable Gateway fields show for Multi-organization enterprises * Remove flag from OrganizationsController * Remove flag from billing-owned provider controllers * Remove flag from OrganizationService * Remove flag from RemoveOrganizationFromProviderCommand * Remove flag from ProviderService * Remove flag * Run dotnet format * Fix failing tests
70 lines
2.3 KiB
C#
70 lines
2.3 KiB
C#
using Bit.Core.AdminConsole.Entities.Provider;
|
|
using Bit.Core.AdminConsole.Repositories;
|
|
using Bit.Core.Billing.Extensions;
|
|
using Bit.Core.Context;
|
|
using Bit.Core.Services;
|
|
|
|
namespace Bit.Api.Billing.Controllers;
|
|
|
|
public abstract class BaseProviderController(
|
|
ICurrentContext currentContext,
|
|
ILogger<BaseProviderController> logger,
|
|
IProviderRepository providerRepository,
|
|
IUserService userService) : BaseBillingController
|
|
{
|
|
protected readonly IUserService UserService = userService;
|
|
|
|
protected Task<(Provider, IResult)> TryGetBillableProviderForAdminOperation(
|
|
Guid providerId) => TryGetBillableProviderAsync(providerId, currentContext.ProviderProviderAdmin);
|
|
|
|
protected Task<(Provider, IResult)> TryGetBillableProviderForServiceUserOperation(
|
|
Guid providerId) => TryGetBillableProviderAsync(providerId, currentContext.ProviderUser);
|
|
|
|
private async Task<(Provider, IResult)> TryGetBillableProviderAsync(
|
|
Guid providerId,
|
|
Func<Guid, bool> checkAuthorization)
|
|
{
|
|
var provider = await providerRepository.GetByIdAsync(providerId);
|
|
|
|
if (provider == null)
|
|
{
|
|
logger.LogError(
|
|
"Cannot find provider ({ProviderID}) for Consolidated Billing operation",
|
|
providerId);
|
|
|
|
return (null, Error.NotFound());
|
|
}
|
|
|
|
if (!checkAuthorization(providerId))
|
|
{
|
|
var user = await UserService.GetUserByPrincipalAsync(User);
|
|
|
|
logger.LogError(
|
|
"User ({UserID}) is not authorized to perform Consolidated Billing operation for provider ({ProviderID})",
|
|
user?.Id, providerId);
|
|
|
|
return (null, Error.Unauthorized());
|
|
}
|
|
|
|
if (!provider.IsBillable())
|
|
{
|
|
logger.LogError(
|
|
"Cannot run Consolidated Billing operation for provider ({ProviderID}) that is not billable",
|
|
providerId);
|
|
|
|
return (null, Error.Unauthorized());
|
|
}
|
|
|
|
if (provider.IsStripeEnabled())
|
|
{
|
|
return (provider, null);
|
|
}
|
|
|
|
logger.LogError(
|
|
"Cannot run Consolidated Billing operation for provider ({ProviderID}) that is missing Stripe configuration",
|
|
providerId);
|
|
|
|
return (null, Error.ServerError());
|
|
}
|
|
}
|