mirror of
https://github.com/mtrojnar/osslsigncode.git
synced 2025-07-02 19:22:47 -05:00
Set compiler and linker flags
This commit is contained in:
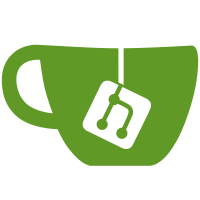
committed by
Michał Trojnara
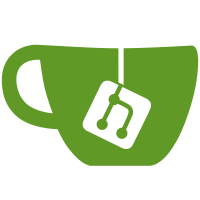
parent
6b3450ada8
commit
7b29b45348
92
cmake/SetCompilerFlags.cmake
Normal file
92
cmake/SetCompilerFlags.cmake
Normal file
@ -0,0 +1,92 @@
|
|||||||
|
include(CheckCCompilerFlag)
|
||||||
|
|
||||||
|
function(add_compile_flags_target target)
|
||||||
|
if (CMAKE_C_COMPILER_ID MATCHES "Clang|AppleClang|GNU" )
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-ggdb -g>)
|
||||||
|
endif()
|
||||||
|
|
||||||
|
if(CMAKE_C_COMPILER_ID MATCHES "GNU|Clang")
|
||||||
|
# Support address space layout randomization (ASLR)
|
||||||
|
target_compile_options(${target} PRIVATE -fPIE)
|
||||||
|
check_c_compiler_flag("-fstack-protector-all" HAVE_STACK_PROTECTOR_ALL)
|
||||||
|
if(HAVE_STACK_PROTECTOR_ALL)
|
||||||
|
target_link_options(${target} PRIVATE -fstack-protector-all)
|
||||||
|
else()
|
||||||
|
check_c_compiler_flag("-fstack-protector" HAVE_STACK_PROTECTOR)
|
||||||
|
if(HAVE_STACK_PROTECTOR)
|
||||||
|
target_link_options(${target} PRIVATE -fstack-protector)
|
||||||
|
else()
|
||||||
|
message(WARNING "No stack protection supported")
|
||||||
|
endif()
|
||||||
|
endif()
|
||||||
|
target_link_options(${target} PRIVATE -fstack-check)
|
||||||
|
target_link_options(${target} PRIVATE -fPIE -pie)
|
||||||
|
target_link_options(${target} PRIVATE -Wl,-z,relro)
|
||||||
|
target_link_options(${target} PRIVATE -Wl,-z,now)
|
||||||
|
target_link_options(${target} PRIVATE -Wl,-z,noexecstack)
|
||||||
|
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-O2>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-pedantic>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-Wno-long-long>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-Wconversion>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-D_FORTIFY_SOURCE=2>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-Wformat=2>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-Wundef>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-Wshadow>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-Wredundant-decls>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-Wcast-qual>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-Wnull-dereference>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-Wmissing-declarations>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-Wmissing-prototypes>)
|
||||||
|
endif()
|
||||||
|
|
||||||
|
if(CMAKE_C_COMPILER_ID MATCHES "GNU")
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-Wall>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-Wextra>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-Wno-deprecated-declarations>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-Wstrict-aliasing=3>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-Wstrict-overflow=2>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-Wlogical-op>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-Wwrite-strings>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-Wcast-align=strict>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-Wdisabled-optimization>)
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:-Wshift-overflow=2>)
|
||||||
|
endif()
|
||||||
|
|
||||||
|
if(MSVC)
|
||||||
|
# Enable parallel builds
|
||||||
|
add_definitions(/MP)
|
||||||
|
# Use address space layout randomization, generate PIE code for ASLR (default on)
|
||||||
|
target_link_options(${target} PRIVATE /DYNAMICBASE)
|
||||||
|
# Create terminal server aware application (default on)
|
||||||
|
target_link_options(${target} PRIVATE /TSAWARE)
|
||||||
|
# Mark the binary as compatible with Intel Control-flow Enforcement Technology (CET) Shadow Stack
|
||||||
|
target_link_options(${target} PRIVATE /CETCOMPAT)
|
||||||
|
# Enable compiler generation of Control Flow Guard security checks
|
||||||
|
target_compile_options(${target} PRIVATE /guard:cf)
|
||||||
|
target_link_options(${target} PRIVATE /guard:cf)
|
||||||
|
# Buffer Security Check
|
||||||
|
target_compile_options(${target} PRIVATE /GS)
|
||||||
|
# Suppress startup banner
|
||||||
|
target_link_options(${target} PRIVATE /NOLOGO)
|
||||||
|
# Generate debug info
|
||||||
|
target_link_options(${target} PRIVATE /DEBUG)
|
||||||
|
if("${CMAKE_SIZEOF_VOID_P}" STREQUAL "8")
|
||||||
|
# High entropy ASLR for 64 bits targets (default on)
|
||||||
|
target_link_options(${target} PRIVATE /HIGHENTROPYVA)
|
||||||
|
# Enable generation of EH Continuation (EHCONT) metadata by the compiler
|
||||||
|
target_compile_options(${target} PRIVATE /guard:ehcont)
|
||||||
|
target_link_options(${target} PRIVATE /guard:ehcont)
|
||||||
|
else()
|
||||||
|
# Can handle addresses larger than 2 gigabytes
|
||||||
|
target_link_options(${target} PRIVATE /LARGEADDRESSAWARE)
|
||||||
|
# Safe structured exception handlers (x86 only)
|
||||||
|
target_link_options(${target} PRIVATE /SAFESEH)
|
||||||
|
endif()
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:/D_FORTIFY_SOURCE=2>)
|
||||||
|
# Unrecognized compiler options are errors
|
||||||
|
target_compile_options(${target} PRIVATE $<$<CONFIG:DEBUG>:/options:strict>)
|
||||||
|
endif()
|
||||||
|
endfunction()
|
||||||
|
|
||||||
|
add_compile_flags_target(osslsigncode)
|
@ -1,30 +0,0 @@
|
|||||||
# add command line options
|
|
||||||
|
|
||||||
# set Release build mode
|
|
||||||
if(NOT CMAKE_BUILD_TYPE)
|
|
||||||
set(CMAKE_BUILD_TYPE "Release" CACHE STRING "Choose Release or Debug" FORCE)
|
|
||||||
endif()
|
|
||||||
|
|
||||||
option(enable-strict "Enable strict compile mode" OFF)
|
|
||||||
option(enable-pedantic "Enable pedantic compile mode" OFF)
|
|
||||||
option(with-curl "Enable curl" ON)
|
|
||||||
|
|
||||||
# enable compile options
|
|
||||||
if(enable-strict)
|
|
||||||
message(STATUS "Enable strict compile mode")
|
|
||||||
if(MSVC)
|
|
||||||
# Microsoft Visual C warning level
|
|
||||||
add_compile_options(/Wall)
|
|
||||||
else()
|
|
||||||
add_compile_options(-Wall -Wextra)
|
|
||||||
endif()
|
|
||||||
endif()
|
|
||||||
|
|
||||||
if(enable-pedantic)
|
|
||||||
message(STATUS "Enable pedantic compile mode")
|
|
||||||
if(MSVC)
|
|
||||||
add_compile_options(/W4)
|
|
||||||
else()
|
|
||||||
add_compile_options(-pedantic)
|
|
||||||
endif()
|
|
||||||
endif()
|
|
Reference in New Issue
Block a user