mirror of
https://github.com/bitwarden/server.git
synced 2025-07-09 03:43:51 -05:00
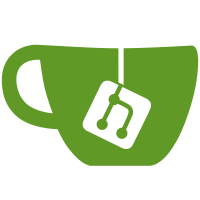
* Add Disable Send policy * Test DisableSend policy * PR Review * Update tests for using CurrentContext This required making an interface for CurrentContext and mocking out the members used. The interface can be expanded as needed for tests. I moved CurrentContext to a folder, which changes the namespace and causes a lot of file touches, but most are just adding a reference * Fix failing test * Update exemption to include all exempt users * Move all CurrentContext usages to ICurrentContext * PR review. Match messaging with Web
69 lines
2.1 KiB
C#
69 lines
2.1 KiB
C#
using System;
|
|
using System.ComponentModel.DataAnnotations;
|
|
using Bit.Core.Enums;
|
|
using Bit.Core.Models.Table;
|
|
|
|
namespace Bit.Portal.Models
|
|
{
|
|
public class PolicyModel
|
|
{
|
|
public PolicyModel() { }
|
|
|
|
public PolicyModel(Policy policy)
|
|
: this(policy.Type, policy.Enabled)
|
|
{ }
|
|
|
|
public PolicyModel(PolicyType policyType, bool enabled)
|
|
{
|
|
switch (policyType)
|
|
{
|
|
case PolicyType.TwoFactorAuthentication:
|
|
NameKey = "TwoStepLogin";
|
|
DescriptionKey = "TwoStepLoginDescription";
|
|
break;
|
|
|
|
case PolicyType.MasterPassword:
|
|
NameKey = "MasterPassword";
|
|
DescriptionKey = "MasterPasswordDescription";
|
|
break;
|
|
|
|
case PolicyType.PasswordGenerator:
|
|
NameKey = "PasswordGenerator";
|
|
DescriptionKey = "PasswordGeneratorDescription";
|
|
break;
|
|
|
|
case PolicyType.SingleOrg:
|
|
NameKey = "SingleOrganization";
|
|
DescriptionKey = "SingleOrganizationDescription";
|
|
break;
|
|
|
|
case PolicyType.RequireSso:
|
|
NameKey = "RequireSso";
|
|
DescriptionKey = "RequireSsoDescription";
|
|
break;
|
|
|
|
case PolicyType.PersonalOwnership:
|
|
NameKey = "PersonalOwnership";
|
|
DescriptionKey = "PersonalOwnershipDescription";
|
|
break;
|
|
|
|
case PolicyType.DisableSend:
|
|
NameKey = "DisableSend";
|
|
DescriptionKey = "DisableSendDescription";
|
|
break;
|
|
|
|
default:
|
|
throw new ArgumentOutOfRangeException();
|
|
}
|
|
|
|
PolicyType = policyType;
|
|
Enabled = enabled;
|
|
}
|
|
|
|
public string NameKey { get; set; }
|
|
public string DescriptionKey { get; set; }
|
|
public PolicyType PolicyType { get; set; }
|
|
public bool Enabled { get; set; }
|
|
}
|
|
}
|