mirror of
https://github.com/bitwarden/server.git
synced 2025-04-09 23:28:12 -05:00
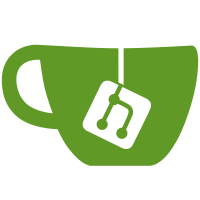
* Add Disable Send policy * Test DisableSend policy * PR Review * Update tests for using CurrentContext This required making an interface for CurrentContext and mocking out the members used. The interface can be expanded as needed for tests. I moved CurrentContext to a folder, which changes the namespace and causes a lot of file touches, but most are just adding a reference * Fix failing test * Update exemption to include all exempt users * Move all CurrentContext usages to ICurrentContext * PR review. Match messaging with Web
204 lines
6.4 KiB
C#
204 lines
6.4 KiB
C#
using System;
|
|
using System.Threading.Tasks;
|
|
using Bit.Core.Context;
|
|
using Bit.Core.Models.Table;
|
|
using Bit.Core.Enums;
|
|
using Newtonsoft.Json;
|
|
using Bit.Core.Models;
|
|
using Azure.Storage.Queues;
|
|
using Microsoft.AspNetCore.Http;
|
|
using System.Collections.Generic;
|
|
|
|
namespace Bit.Core.Services
|
|
{
|
|
public class AzureQueuePushNotificationService : IPushNotificationService
|
|
{
|
|
private readonly QueueClient _queueClient;
|
|
private readonly GlobalSettings _globalSettings;
|
|
private readonly IHttpContextAccessor _httpContextAccessor;
|
|
|
|
private JsonSerializerSettings _jsonSettings = new JsonSerializerSettings
|
|
{
|
|
NullValueHandling = NullValueHandling.Ignore
|
|
};
|
|
|
|
public AzureQueuePushNotificationService(
|
|
GlobalSettings globalSettings,
|
|
IHttpContextAccessor httpContextAccessor)
|
|
{
|
|
_queueClient = new QueueClient(globalSettings.Notifications.ConnectionString, "notifications");
|
|
_globalSettings = globalSettings;
|
|
_httpContextAccessor = httpContextAccessor;
|
|
}
|
|
|
|
public async Task PushSyncCipherCreateAsync(Cipher cipher, IEnumerable<Guid> collectionIds)
|
|
{
|
|
await PushCipherAsync(cipher, PushType.SyncCipherCreate, collectionIds);
|
|
}
|
|
|
|
public async Task PushSyncCipherUpdateAsync(Cipher cipher, IEnumerable<Guid> collectionIds)
|
|
{
|
|
await PushCipherAsync(cipher, PushType.SyncCipherUpdate, collectionIds);
|
|
}
|
|
|
|
public async Task PushSyncCipherDeleteAsync(Cipher cipher)
|
|
{
|
|
await PushCipherAsync(cipher, PushType.SyncLoginDelete, null);
|
|
}
|
|
|
|
private async Task PushCipherAsync(Cipher cipher, PushType type, IEnumerable<Guid> collectionIds)
|
|
{
|
|
if (cipher.OrganizationId.HasValue)
|
|
{
|
|
var message = new SyncCipherPushNotification
|
|
{
|
|
Id = cipher.Id,
|
|
OrganizationId = cipher.OrganizationId,
|
|
RevisionDate = cipher.RevisionDate,
|
|
CollectionIds = collectionIds,
|
|
};
|
|
|
|
await SendMessageAsync(type, message, true);
|
|
}
|
|
else if (cipher.UserId.HasValue)
|
|
{
|
|
var message = new SyncCipherPushNotification
|
|
{
|
|
Id = cipher.Id,
|
|
UserId = cipher.UserId,
|
|
RevisionDate = cipher.RevisionDate,
|
|
};
|
|
|
|
await SendMessageAsync(type, message, true);
|
|
}
|
|
}
|
|
|
|
public async Task PushSyncFolderCreateAsync(Folder folder)
|
|
{
|
|
await PushFolderAsync(folder, PushType.SyncFolderCreate);
|
|
}
|
|
|
|
public async Task PushSyncFolderUpdateAsync(Folder folder)
|
|
{
|
|
await PushFolderAsync(folder, PushType.SyncFolderUpdate);
|
|
}
|
|
|
|
public async Task PushSyncFolderDeleteAsync(Folder folder)
|
|
{
|
|
await PushFolderAsync(folder, PushType.SyncFolderDelete);
|
|
}
|
|
|
|
private async Task PushFolderAsync(Folder folder, PushType type)
|
|
{
|
|
var message = new SyncFolderPushNotification
|
|
{
|
|
Id = folder.Id,
|
|
UserId = folder.UserId,
|
|
RevisionDate = folder.RevisionDate
|
|
};
|
|
|
|
await SendMessageAsync(type, message, true);
|
|
}
|
|
|
|
public async Task PushSyncCiphersAsync(Guid userId)
|
|
{
|
|
await PushUserAsync(userId, PushType.SyncCiphers);
|
|
}
|
|
|
|
public async Task PushSyncVaultAsync(Guid userId)
|
|
{
|
|
await PushUserAsync(userId, PushType.SyncVault);
|
|
}
|
|
|
|
public async Task PushSyncOrgKeysAsync(Guid userId)
|
|
{
|
|
await PushUserAsync(userId, PushType.SyncOrgKeys);
|
|
}
|
|
|
|
public async Task PushSyncSettingsAsync(Guid userId)
|
|
{
|
|
await PushUserAsync(userId, PushType.SyncSettings);
|
|
}
|
|
|
|
public async Task PushLogOutAsync(Guid userId)
|
|
{
|
|
await PushUserAsync(userId, PushType.LogOut);
|
|
}
|
|
|
|
private async Task PushUserAsync(Guid userId, PushType type)
|
|
{
|
|
var message = new UserPushNotification
|
|
{
|
|
UserId = userId,
|
|
Date = DateTime.UtcNow
|
|
};
|
|
|
|
await SendMessageAsync(type, message, false);
|
|
}
|
|
|
|
public async Task PushSyncSendCreateAsync(Send send)
|
|
{
|
|
await PushSendAsync(send, PushType.SyncSendCreate);
|
|
}
|
|
|
|
public async Task PushSyncSendUpdateAsync(Send send)
|
|
{
|
|
await PushSendAsync(send, PushType.SyncSendUpdate);
|
|
}
|
|
|
|
public async Task PushSyncSendDeleteAsync(Send send)
|
|
{
|
|
await PushSendAsync(send, PushType.SyncSendDelete);
|
|
}
|
|
|
|
private async Task PushSendAsync(Send send, PushType type)
|
|
{
|
|
if (send.UserId.HasValue)
|
|
{
|
|
var message = new SyncSendPushNotification
|
|
{
|
|
Id = send.Id,
|
|
UserId = send.UserId.Value,
|
|
RevisionDate = send.RevisionDate
|
|
};
|
|
|
|
await SendMessageAsync(type, message, true);
|
|
}
|
|
}
|
|
|
|
private async Task SendMessageAsync<T>(PushType type, T payload, bool excludeCurrentContext)
|
|
{
|
|
var contextId = GetContextIdentifier(excludeCurrentContext);
|
|
var message = JsonConvert.SerializeObject(new PushNotificationData<T>(type, payload, contextId),
|
|
_jsonSettings);
|
|
await _queueClient.SendMessageAsync(message);
|
|
}
|
|
|
|
private string GetContextIdentifier(bool excludeCurrentContext)
|
|
{
|
|
if (!excludeCurrentContext)
|
|
{
|
|
return null;
|
|
}
|
|
|
|
var currentContext = _httpContextAccessor?.HttpContext?.
|
|
RequestServices.GetService(typeof(ICurrentContext)) as ICurrentContext;
|
|
return currentContext?.DeviceIdentifier;
|
|
}
|
|
|
|
public Task SendPayloadToUserAsync(string userId, PushType type, object payload, string identifier,
|
|
string deviceId = null)
|
|
{
|
|
// Noop
|
|
return Task.FromResult(0);
|
|
}
|
|
|
|
public Task SendPayloadToOrganizationAsync(string orgId, PushType type, object payload, string identifier,
|
|
string deviceId = null)
|
|
{
|
|
// Noop
|
|
return Task.FromResult(0);
|
|
}
|
|
}
|
|
}
|