mirror of
https://github.com/bitwarden/server.git
synced 2025-07-14 22:27:32 -05:00
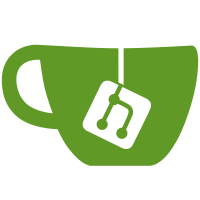
* Implement userkey rotation v2 * Update request models * Cleanup * Update tests * Improve test * Add tests * Fix formatting * Fix test * Remove whitespace * Fix namespace * Enable nullable on models * Fix build * Add tests and enable nullable on masterpasswordunlockdatamodel * Fix test * Remove rollback * Add tests * Make masterpassword hint optional * Update user query * Add EF test * Improve test * Cleanup * Set masterpassword hint * Remove connection close * Add tests for invalid kdf types * Update test/Core.Test/KeyManagement/UserKey/RotateUserAccountKeysCommandTests.cs Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com> * Fix formatting * Update src/Api/KeyManagement/Models/Requests/RotateAccountKeysAndDataRequestModel.cs Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com> * Update src/Api/Auth/Models/Request/Accounts/MasterPasswordUnlockDataModel.cs Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com> * Update src/Api/Auth/Models/Request/Accounts/MasterPasswordUnlockDataModel.cs Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com> * Update src/Api/KeyManagement/Models/Requests/AccountKeysRequestModel.cs Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com> * Fix imports * Fix tests * Remove null check * Add rollback --------- Co-authored-by: Thomas Avery <43214426+Thomas-Avery@users.noreply.github.com>
69 lines
2.5 KiB
C#
69 lines
2.5 KiB
C#
#nullable enable
|
|
using System.ComponentModel.DataAnnotations;
|
|
using Bit.Api.Auth.Models.Request.Accounts;
|
|
using Bit.Core.Enums;
|
|
using Xunit;
|
|
|
|
namespace Bit.Api.Test.KeyManagement.Models.Request;
|
|
|
|
public class MasterPasswordUnlockDataModelTests
|
|
{
|
|
|
|
readonly string _mockEncryptedString = "2.3Uk+WNBIoU5xzmVFNcoWzz==|1MsPIYuRfdOHfu/0uY6H2Q==|/98sp4wb6pHP1VTZ9JcNCYgQjEUMFPlqJgCwRk1YXKg=";
|
|
|
|
[Theory]
|
|
[InlineData(KdfType.PBKDF2_SHA256, 5000, null, null)]
|
|
[InlineData(KdfType.PBKDF2_SHA256, 100000, null, null)]
|
|
[InlineData(KdfType.PBKDF2_SHA256, 600000, null, null)]
|
|
[InlineData(KdfType.Argon2id, 3, 64, 4)]
|
|
public void Validate_Success(KdfType kdfType, int kdfIterations, int? kdfMemory, int? kdfParallelism)
|
|
{
|
|
var model = new MasterPasswordUnlockDataModel
|
|
{
|
|
KdfType = kdfType,
|
|
KdfIterations = kdfIterations,
|
|
KdfMemory = kdfMemory,
|
|
KdfParallelism = kdfParallelism,
|
|
Email = "example@example.com",
|
|
MasterKeyAuthenticationHash = "hash",
|
|
MasterKeyEncryptedUserKey = _mockEncryptedString,
|
|
MasterPasswordHint = "hint"
|
|
};
|
|
var result = Validate(model);
|
|
Assert.Empty(result);
|
|
}
|
|
|
|
[Theory]
|
|
[InlineData(KdfType.Argon2id, 1, null, 1)]
|
|
[InlineData(KdfType.Argon2id, 1, 64, null)]
|
|
[InlineData(KdfType.PBKDF2_SHA256, 5000, 0, null)]
|
|
[InlineData(KdfType.PBKDF2_SHA256, 5000, null, 0)]
|
|
[InlineData(KdfType.PBKDF2_SHA256, 5000, 0, 0)]
|
|
[InlineData((KdfType)2, 100000, null, null)]
|
|
[InlineData((KdfType)2, 2, 64, 4)]
|
|
public void Validate_Failure(KdfType kdfType, int kdfIterations, int? kdfMemory, int? kdfParallelism)
|
|
{
|
|
var model = new MasterPasswordUnlockDataModel
|
|
{
|
|
KdfType = kdfType,
|
|
KdfIterations = kdfIterations,
|
|
KdfMemory = kdfMemory,
|
|
KdfParallelism = kdfParallelism,
|
|
Email = "example@example.com",
|
|
MasterKeyAuthenticationHash = "hash",
|
|
MasterKeyEncryptedUserKey = _mockEncryptedString,
|
|
MasterPasswordHint = "hint"
|
|
};
|
|
var result = Validate(model);
|
|
Assert.Single(result);
|
|
Assert.NotNull(result.First().ErrorMessage);
|
|
}
|
|
|
|
private static List<ValidationResult> Validate(MasterPasswordUnlockDataModel model)
|
|
{
|
|
var results = new List<ValidationResult>();
|
|
Validator.TryValidateObject(model, new ValidationContext(model), results, true);
|
|
return results;
|
|
}
|
|
}
|