mirror of
https://github.com/bitwarden/server.git
synced 2025-07-09 03:43:51 -05:00
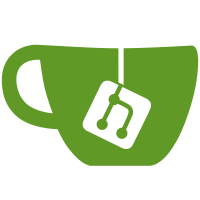
* Moved AccountsBilling controller to be owned by Billing * Added org billing history endpoint * Updated GetBillingInvoicesAsync to only retrieve paid, open, and uncollectible invoices, and added option to limit results * Removed invoices and transactions from GetBillingAsync * Limiting the number of invoices and transactions returned * Moved Billing models to Billing namespace * Split billing info and billing history objects * Removed billing method GetBillingBalanceAndSourceAsync * Removed unused using * Cleaned up BillingInfo a bit * Update migration scripts to use `CREATE OR ALTER` instead of checking for the `OBJECT_ID` * Applying limit to aggregated invoices after they return from Stripe
73 lines
2.1 KiB
C#
73 lines
2.1 KiB
C#
using Bit.Api.Billing.Models.Responses;
|
|
using Bit.Core.Billing.Services;
|
|
using Bit.Core.Context;
|
|
using Bit.Core.Repositories;
|
|
using Bit.Core.Services;
|
|
using Bit.Core.Utilities;
|
|
using Microsoft.AspNetCore.Authorization;
|
|
using Microsoft.AspNetCore.Mvc;
|
|
|
|
namespace Bit.Api.Billing.Controllers;
|
|
|
|
[Route("organizations/{organizationId:guid}/billing")]
|
|
[Authorize("Application")]
|
|
public class OrganizationBillingController(
|
|
ICurrentContext currentContext,
|
|
IOrganizationBillingService organizationBillingService,
|
|
IOrganizationRepository organizationRepository,
|
|
IPaymentService paymentService) : Controller
|
|
{
|
|
[HttpGet("metadata")]
|
|
public async Task<IResult> GetMetadataAsync([FromRoute] Guid organizationId)
|
|
{
|
|
var metadata = await organizationBillingService.GetMetadata(organizationId);
|
|
|
|
if (metadata == null)
|
|
{
|
|
return TypedResults.NotFound();
|
|
}
|
|
|
|
var response = OrganizationMetadataResponse.From(metadata);
|
|
|
|
return TypedResults.Ok(response);
|
|
}
|
|
|
|
[HttpGet("history")]
|
|
public async Task<IResult> GetHistoryAsync([FromRoute] Guid organizationId)
|
|
{
|
|
var organization = await organizationRepository.GetByIdAsync(organizationId);
|
|
|
|
if (organization == null)
|
|
{
|
|
return TypedResults.NotFound();
|
|
}
|
|
|
|
var billingInfo = await paymentService.GetBillingHistoryAsync(organization);
|
|
|
|
return TypedResults.Ok(billingInfo);
|
|
}
|
|
|
|
[HttpGet]
|
|
[SelfHosted(NotSelfHostedOnly = true)]
|
|
public async Task<IResult> GetBillingAsync(Guid organizationId)
|
|
{
|
|
if (!await currentContext.ViewBillingHistory(organizationId))
|
|
{
|
|
return TypedResults.Unauthorized();
|
|
}
|
|
|
|
var organization = await organizationRepository.GetByIdAsync(organizationId);
|
|
|
|
if (organization == null)
|
|
{
|
|
return TypedResults.NotFound();
|
|
}
|
|
|
|
var billingInfo = await paymentService.GetBillingAsync(organization);
|
|
|
|
var response = new BillingResponseModel(billingInfo);
|
|
|
|
return TypedResults.Ok(response);
|
|
}
|
|
}
|